Efficient way to reverse multidimensional PHP array
Two effective ways to reverse multidimensional PHP arrays: Recursively using the array_reverse() function: Recursively reverse the elements of each nested array. PHP 7's array_reverse() function: Reverse multidimensional arrays using the new overload of the array_reverse() function.
Efficient way to reverse multi-dimensional PHP array
When dealing with multi-dimensional arrays in PHP, sometimes you need to reverse the array. This can be used in various scenarios, such as creating a reverse lookup table or simply displaying data in reverse order.
There are many ways to reverse a multi-dimensional array, this article will introduce the two most effective ones:
Using array_reverse()
Recursion
This method uses the array_reverse()
function and recursion to reverse the elements of each nested array.
<?php function reverseArray($array) { if (!is_array($array)) { return $array; } return array_map('reverseArray', array_reverse($array)); }
Using PHP 7’s array_reverse()
PHP 7 introduces a new overload of the array_reverse()
function , which allows inversion of multidimensional arrays.
<?php function reverseArray(array &$array): void { array_reverse($array, true); }
Practical case
To use these methods to reverse a multidimensional array, you can follow these steps:
<?php $array = [ 'name' => 'John Doe', 'profile' => [ 'age' => 30, 'address' => '123 Main Street', ], ]; // 使用递归方法 $reversedArray = reverseArray($array); // 使用 PHP 7 方法 reverseArray($array); print_r($reversedArray); // 显示反转后的数组
Output:
Array ( [name] => John Doe [profile] => Array ( [address] => 123 Main Street [age] => 30 ) )
As you can see, both methods successfully reverse the multidimensional array. You can choose the most appropriate method based on your project's specific requirements.
The above is the detailed content of Efficient way to reverse multidimensional PHP array. For more information, please follow other related articles on the PHP Chinese website!
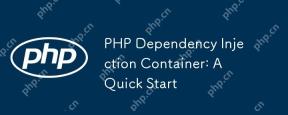
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
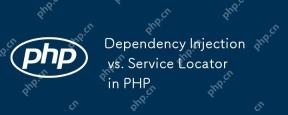
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
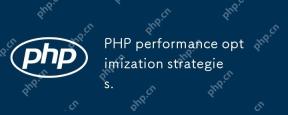
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
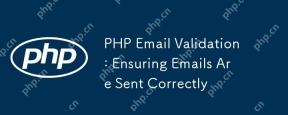
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
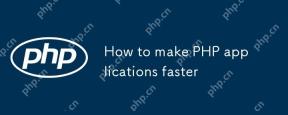
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
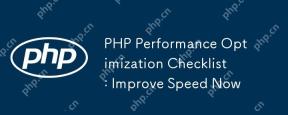
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
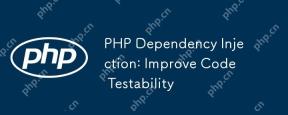
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
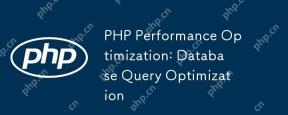
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
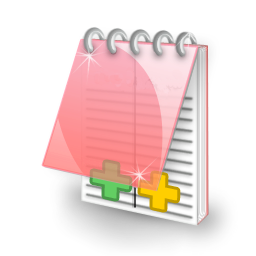
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version
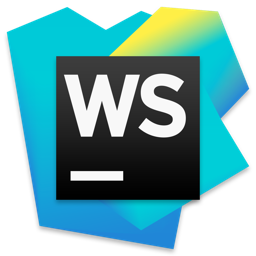
WebStorm Mac version
Useful JavaScript development tools
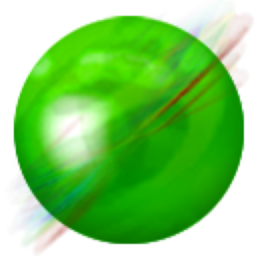
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
