In Java, "Value" usually refers to the value held by an object or variable, including basic types (such as int, double) and reference types (such as objects). Primitive types store actual values, while reference types store references to objects. Objects in Java are passed by reference, and modifications to the reference of the object can affect the original object. Additionally, some classes, such as String, are immutable, meaning their value cannot be changed after creation.
Value in Java
In Java, the word "Value" usually refers to the value held by an object or variable. Some value. These values can be primitive types (such as int, double, boolean) or reference types (such as objects).
Basic Types
Basic types store their values directly and cannot change the referenced value. For example:
int myInt = 10; // myInt 现在包含值 10
Reference type
Reference type stores a reference to an object rather than the actual value of the object. Therefore, the referenced object can be changed. For example:
Integer myInteger = new Integer(10); // myInteger 现在引用一个包含值 10 的 Integer 对象 myInteger = new Integer(20); // myInteger 现在引用一个包含值 20 的 Integer 对象
Value and Reference
In Java, objects are passed by reference. This means that when you pass an object reference, you are actually passing a reference to that object. For example:
public void changeValue(Integer myInteger) { myInteger = new Integer(30); } Integer myInteger = new Integer(10); changeValue(myInteger); System.out.println(myInteger); // 输出:30
In this example, although the value of myInteger
is reassigned in the changeValue
method, this also modifies the actual object passed to the method .
Immutable types
In Java, certain classes (such as wrapper classes for String and Integer) are immutable. This means that once these objects are created, their values cannot be changed.
String myString = "Hello"; // myString 现在包含字符串 "Hello" myString = "World"; // 不会改变 myString 的值,而是创建一个新的 String 对象
The above is the detailed content of What does value mean in java. For more information, please follow other related articles on the PHP Chinese website!
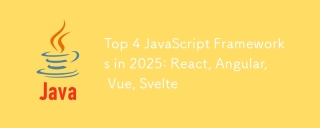
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
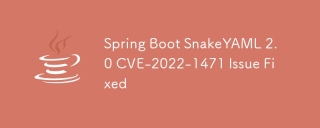
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
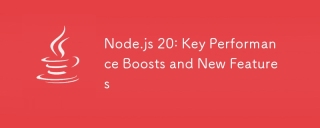
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
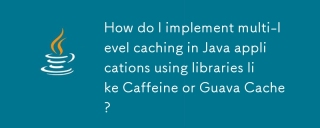
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
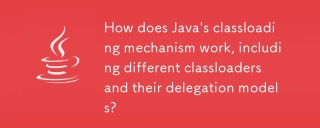
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
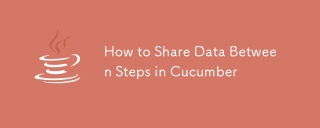
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
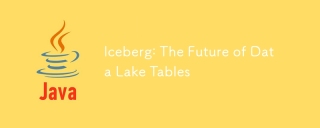
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
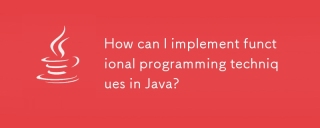
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
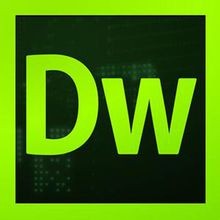
Dreamweaver CS6
Visual web development tools
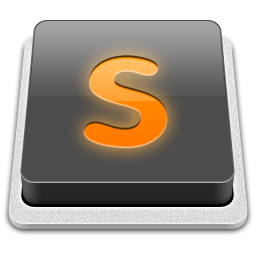
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!
