JVM Internals: Diving Deep into the Java Virtual Machine
JVM的核心组件包括Class Loader、Runtime Data Area和Execution Engine。1) Class Loader负责加载、链接和初始化类和接口。2) Runtime Data Area包含Method Area、Heap、Stack、PC Register和Native Method Stacks。3) Execution Engine由Interpreter、JIT Compiler和Garbage Collector组成,负责 bytecode的执行和优化。
JVM Internals: Diving Deep into the Java Virtual Machine
When it comes to understanding the internals of the Java Virtual Machine (JVM), it's like peeling an onion—there are layers upon layers of fascinating complexity. Let's dive into the heart of JVM and explore its inner workings, sharing some personal insights and experiences along the way.
Java's magic lies in its ability to run anywhere, thanks to the JVM. But what exactly happens under the hood when we execute a Java program? Let's break it down and see how the JVM transforms our code into something that can run on any machine.
The JVM is essentially a runtime environment that converts Java bytecode into machine-specific instructions. This process involves several key components: the Class Loader, the Runtime Data Area, and the Execution Engine. Each plays a crucial role in executing our Java applications.
Let's start with the Class Loader. When you run a Java program, the Class Loader is the first to spring into action. It's responsible for loading, linking, and initializing classes and interfaces. Imagine it as a librarian, meticulously organizing and retrieving books (classes) from the shelves (classpath). I've seen many developers underestimate the importance of the Class Loader, only to run into issues with class loading conflicts in larger projects. My advice? Always be mindful of your classpath and understand how different Class Loaders work together.
// 示例:简单的类加载器 public class CustomClassLoader extends ClassLoader { @Override protected Class> findClass(String name) throws ClassNotFoundException { byte[] classData = loadClassData(name); if (classData == null) { throw new ClassNotFoundException(); } return defineClass(name, classData, 0, classData.length); } <pre class='brush:php;toolbar:false;'>private byte[] loadClassData(String className) { // 这里可以实现从文件系统或网络加载类 // 为了简洁,这里返回 null return null; }
}
Now, let's move to the Runtime Data Area, where the JVM stores data during program execution. This area is divided into several parts: the Method Area, the Heap, the Stack, the Program Counter (PC) Register, and Native Method Stacks. The Heap is where objects live, and managing it efficiently is crucial for performance. I've spent countless hours optimizing heap usage in applications, and one thing I've learned is that understanding garbage collection algorithms can save you from many headaches.
// 示例:简单展示堆内存分配 public class HeapExample { public static void main(String[] args) { // 分配一个大对象 byte[] largeObject = new byte[1024 * 1024]; // 1MB System.out.println("Allocated large object"); } }
The Execution Engine is where the magic happens. It includes the Interpreter, the Just-In-Time (JIT) Compiler, and the Garbage Collector. The Interpreter reads bytecode and executes it line by line, which is great for starting up quickly but can be slow for long-running applications. The JIT Compiler, on the other hand, translates bytecode into native machine code at runtime, which can significantly boost performance. Balancing the use of the Interpreter and JIT Compiler is an art, and I've seen applications go from sluggish to lightning-fast with the right tuning.
// 示例:展示JIT编译的影响 public class JITExample { public static void main(String[] args) { long start = System.currentTimeMillis(); for (int i = 0; i }
Understanding the JVM's garbage collection mechanisms is crucial. The JVM uses various algorithms like Serial GC, Parallel GC, CMS (Concurrent Mark Sweep), and G1 (Garbage First) to manage memory. Each has its strengths and weaknesses, and choosing the right one can be a game-changer for your application's performance. I once worked on a project where switching from CMS to G1 reduced pause times significantly, improving the user experience dramatically.
// 示例:显示如何设置垃圾收集器 public class GCExample { public static void main(String[] args) { // 设置G1垃圾收集器 System.setProperty("java.vm.info", "G1 GC"); // 这里可以添加更多的GC参数 System.out.println("Using G1 Garbage Collector"); } }
When it comes to performance optimization, profiling tools are your best friends. I've used tools like VisualVM and JProfiler to identify bottlenecks and optimize JVM settings. One common pitfall I've encountered is over-optimizing—sometimes, the simplest solution is the best. Always measure before and after making changes to ensure you're actually improving performance.
In my experience, the key to mastering JVM internals is continuous learning and experimentation. The JVM is a complex beast, and staying updated with its latest features and optimizations can give your applications a significant edge. Whether you're dealing with memory management, optimizing garbage collection, or fine-tuning JIT compilation, understanding the JVM internals will make you a better Java developer.
So, next time you're debugging a Java application or trying to squeeze out that last bit of performance, remember the intricate dance happening inside the JVM. It's not just about writing code; it's about understanding how that code is executed and optimized at runtime. Dive deep into the JVM, and you'll unlock a world of possibilities for your Java applications.
The above is the detailed content of JVM Internals: Diving Deep into the Java Virtual Machine. For more information, please follow other related articles on the PHP Chinese website!
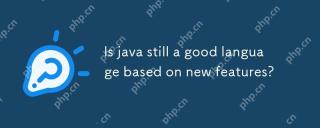
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
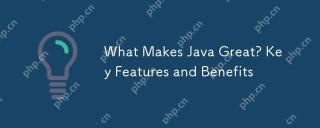
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
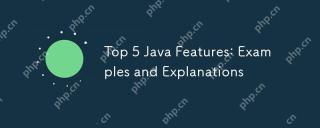
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.
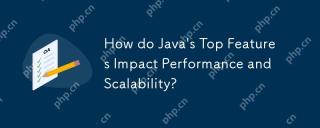
Java'stopfeaturessignificantlyenhanceitsperformanceandscalability.1)Object-orientedprincipleslikepolymorphismenableflexibleandscalablecode.2)Garbagecollectionautomatesmemorymanagementbutcancauselatencyissues.3)TheJITcompilerboostsexecutionspeedafteri
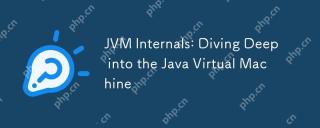
The core components of the JVM include ClassLoader, RuntimeDataArea and ExecutionEngine. 1) ClassLoader is responsible for loading, linking and initializing classes and interfaces. 2) RuntimeDataArea contains MethodArea, Heap, Stack, PCRegister and NativeMethodStacks. 3) ExecutionEngine is composed of Interpreter, JITCompiler and GarbageCollector, responsible for the execution and optimization of bytecode.
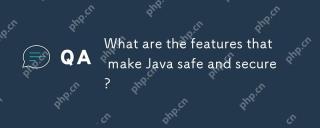
Java'ssafetyandsecurityarebolsteredby:1)strongtyping,whichpreventstype-relatederrors;2)automaticmemorymanagementviagarbagecollection,reducingmemory-relatedvulnerabilities;3)sandboxing,isolatingcodefromthesystem;and4)robustexceptionhandling,ensuringgr
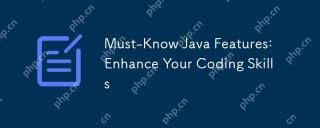
Javaoffersseveralkeyfeaturesthatenhancecodingskills:1)Object-orientedprogrammingallowsmodelingreal-worldentities,exemplifiedbypolymorphism.2)Exceptionhandlingprovidesrobusterrormanagement.3)Lambdaexpressionssimplifyoperations,improvingcodereadability
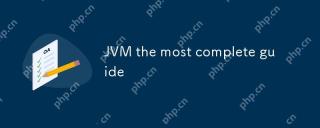
TheJVMisacrucialcomponentthatrunsJavacodebytranslatingitintomachine-specificinstructions,impactingperformance,security,andportability.1)TheClassLoaderloads,links,andinitializesclasses.2)TheExecutionEngineexecutesbytecodeintomachineinstructions.3)Memo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
