Iterator in Java is a tool used to traverse collections. Usage methods include: Obtaining an Iterator: Use the iterator() method of the Collection interface. Loop through the collection: use hasNext() to check if the next element exists, use next() to get the current element and move to the next element.
Usage of Iterator in Java
What is Iterator?
The Iterator interface is a tool in the Java programming language for traversing collections. It provides a set of methods that allow the programmer to access each element in the collection sequentially.
Usage of Iterator:
-
Get Iterator:
- Use Collection interface
iterator()
Method gets the Iterator of the collection.
- Use Collection interface
-
Loop through the collection:
- Use the
hasNext()
method to check whether the Iterator still exists The next element. - If there is, use the
next()
method to get the current element and move to the next element.
- Use the
Sample code:
// 创建一个字符串集合 Set<String> names = new HashSet<>(); names.add("Alice"); names.add("Bob"); names.add("Carol"); // 获取集合的 Iterator Iterator<String> iterator = names.iterator(); // 循环遍历集合 while (iterator.hasNext()) { String name = iterator.next(); System.out.println(name); }
Advantages:
- Flexibility: Iterator can be used to traverse various types of collections, including List, Set and Map.
- Sequential traversal: Iterator provides an ordered way to traverse the elements in a collection.
- Avoid concurrent modifications: Using Iterator can avoid concurrent modifications to the collection when traversing the collection, thereby preventing data corruption.
Note:
Iterator is a read-only interface and cannot be used to modify elements in the collection. If you need to modify the collection, you need to use other methods, such as List.set()
or Map.put()
.
The above is the detailed content of How to use iterator in java. For more information, please follow other related articles on the PHP Chinese website!
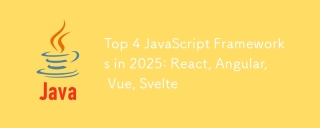
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
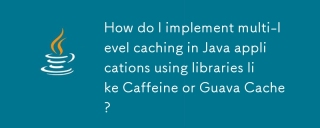
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
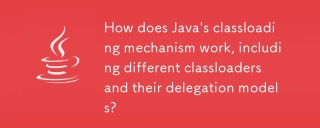
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
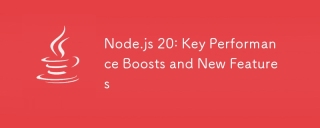
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
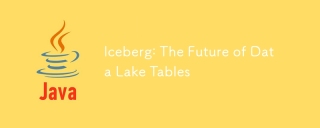
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
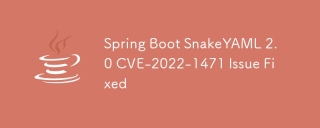
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
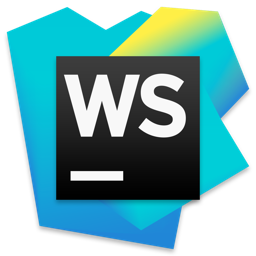
WebStorm Mac version
Useful JavaScript development tools
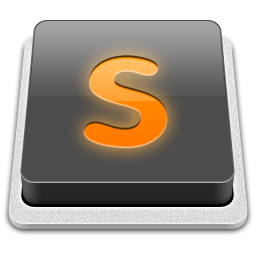
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
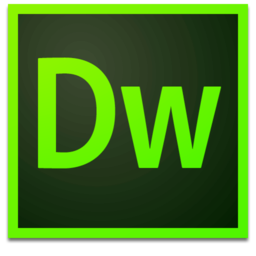
Dreamweaver Mac version
Visual web development tools
