Anonymous inner class is a special inner class used to implement the callback mechanism and provide customized behavior for other code. They achieve their functions by overriding non-abstract methods of external classes, including event handling, callback mechanisms and dynamic behavior. In practice, anonymous inner classes can be used to implement button click event handlers and callback functions.
The role of anonymous inner classes in Java
Anonymous inner classes is a special inner class in Java that has no clear class name, but directly implemented as an inner class of its outer class. Anonymous inner classes are mainly used to implement callback mechanisms and provide customized behavior for other code.
Create anonymous inner classes
Anonymous inner classes can be created through the following syntax:
new OuterClass() { // 匿名内部类的实现 }
For example:
// 创建一个按钮,当按下时打印"Hello World" JButton button = new JButton() { @Override public void actionPerformed(ActionEvent e) { System.out.println("Hello World!"); } };
Function
Anonymous inner classes Classes can achieve the following functions by overriding non-abstract methods of external classes:
- Event processing: Anonymous inner classes are often used for event processing, such as mouse click events and keyboard input events. wait.
- Callback mechanism: Anonymous inner classes can provide callback functions for other codes to achieve customized processing.
- Dynamic behavior: Anonymous inner classes can dynamically modify the behavior of outer classes according to specific scenarios.
Practical Case
Case 1: Event Handling
Use an anonymous inner class to implement a button click event handler:
// 创建一个按钮 JButton button = new JButton("Click Me"); // 为按钮添加点击事件处理器 button.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { System.out.println("Button clicked!"); } });
Case 2: Callback mechanism
Define an interface to define a callback method that needs to be implemented:
interface MyCallback { void callback(String message); }
Use an anonymous inner class to implement the callback method:
MyCallback callback = new MyCallback() { @Override public void callback(String message) { System.out.println("Callback received: " + message); } };
Then you can call the callback
method where needed:
callback.callback("Hello from callback!");
The above is the detailed content of What is the purpose of anonymous inner classes in Java?. For more information, please follow other related articles on the PHP Chinese website!
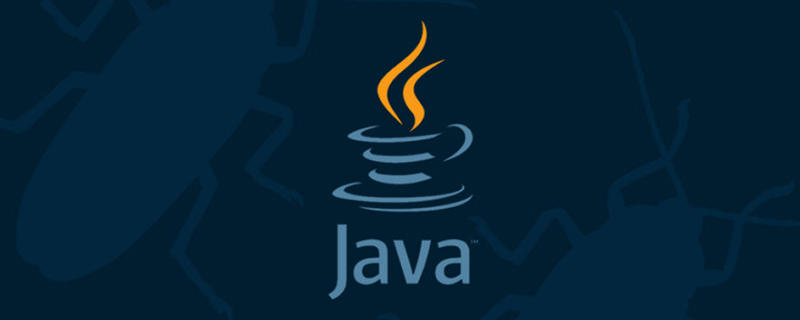
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
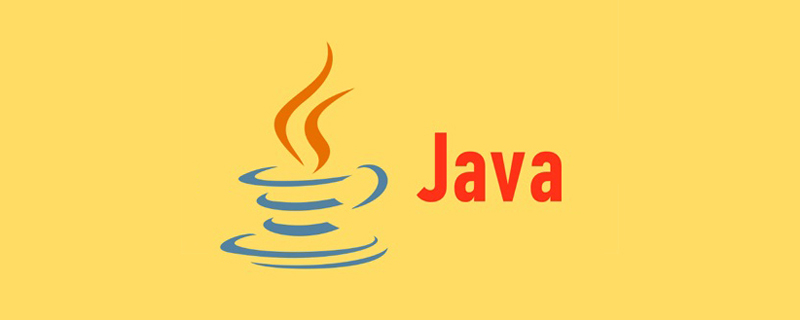
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
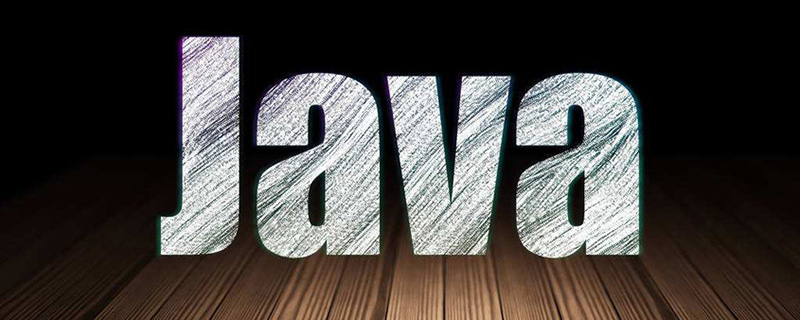
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
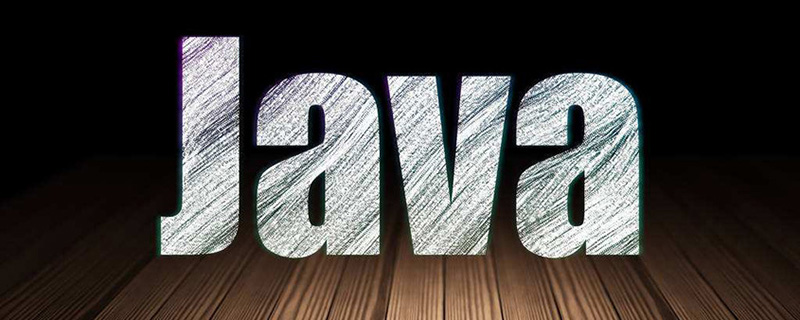
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
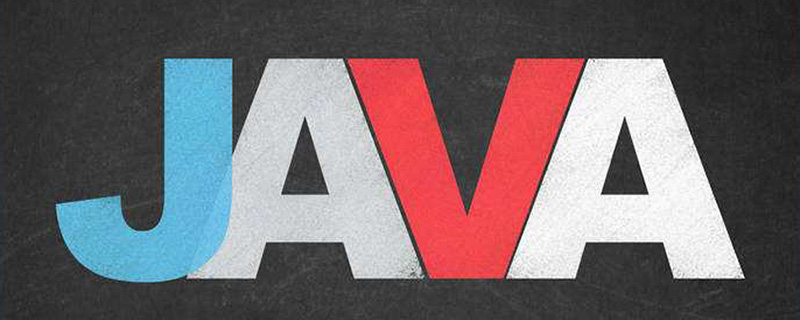
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
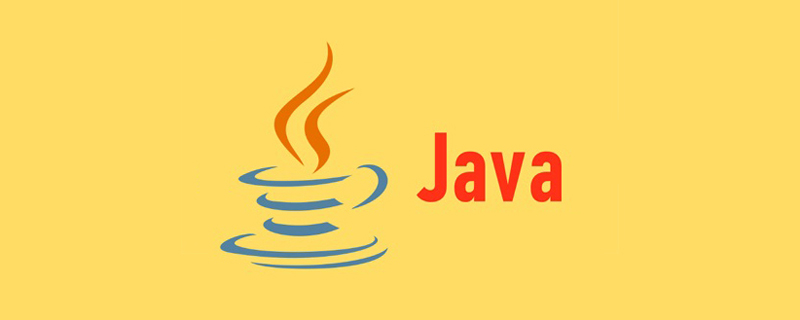
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
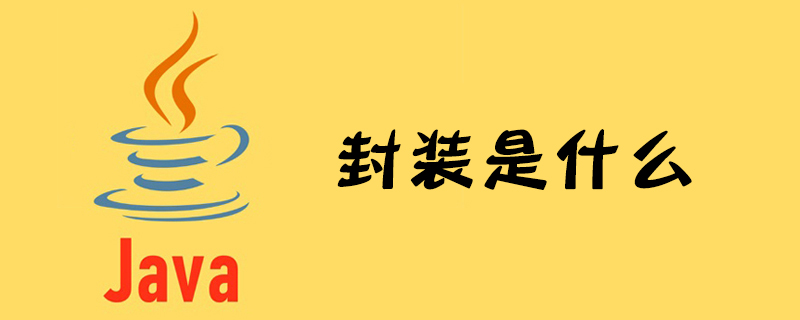
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
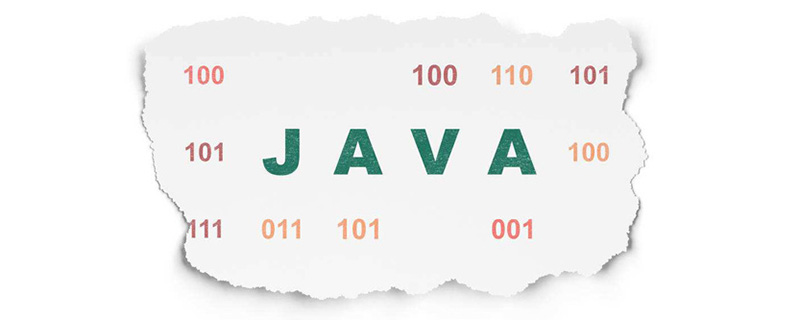
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
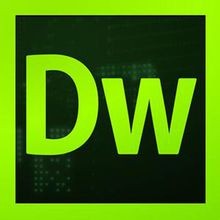
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
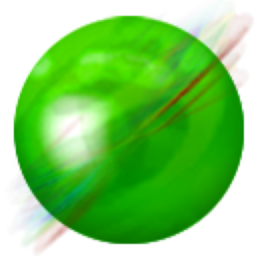
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
