Best practices for golang function definition
Following Go function definition best practices can improve code quality: use clear names to clearly describe function behavior; define appropriate parameter signatures, including required types and order; clearly specify return value types, and handle potential errors; handle Specify concurrency semantics when concurring, use Goroutine and synchronization mechanisms; organize related functions into separate files to improve modularity and maintainability.
Go function definition best practices
Introduction
Functions are important for code organization and reuse in Go component. Following good function definition best practices can improve code readability, maintainability, and performance. This article will introduce the best practices for Go function definition and provide practical examples.
Rule 1: Use clear names
Function names should describe the behavior of the function and avoid abbreviations or generic names. Clear names make it easier to understand the purpose of the code. For example:
// 使用明确的名称 func CalculateTax(amount float64, rate float64) float64 {...} // 与使用通用名称相比 func Calc(amount float64, rate float64) float64 {...}
Rule 2: Define appropriate parameter signatures
Parameter types and order are critical to the correctness of a function. Use types that match the purpose of the function and maintain consistent parameter order. For example:
// 与混合类型的签名相比,使用明确的参数类型 func FormatDate(date time.Time, format string) string {...} // 使用明确的参数顺序,避免混乱 func CreateUser(name string, email string) {...}
Rule 3: Specify return values and handle errors
Explicitly specify the return value type of the function and use error values to handle potential errors. This helps detect problems at compile time and improves code reliability. For example:
// 指定返回值类型 func GetUsername(id int) (string, error) {...} // 使用错误值处理错误 func WriteToFile(filename string, data []byte) error {...}
Rule 4: Handling concurrency
If a function needs to handle concurrency, please specify concurrency semantics explicitly. Use appropriate Goroutines and synchronization mechanisms such as channels, locks, and atomic operations. For example:
// 使用信道进行并发 func ProcessTasks(tasks []func()) { resultCh := make(chan int) for _, task := range tasks { go func() { resultCh <- task() }() } // 从信道中读取结果 ... }
Rule 5: File Organization
Organize related functions into separate files to improve modularity and maintainability. Follow clear file naming conventions and consider using Go modules for version control. For example:
// file: user.go package user func GetUser(id int) (*User, error) {...} func CreateUser(name string, email string) error {...} // file: order.go package order func CreateOrder(customer *User, products []Product) error {...}
Practical case
The following is an example of a Go function defined using best practices:
// file: utils.go package utils // CalculateTax 计算给定金额和税率的税款 func CalculateTax(amount float64, rate float64) float64 { return amount * rate }
This function has an explicit name and parameter signatures, specifying the return value type, and using Go's floating point arithmetic. It is organized into separate utils
files to promote modularity.
Conclusion
Following best practices for Go function definitions can significantly improve code understandability, maintainability, and performance. You can write high-quality Go functions using coding conventions by using clear names, appropriate parameter signatures, specifying return values, and handling errors.
The above is the detailed content of Best practices for golang function definition. For more information, please follow other related articles on the PHP Chinese website!
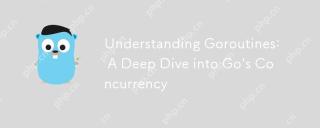
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
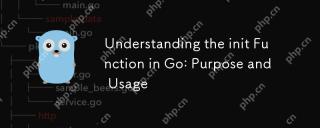
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
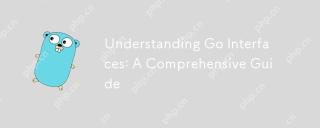
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
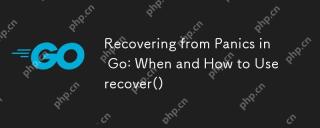
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
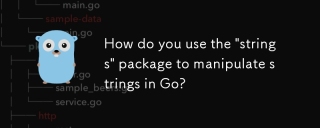
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
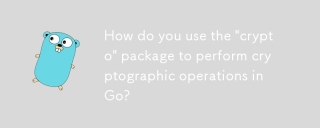
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
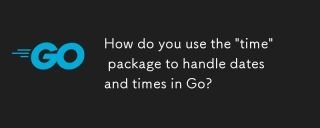
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
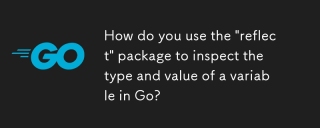
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
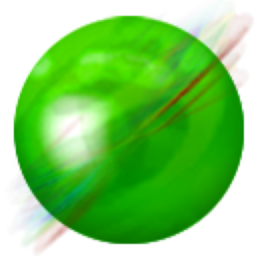
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
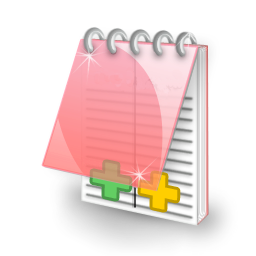
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
