


How to enhance the performance of Java functions through asynchronous programming?
Answer: Asynchronous programming is the key to improving the performance of Java functions, using dedicated threads or callbacks to perform long-term or I/O-intensive tasks concurrently. The benefits of asynchronous programming include: higher concurrency and improved responsiveness. Lower latency, less time waiting for I/O operations to complete. Better scalability to handle large volumes of operations without performance degradation.
Asynchronous programming: the key to improving Java function performance
Introduction
In In today's competitive digital age, optimizing code performance is crucial. Traditional synchronous programming methods can lead to bottlenecks and inefficiencies. This article will explore how to use asynchronous programming to improve the performance of Java functions and provide practical cases.
Asynchronous Programming
Asynchronous programming is a programming paradigm that allows functions to perform long-lasting or I/O-intensive tasks without blocking other operations. This is accomplished by delegating such tasks to dedicated threads or callbacks.
Asynchronous Programming in Java
Java provides a variety of APIs for asynchronous programming, including CompletableFuture, RxJava, Vert.x, etc. These APIs make it easy to create and manage asynchronous tasks.
Practical case: Concurrent file reading and writing
Consider a file processing function that needs to read and write multiple files at the same time. With synchronous programming, each file operation blocks the next operation.
// 同步编程:顺序执行文件操作 for (String filename : filenames) { String data = readFile(filename); writeFile(filename, data); }
Using asynchronous programming, we can use callback notification functions when file operations are completed. This allows the function to continue running while performing other operations.
// 异步编程:并发执行文件操作 CompletableFuture<Void>[] futures = new CompletableFuture[filenames.length]; for (int i = 0; i < filenames.length; i++) { futures[i] = CompletableFuture.runAsync(() -> { String data = readFile(filenames[i]); writeFile(filenames[i], data); }); } CompletableFuture.allOf(futures).join(); // 阻塞等到所有任务完成
Benefits
Asynchronous programming provides many benefits in Java functions:
- Higher concurrency:Execute tasks simultaneously to improve responsiveness.
- Lower latency: Reduce the time you wait for I/O operations to complete.
- Better scalability: Can handle a large number of operations without degrading performance.
Conclusion
By leveraging asynchronous programming, you can significantly improve the performance of your Java functions. By delegating I/O-intensive tasks to dedicated threads or callbacks, you can release the blocking of synchronous programming, resulting in more efficient and responsive applications.
The above is the detailed content of How to enhance the performance of Java functions through asynchronous programming?. For more information, please follow other related articles on the PHP Chinese website!
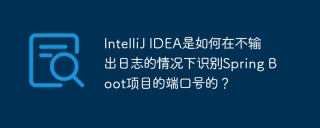
Start Spring using IntelliJIDEAUltimate version...
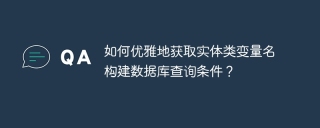
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
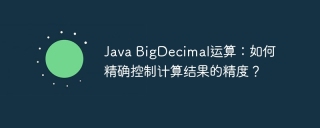
Java...
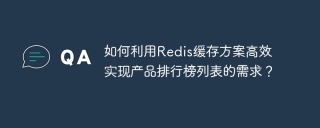
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
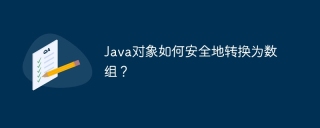
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
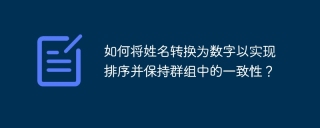
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
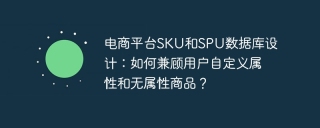
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
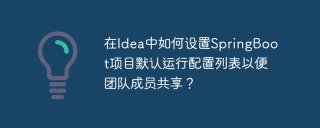
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
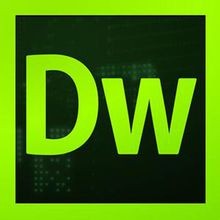
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
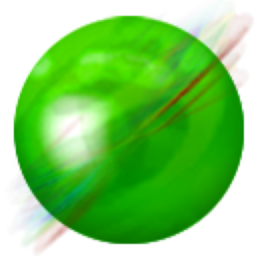
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment