How do you test Java applications for platform compatibility?
To effectively test Java applications for platform compatibility, follow these steps: 1) Set up automated testing across multiple platforms using CI tools like Jenkins or GitHub Actions. 2) Conduct manual testing on real hardware to catch issues not found in CI environments. 3) Check cross-platform compatibility of libraries and dependencies. 4) Use environment-specific configurations to handle platform differences. 5) Monitor performance and resource utilization across platforms to optimize application behavior.
Testing Java applications for platform compatibility is crucial to ensure that your software runs smoothly across different operating systems and environments. Let's dive into how you can effectively test for platform compatibility, sharing some personal experiences and insights along the way.
When I first started working on Java projects, I quickly realized that what works perfectly on my development machine might not behave the same way on a client's Windows server or a colleague's Mac. This realization led me to develop a robust testing strategy that I'll share with you.
To begin with, understanding the nuances of Java's "write once, run anywhere" philosophy is key. Java's platform independence is a double-edged sword; while it promises broad compatibility, real-world applications often encounter platform-specific issues. Here's how you can tackle these challenges:
Automated Testing Across Multiple Platforms
Setting up a continuous integration (CI) pipeline that includes testing on different operating systems is a game-changer. Tools like Jenkins or GitHub Actions can be configured to run your tests on Windows, macOS, and various Linux distributions. Here's a snippet of how you might configure a GitHub Actions workflow to test on multiple platforms:
name: Java CI on: [push, pull_request] jobs: build: runs-on: ${{ matrix.os }} strategy: matrix: os: [ubuntu-latest, windows-latest, macos-latest] java: [11, 17] steps: - uses: actions/checkout@v2 - name: Set up JDK uses: actions/setup-java@v2 with: java-version: ${{ matrix.java }} - name: Build with Maven run: mvn -B package --file pom.xml - name: Run Tests run: mvn test
This approach ensures that your application is tested in environments that closely mimic your target platforms. However, remember that CI environments might not perfectly replicate production environments, so consider using containerization with Docker to further simulate different setups.
Manual Testing and Real-World Scenarios
While automated tests are essential, nothing beats manual testing on actual hardware. I've found that setting up a few machines or virtual machines with different OS configurations can uncover issues that automated tests might miss. For instance, I once encountered a font rendering issue on Windows that was not apparent in our CI pipeline. Manual testing on a real Windows machine helped us identify and fix this problem.
Cross-Platform Libraries and Dependencies
Java's ecosystem is rich with libraries, but not all are created equal when it comes to cross-platform compatibility. Always check the documentation of third-party libraries for any known platform-specific issues. I've had experiences where a library worked flawlessly on Linux but caused memory leaks on Windows. Regularly updating dependencies and keeping an eye on their compatibility reports can save you from such headaches.
Environment-Specific Configurations
Sometimes, your application might need different configurations for different platforms. Java's system properties and environment variables can be used to handle these differences. Here's an example of how you might use system properties to configure your application:
public class AppConfig { private static final String OS_NAME = System.getProperty("os.name").toLowerCase(); public static boolean isWindows() { return OS_NAME.contains("win"); } public static boolean isMac() { return OS_NAME.contains("mac"); } public static boolean isUnix() { return OS_NAME.contains("nix") || OS_NAME.contains("nux") || OS_NAME.contains("aix"); } public static void configureForPlatform() { if (isWindows()) { // Windows-specific configuration } else if (isMac()) { // macOS-specific configuration } else if (isUnix()) { // Unix/Linux-specific configuration } } }
This approach allows you to tailor your application's behavior to the specific platform it's running on, which can be crucial for handling platform-specific quirks.
Performance and Resource Utilization
Different platforms can have varying performance characteristics. I've seen applications that run smoothly on Linux but struggle with memory on Windows due to different JVM implementations. Using profiling tools like VisualVM or JProfiler can help you identify and optimize these differences. Here's a simple example of how you might use VisualVM to monitor your application:
public class PerformanceTest { public static void main(String[] args) { // Start VisualVM and attach it to this JVM for (int i = 0; i < 1000000; i ) { // Some intensive operation String s = "Hello, World!"; s = s.toUpperCase(); } } }
Challenges and Pitfalls
Testing for platform compatibility isn't without its challenges. One common pitfall is assuming that because your application works on one version of an OS, it will work on all versions. I've learned the hard way that even minor OS updates can introduce breaking changes. Always test on the latest versions of each OS, as well as a few older versions to ensure backward compatibility.
Another challenge is dealing with different file systems and path separators. Java's File
and Path
classes handle this well, but you might still encounter issues with third-party libraries that don't respect these abstractions. Always test file operations thoroughly across different platforms.
Conclusion
Testing Java applications for platform compatibility is an ongoing process that requires a combination of automated and manual testing, careful management of dependencies, and an understanding of platform-specific configurations. By following these strategies, you can ensure that your Java application runs smoothly across different environments, providing a seamless experience for all users. Remember, the key to successful platform compatibility testing is to never assume, always verify.
The above is the detailed content of How do you test Java applications for platform compatibility?. For more information, please follow other related articles on the PHP Chinese website!
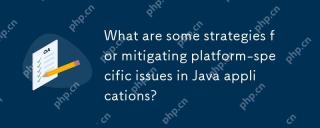
How does Java alleviate platform-specific problems? Java implements platform-independent through JVM and standard libraries. 1) Use bytecode and JVM to abstract the operating system differences; 2) The standard library provides cross-platform APIs, such as Paths class processing file paths, and Charset class processing character encoding; 3) Use configuration files and multi-platform testing in actual projects for optimization and debugging.
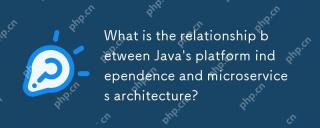
Java'splatformindependenceenhancesmicroservicesarchitecturebyofferingdeploymentflexibility,consistency,scalability,andportability.1)DeploymentflexibilityallowsmicroservicestorunonanyplatformwithaJVM.2)Consistencyacrossservicessimplifiesdevelopmentand
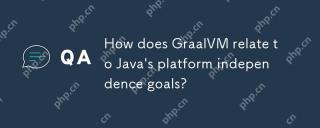
GraalVM enhances Java's platform independence in three ways: 1. Cross-language interoperability, allowing Java to seamlessly interoperate with other languages; 2. Independent runtime environment, compile Java programs into local executable files through GraalVMNativeImage; 3. Performance optimization, Graal compiler generates efficient machine code to improve the performance and consistency of Java programs.
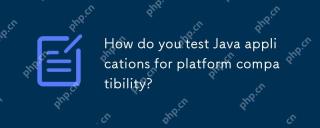
ToeffectivelytestJavaapplicationsforplatformcompatibility,followthesesteps:1)SetupautomatedtestingacrossmultipleplatformsusingCItoolslikeJenkinsorGitHubActions.2)ConductmanualtestingonrealhardwaretocatchissuesnotfoundinCIenvironments.3)Checkcross-pla
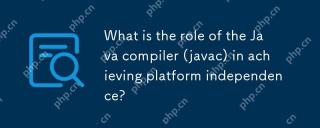
The Java compiler realizes Java's platform independence by converting source code into platform-independent bytecode, allowing Java programs to run on any operating system with JVM installed.
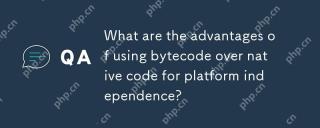
Bytecodeachievesplatformindependencebybeingexecutedbyavirtualmachine(VM),allowingcodetorunonanyplatformwiththeappropriateVM.Forexample,JavabytecodecanrunonanydevicewithaJVM,enabling"writeonce,runanywhere"functionality.Whilebytecodeoffersenh

Java cannot achieve 100% platform independence, but its platform independence is implemented through JVM and bytecode to ensure that the code runs on different platforms. Specific implementations include: 1. Compilation into bytecode; 2. Interpretation and execution of JVM; 3. Consistency of the standard library. However, JVM implementation differences, operating system and hardware differences, and compatibility of third-party libraries may affect its platform independence.
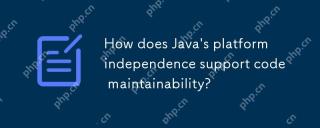
Java realizes platform independence through "write once, run everywhere" and improves code maintainability: 1. High code reuse and reduces duplicate development; 2. Low maintenance cost, only one modification is required; 3. High team collaboration efficiency is high, convenient for knowledge sharing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
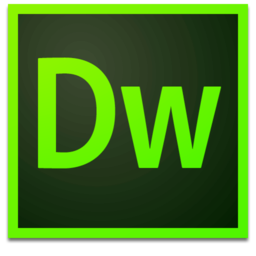
Dreamweaver Mac version
Visual web development tools
