


What are the implementation methods of parallel programming in Java? How to choose?
Java parallel programming implementation methods: 1. Multi-threading, 2. Thread pool, 3. Lock, 4. Atomic variables. Choosing the appropriate method depends on the requirements, for example: High throughput: multi-threading or thread pool Low response time: threads Pool or atomic variable resources are limited: thread pool or lock
Java parallel programming implementation
Java provides a variety of Mechanisms to implement parallel programming, including:
- Multi-threading: Create and run multiple threads of simultaneous execution.
- Thread pool: Manage and reuse threads to improve performance.
- Lock: Used to coordinate access to shared resources and prevent conflicts.
- Atomic variables: Provides thread-safe variables for update operations.
How to choose the appropriate implementation method?
Choosing the appropriate parallel programming implementation depends on the needs of the application:
- High throughput: Multiple threads or thread pools.
- Low response time: Thread pool or atomic variables.
- Resource limited: Thread pool or lock.
Practical case:
Use thread pool to improve throughput:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class ThreadPoolDemo { public static void main(String[] args) { // 创建一个固定大小的线程池 ExecutorService executor = Executors.newFixedThreadPool(10); // 创建任务 Runnable task = () -> { System.out.println("Hello from thread " + Thread.currentThread().getName()); }; // 提交任务到线程池 for (int i = 0; i < 100; i++) { executor.submit(task); } // 等待所有任务完成 executor.shutdown(); while (!executor.isTerminated()) { try { Thread.sleep(100); } catch (InterruptedException e) { e.printStackTrace(); } } } }
Use atomic variables Implement thread safety:
import java.util.concurrent.atomic.AtomicInteger; public class AtomicVariableDemo { public static void main(String[] args) { // 创建一个原子整数 AtomicInteger counter = new AtomicInteger(0); // 两个线程同时更新计数器 Thread thread1 = new Thread(() -> { for (int i = 0; i < 100000; i++) { counter.incrementAndGet(); } }); Thread thread2 = new Thread(() -> { for (int i = 0; i < 100000; i++) { counter.incrementAndGet(); } }); thread1.start(); thread2.start(); // 等待线程完成 try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } // 打印最终计数器值 System.out.println("Final count: " + counter.get()); } }
The above is the detailed content of What are the implementation methods of parallel programming in Java? How to choose?. For more information, please follow other related articles on the PHP Chinese website!
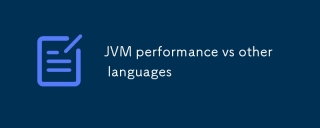
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
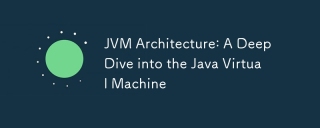
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
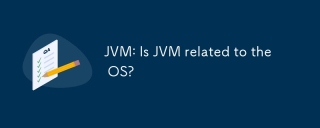
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
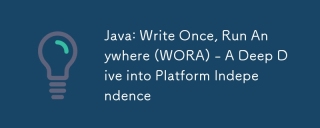
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
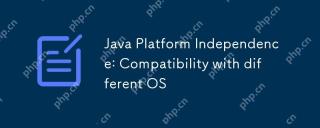
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
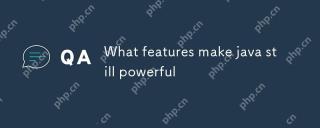
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
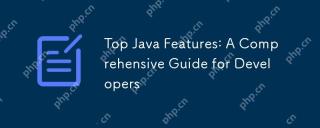
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
