What is the role of Semaphore in Java function concurrency and multi-threading?
Semaphore is a mechanism for controlling multi-threaded resource access in Java concurrent programming. It is implemented by creating a license. The license count is specified during initialization, indicating the number of protected resources that a thread can access at the same time. When a thread attempts to access a resource, it An attempt will be made to obtain a license, and if no license is available, the thread will be blocked until a license is available.
Concurrency of Java functions and the role of Semaphore in multi-threading
Semaphore is an important mechanism in Java concurrent programming, used in multi-threaded scenarios Control access to resources. It does this by creating licenses for the shared data structures that protect the resources.
Principle of action
Semaphore specifies a license count when initialized, which indicates the maximum number of threads that can access protected resources at the same time. When a thread attempts to access a resource, it attempts to obtain a license on the semaphore. If available, the license is granted and the thread can access the resource. If no license is available, the thread will be blocked until a license becomes available.
Practical Case
Suppose we have a shared resource, that is, a queue. Only 5 threads can access the queue simultaneously. We can use a Semaphore to ensure this:
import java.util.concurrent.Semaphore; import java.util.Queue; public class Example { private static final int MAX_ACCESS_COUNT = 5; private static Semaphore semaphore = new Semaphore(MAX_ACCESS_COUNT); private static Queue<Integer> queue = new ConcurrentLinkedQueue<>(); public static void main(String[] args) { for (int i = 0; i < 10; i++) { new Thread(() -> accessQueue()).start(); } } private static void accessQueue() { try { // 尝试获取一个许可证 semaphore.acquire(); // 访问队列 queue.add((int) (Math.random() * 100)); // 释放许可证 semaphore.release(); } catch (InterruptedException e) { e.printStackTrace(); } } }
In this example, the semaphore is initialized with 5 licenses. This ensures that only a maximum of 5 threads can access the queue simultaneously. Other threads will be blocked until a license is available.
Conclusion
Semaphore is very useful in controlling concurrent access to shared resources. It helps prevent data races and inconsistencies by limiting the number of threads that can access a resource simultaneously.
The above is the detailed content of What is the role of Semaphore in Java function concurrency and multi-threading?. For more information, please follow other related articles on the PHP Chinese website!
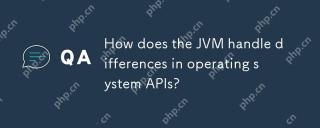
JVM handles operating system API differences through JavaNativeInterface (JNI) and Java standard library: 1. JNI allows Java code to call local code and directly interact with the operating system API. 2. The Java standard library provides a unified API, which is internally mapped to different operating system APIs to ensure that the code runs across platforms.
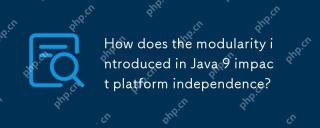
modularitydoesnotdirectlyaffectJava'splatformindependence.Java'splatformindependenceismaintainedbytheJVM,butmodularityinfluencesapplicationstructureandmanagement,indirectlyimpactingplatformindependence.1)Deploymentanddistributionbecomemoreefficientwi
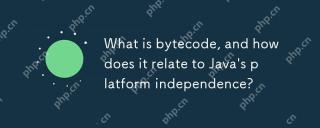
BytecodeinJavaistheintermediaterepresentationthatenablesplatformindependence.1)Javacodeiscompiledintobytecodestoredin.classfiles.2)TheJVMinterpretsorcompilesthisbytecodeintomachinecodeatruntime,allowingthesamebytecodetorunonanydevicewithaJVM,thusfulf
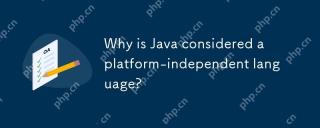
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),whichexecutesbytecodeonanydevicewithaJVM.1)Javacodeiscompiledintobytecode.2)TheJVMinterpretsandexecutesthisbytecodeintomachine-specificinstructions,allowingthesamecodetorunondifferentp
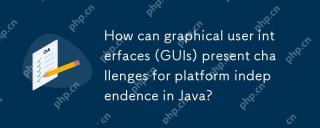
Platform independence in JavaGUI development faces challenges, but can be dealt with by using Swing, JavaFX, unifying appearance, performance optimization, third-party libraries and cross-platform testing. JavaGUI development relies on AWT and Swing, which aims to provide cross-platform consistency, but the actual effect varies from operating system to operating system. Solutions include: 1) using Swing and JavaFX as GUI toolkits; 2) Unify the appearance through UIManager.setLookAndFeel(); 3) Optimize performance to suit different platforms; 4) using third-party libraries such as ApachePivot or SWT; 5) conduct cross-platform testing to ensure consistency.
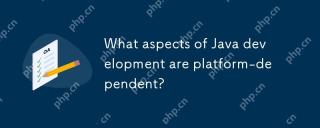
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
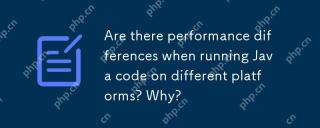
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
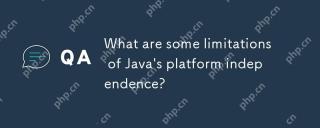
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
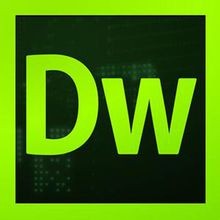
Dreamweaver CS6
Visual web development tools
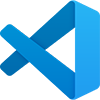
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
