NIO technology implemented in Java functions allows applications to efficiently handle I/O operations without blocking other threads. It leverages: Non-blocking channels (NioSocketChannel and NioServerSocketChannel) Selector to monitor the status of the channel Server-side listens for incoming connections and creates new channels Clients connect to the server and send requests Advantages include high responsiveness, thread isolation, and scalability
NIO technology implemented in Java functions
Overview
NIO (non Blocking I/O technology is an asynchronous I/O technology that allows applications to interact with networks and files without blocking other threads. Implementing NIO in Java functions can improve your application's I/O performance and responsiveness.
Practical case
Using NioServerSocketChannel and NioSocketChannel
NIO server-side useNioServerSocketChannel
Listening incoming connections and use NioSocketChannel
to create a new channel for each connection. The client uses NioSocketChannel
to connect to the server.
// 服务器端 ServerSocketChannel serverSocketChannel = ServerSocketChannel.open(); serverSocketChannel.bind(new InetSocketAddress(PORT)); while (true) { SocketChannel socketChannel = serverSocketChannel.accept(); ... // 处理请求 } // 客户端 SocketChannel socketChannel = SocketChannel.open(); socketChannel.connect(new InetSocketAddress(HOST, PORT)); ... // 发送请求
Using Selector
Selector is used to monitor the status of multiple channels. The selector notifies the application when one or more channels are readable, writable, or connected.
// 初始化 selector Selector selector = Selector.open(); // 注册服务器端 channel 到 selector serverSocketChannel.register(selector, SelectionKey.OP_ACCEPT); // 循环监听事件 while (true) { // 阻塞直到一个或多个通道就绪 int readyChannels = selector.select(); if (readyChannels > 0) { Set<SelectionKey> selectedKeys = selector.selectedKeys(); for (SelectionKey key : selectedKeys) { if (key.isAcceptable()) { // 处理传入连接 } else if (key.isReadable()) { // 处理可读数据 } else if (key.isWritable()) { // 处理可写数据 } } selectedKeys.clear(); } }
Advantages
- Non-blocking operation, improve responsiveness
- Thread isolation, minimize context switching
- High scalability, supporting a large number of concurrent connections
Limitations
- Increased complexity, requiring more advanced programming skills
- Implement pseudo-asynchronous mode, which may still block the current thread
The above is the detailed content of How is NIO technology implemented in Java functions?. For more information, please follow other related articles on the PHP Chinese website!
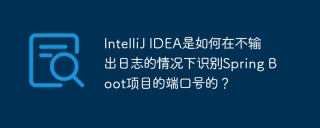
Start Spring using IntelliJIDEAUltimate version...
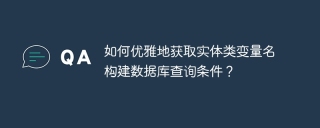
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
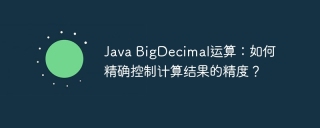
Java...
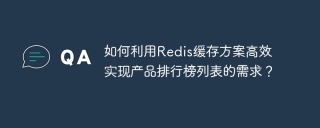
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
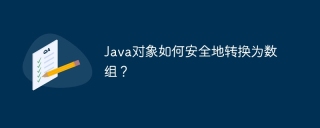
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
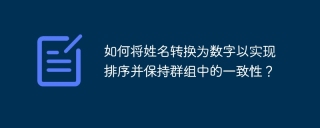
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
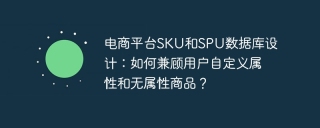
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
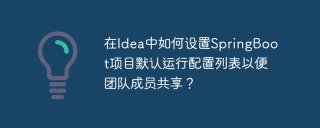
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
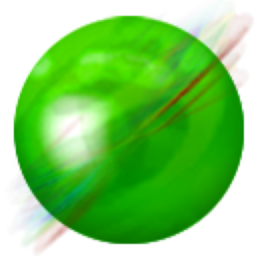
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
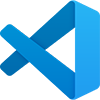
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment