


What is the difference between golang function overloading and polymorphism?
Function overloading is not supported in the Go language because it uses duck typing to determine the value type based on the actual type. Polymorphism is achieved through interface types and method calls, and objects of different categories can respond in the same way. Specifically, by defining interfaces and implementing these methods, Go language can make objects of different types have similar behaviors, thereby supporting polymorphism.
The difference between function overloading and polymorphism in Go language
Function overloading
Function overloading It refers to defining multiple functions with the same name but different parameter types in the same scope. Go language does not support function overloading. The reason is that the Go language uses duck typing, which determines the type of a value based on its actual type rather than its declared type. So if two functions have the same name but different parameter types, the Go compiler will still treat them as different functions.
Polymorphism
Polymorphism is a concept that enables objects of different categories to respond in the same way by calling methods of inheritance or interfaces. The Go language supports polymorphism through the use of interface types. An interface defines a set of methods, and any type can implement the interface as long as it implements these methods.
Practical case
Suppose we have the following Shape interface:
type Shape interface { Area() float64 }
We define two types that implement this interface:
Rectangle.go
type Rectangle struct { Length, Width float64 } func (r Rectangle) Area() float64 { return r.Length * r.Width }
Circle.go
type Circle struct { Radius float64 } func (c Circle) Area() float64 { return math.Pi * c.Radius * c.Radius }
We can then use a function to calculate the areas of different shapes without knowing them The specific type:
ShapeUtil.go
func CalculateArea(shape Shape) float64 { return shape.Area() }
main.go
func main() { rect := Rectangle{Length: 5.0, Width: 3.0} circle := Circle{Radius: 4.0} fmt.Println("Rectangle area:", CalculateArea(rect)) fmt.Println("Circle area:", CalculateArea(circle)) }
In the main function, regardless of the shape Regardless of the specific type, you can use the CalculateArea function to calculate their area.
The above is the detailed content of What is the difference between golang function overloading and polymorphism?. For more information, please follow other related articles on the PHP Chinese website!
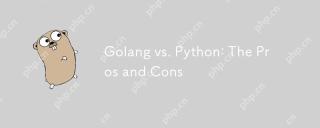
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
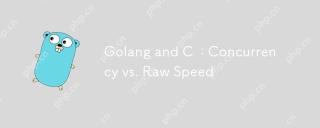
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
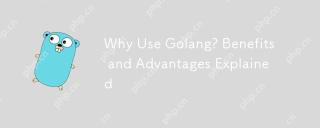
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
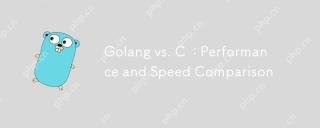
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
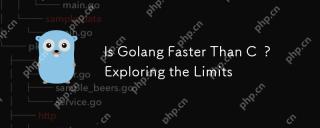
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
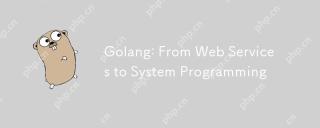
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
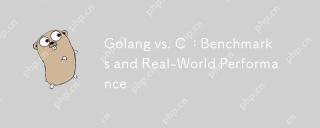
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
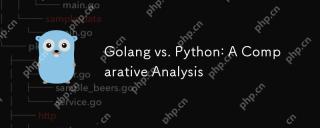
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
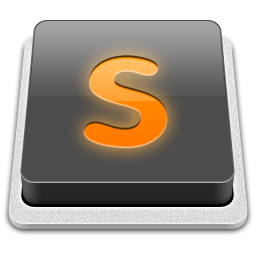
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
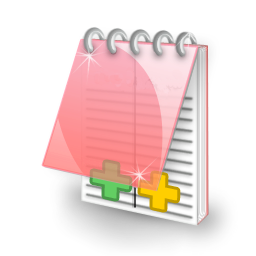
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.