The embodiment of polymorphism in C++ function overloading and rewriting
Polymorphism in C: Function overloading allows multiple functions with the same name but different argument lists, with the function chosen to be executed based on the argument types when called. Function overriding allows a derived class to redefine methods that already exist in the base class, thereby achieving different types of behavior, depending on the type of object.
The embodiment of polymorphism in C function overloading and rewriting
Polymorphism is a key concept in object-oriented programming one. It allows objects of different types (derived classes) to respond differently to the same function call. C implements polymorphism through function overloading and overriding.
Function overloading
Function overloading refers to multiple functions with the same name but different parameter lists. The compiler will choose the correct function based on the argument types when it is actually called. For example, the following code overloads the area()
function, which can calculate the area of a circle or rectangle:
class Circle { public: double area(double radius) { return 3.14159 * radius * radius; } }; class Rectangle { public: double area(double length, double width) { return length * width; } };
Override
Override It refers to redefining methods in the derived class that already exist in the base class. It allows derived classes to provide their own implementations, enabling different types of behavior. For example, the following code overrides the area()
method of the base class Rectangle
in the derived class Square
to calculate the area of a square:
class Rectangle { public: virtual double area(double length, double width) { return length * width; } }; class Square : public Rectangle { public: virtual double area(double side) override { return side * side; } };
Practical case
Consider a graphics library with a Shape
base class and Circle
, Rectangle
and Square
Derived classes. We want to create a function draw()
to draw different graphics. By using overloads, we can provide a different draw()
method to handle each shape type:
struct IShape { virtual void draw() = 0; }; struct Circle : public IShape { void draw() override { // 代码绘制圆 } }; struct Rectangle : public IShape { void draw() override { // 代码绘制矩形 } }; struct Square : public Rectangle { void draw() override { // 代码绘制正方形 } };
When calling the draw()
method, C will Choose the correct function version based on the type of actual object. This allows us to write generic code to handle different types of graphics without the need for explicit conversions or casts.
The above is the detailed content of The embodiment of polymorphism in C++ function overloading and rewriting. For more information, please follow other related articles on the PHP Chinese website!
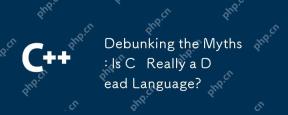
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
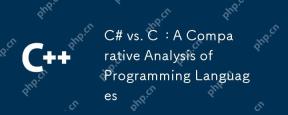
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
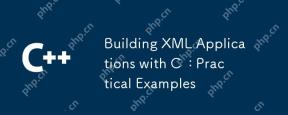
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
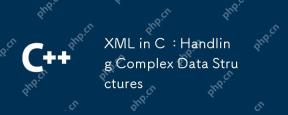
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
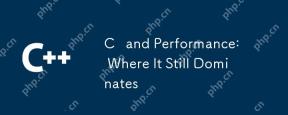
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
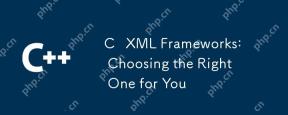
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
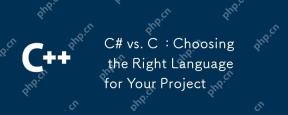
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
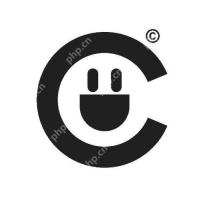
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
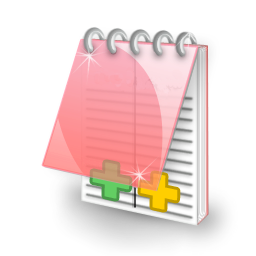
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
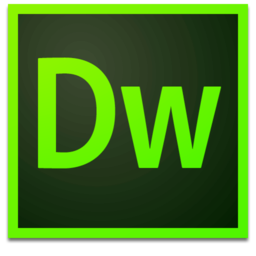
Dreamweaver Mac version
Visual web development tools
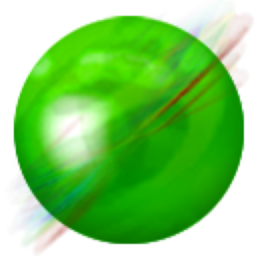
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
