


Quickly calculate array intersection and union using bitwise operations in PHP
In PHP, array intersections and unions can be efficiently calculated through bitwise operators: Intersection: Using the bitwise AND operator (&), co-existing elements are intersections. Union: Using the bitwise OR operator (|), the union contains all elements.
Use bitwise operations in PHP to quickly calculate the intersection and union of arrays
The bitwise operators provide the implementation of arrays in PHP Efficient methods for intersection and union. These operators operate on numbers bit by bit, allowing us to compare array values on a binary bit level.
Intersection
The intersection contains elements that appear in both arrays. We can use the bitwise AND operator &
to calculate the intersection:
<?php $array1 = [1, 2, 3, 4, 5]; $array2 = [3, 4, 5, 6, 7]; $intersection = array_intersect_bitwise($array1, $array2); var_dump($intersection); // 输出: [3, 4, 5] ?>
Union
The union contains all the elements in both arrays . We can use the bitwise OR operator |
to calculate the union:
<?php $array1 = [1, 2, 3, 4, 5]; $array2 = [3, 4, 5, 6, 7]; $union = array_union_bitwise($array1, $array2); var_dump($union); // 输出: [1, 2, 3, 4, 5, 6, 7] ?>
Practical case: Calculate the pages visited by the user
Suppose you There is an array containing the pages visited by the user:
<?php $userPages = [ 'Home', 'About', 'Contact' ]; $adminPages = [ 'Dashboard', 'Users', 'Settings', 'About' ]; ?>
You can use bitwise operations to quickly find out the pages visited by both the user and the administrator:
<?php $intersection = array_intersect_bitwise($userPages, $adminPages); var_dump($intersection); // 输出: ['About'] ?>
The above is the detailed content of Quickly calculate array intersection and union using bitwise operations in PHP. For more information, please follow other related articles on the PHP Chinese website!
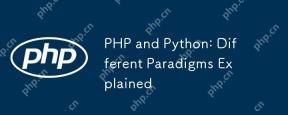
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
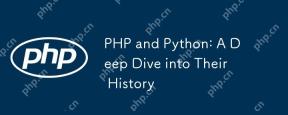
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
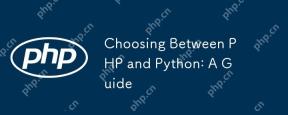
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
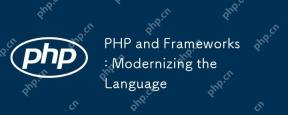
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
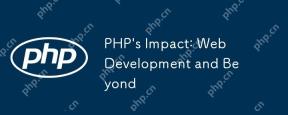
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
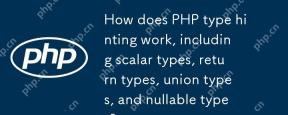
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
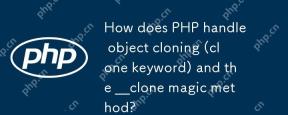
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
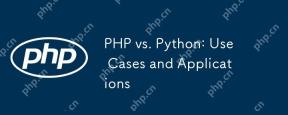
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
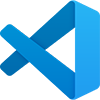
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
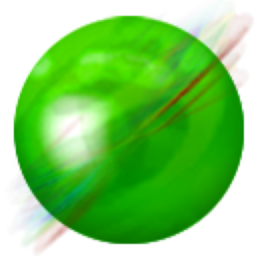
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
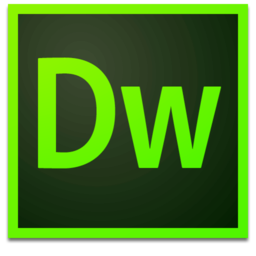
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.