


Application of recursion in C++ algorithms: efficiency improvement and complexity analysis
The application of recursion in C algorithm can improve efficiency. Taking the Fibonacci sequence calculation as an example, the function fibonacci calls itself recursively, with a complexity of O(2^n). However, for recursive problems such as tree structures, recursion can greatly improve efficiency because the size of each problem is halved. But be careful to avoid problems such as infinite recursion and insufficient stack space. For complex recursive problems, loop or iterative methods may be more effective.
Application of Recursion in C Algorithm: Efficiency Improvement and Complexity Analysis
Introduction
Recursion is a powerful programming technique that can be used to simplify algorithms and increase efficiency. In C, recursion is implemented by a function calling itself.
Code Example
Take the following Fibonacci sequence calculation as an example:
int fibonacci(int n) { if (n <= 1) { return n; } else { return fibonacci(n - 1) + fibonacci(n - 2); } }
How to run
- Function
fibonacci
accepts an integer parametern
, representing then
th number in the Fibonacci sequence to be calculated. - If
n
is less than or equal to 1, returnn
directly because this is the first or second item of the sequence. - Otherwise, the function calls itself recursively twice: once with
n - 1
, and once withn - 2
. - The recursive call continues until
n
decreases to 1 or 0. - The function returns the final calculated Fibonacci number.
Efficiency improvement
The efficiency of the recursive algorithm depends on the size of the problem type. For recursive problems such as tree structures, recursion can significantly improve efficiency because the size of each problem is reduced by half.
Complexity Analysis
The complexity of the Fibonacci sequence algorithm is O(2^n), because each recursive call will generate two new recursions transfer. For large values of n
, this results in an inefficient algorithm.
Practical case
- Folder traversal
- Graph search
- Divide and conquer algorithm (such as merge sort)
Notes
- When using recursion, it is important to avoid infinite recursion.
- Recursive algorithms may require a large amount of stack space, especially if the call depth is large.
- For complex recursive problems, it may be more efficient to use a loop or iterative approach (such as dynamic programming).
The above is the detailed content of Application of recursion in C++ algorithms: efficiency improvement and complexity analysis. For more information, please follow other related articles on the PHP Chinese website!
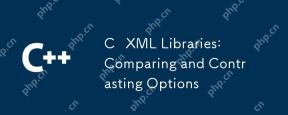
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
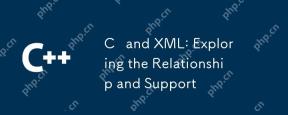
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
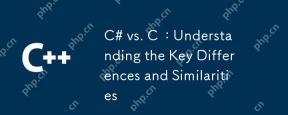
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
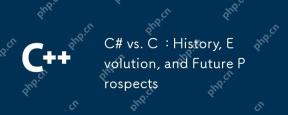
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
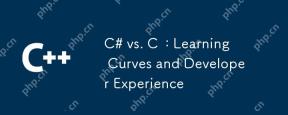
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
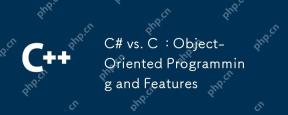
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
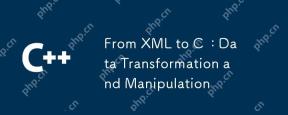
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
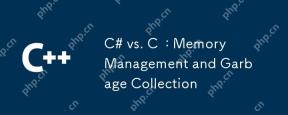
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.