How to identify and fix memory leaks in Java functions?
To find memory leaks in Java, use JVisualVM or JConsole to monitor JVM memory usage and reference graph; fix memory leaks, the most common cause is object references, you can release object references that are no longer needed by using weak references or phantom references , thereby solving the memory leak problem.
How to find and fix memory leaks in Java functions
Memory leaks are a problem that developers often encounter and can cause The application slows down over time and eventually crashes. In Java, memory leaks usually occur when one object references another object, even when it is no longer needed.
Identifying Memory Leaks
The first step in identifying memory leaks is to use the tools provided by the Java Virtual Machine (JVM). The most commonly used tools are Java VisualVM or JConsole. These tools allow you to monitor JVM memory usage and reference graphs.
-
Using JVisualVM
Start JVisualVM and connect to a running Java application. Navigate to the Summary tab and look at the Memory section. Look for growing heap size or frequent garbage collection cycles.
-
Using JConsole
Start JConsole and connect to a running Java application. Navigate to the Memory tab and look at the Heap section. Look for growing heap size or frequent young generation collections.
Fixing memory leaks
After identifying a memory leak, the next step is to fix it. The most common cause is object references. To fix this problem, make sure to release the reference to the object when it is no longer needed.
-
Using Weak References
Weak references will not prevent the garbage collector from reclaiming the object. They are used when an object is no longer needed but still needs to maintain some reference to it.
WeakReference<Object> weakRef = new WeakReference<>(object);
-
Using Phantom References
Ghost references are similar to weak references, but they are retrieved after the object is recycled Garbage collection. This can be used to perform certain operations after the object has been recycled.
PhantomReference<Object> phantomRef = new PhantomReference<>(object, new PhantomReference<>(...);
Practical case
Consider the following code snippet:
public class MemoryLeakExample { private static List<Object> objects = new ArrayList<>(); public static void main(String[] args) { // 创建一个大量对象并将其添加到列表中 for (int i = 0; i < 1000000; i++) { objects.add(new Object()); } // 故意不释放对象引用 } }
This code will create a large number of objects and add them to the list. However, it does not release the reference to the object, which will cause a memory leak.
To fix this problem, we can use weak references:
private static List<WeakReference<Object>> objects = new ArrayList<>();
This way, when the objects are no longer needed, their references can be automatically released.
The above is the detailed content of How to identify and fix memory leaks in Java functions?. For more information, please follow other related articles on the PHP Chinese website!
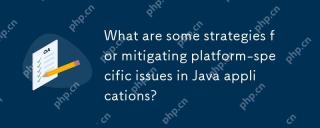
How does Java alleviate platform-specific problems? Java implements platform-independent through JVM and standard libraries. 1) Use bytecode and JVM to abstract the operating system differences; 2) The standard library provides cross-platform APIs, such as Paths class processing file paths, and Charset class processing character encoding; 3) Use configuration files and multi-platform testing in actual projects for optimization and debugging.
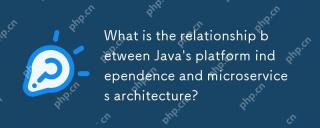
Java'splatformindependenceenhancesmicroservicesarchitecturebyofferingdeploymentflexibility,consistency,scalability,andportability.1)DeploymentflexibilityallowsmicroservicestorunonanyplatformwithaJVM.2)Consistencyacrossservicessimplifiesdevelopmentand
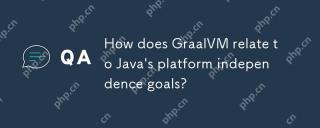
GraalVM enhances Java's platform independence in three ways: 1. Cross-language interoperability, allowing Java to seamlessly interoperate with other languages; 2. Independent runtime environment, compile Java programs into local executable files through GraalVMNativeImage; 3. Performance optimization, Graal compiler generates efficient machine code to improve the performance and consistency of Java programs.
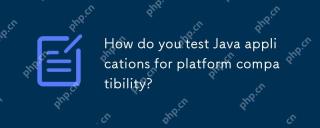
ToeffectivelytestJavaapplicationsforplatformcompatibility,followthesesteps:1)SetupautomatedtestingacrossmultipleplatformsusingCItoolslikeJenkinsorGitHubActions.2)ConductmanualtestingonrealhardwaretocatchissuesnotfoundinCIenvironments.3)Checkcross-pla
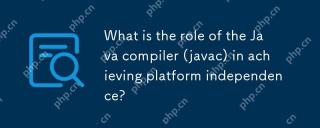
The Java compiler realizes Java's platform independence by converting source code into platform-independent bytecode, allowing Java programs to run on any operating system with JVM installed.
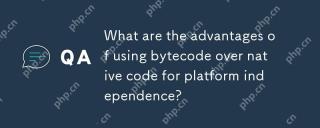
Bytecodeachievesplatformindependencebybeingexecutedbyavirtualmachine(VM),allowingcodetorunonanyplatformwiththeappropriateVM.Forexample,JavabytecodecanrunonanydevicewithaJVM,enabling"writeonce,runanywhere"functionality.Whilebytecodeoffersenh

Java cannot achieve 100% platform independence, but its platform independence is implemented through JVM and bytecode to ensure that the code runs on different platforms. Specific implementations include: 1. Compilation into bytecode; 2. Interpretation and execution of JVM; 3. Consistency of the standard library. However, JVM implementation differences, operating system and hardware differences, and compatibility of third-party libraries may affect its platform independence.
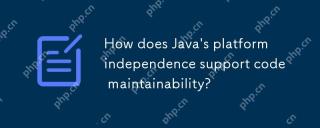
Java realizes platform independence through "write once, run everywhere" and improves code maintainability: 1. High code reuse and reduces duplicate development; 2. Low maintenance cost, only one modification is required; 3. High team collaboration efficiency is high, convenient for knowledge sharing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
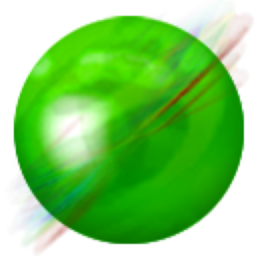
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
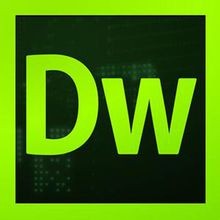
Dreamweaver CS6
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
