What are the specific ways to improve function performance in C++?
Five ways to improve the performance of C functions: inline functions (embed code into the caller); reduce function parameter passing (only pass necessary parameters); use constant references (pass constant references of function parameters); optimize loops ( Use iterators or range loops); use local variables (access global variables or frequently obtain local variable addresses).
Specific methods to improve function performance in C
Improving function performance is the key to improving the overall performance of C programs. This article will introduce some practical ways to optimize functions to achieve better efficiency.
1. Inline functions
Inline functions embed the function code directly into the caller, eliminating the overhead of function calls. When the function body is small and called frequently, using inlining can bring significant performance improvements.
inline int add(int a, int b) { return a + b; }
2. Reduce function parameters
Passing a large number of parameters to the function will increase the code size and function call overhead. Reduce the number of function parameters as much as possible and only pass necessary parameters.
// 原始函数 int sum(std::vector<int>& numbers, int start, int end) { // 优化函数 int sum(std::vector<int>& numbers, int start = 0, int end = -1) {
3. Use constant references
const references are used to pass constant references to function parameters to avoid unnecessary copying. This is especially important for large data structures.
void print_vector(const std::vector<int>& numbers) { for (const int& number : numbers) { std::cout << number << " "; } }
4. Optimize loops
Loops are a common time-consuming part of the code. Use efficient looping constructs such as iterators or range loops, and avoid unnecessary loop iterations.
// 原始循环 for (int i = 0; i < numbers.size(); i++) { std::cout << numbers[i] << " "; } // 优化循环 for (const int& number : numbers) { std::cout << number << " "; }
5. Using local variables
Accessing global variables or frequently obtaining the address of local variables will cause performance degradation. Copying variables into function local variables improves access speed.
// 原始函数 int sum(const std::vector<int>& numbers) { int total = 0; for (int i = 0; i < numbers.size(); i++) { total += numbers[i]; } return total; } // 优化函数 int sum(const std::vector<int>& numbers) { int& total = const_cast<int&>(total); for (int i = 0; i < numbers.size(); i++) { total += numbers[i]; } return total; }
Practical case
The following is an example of using inline functions to optimize code:
// 原始函数 int factorial(int n) { if (n == 0) { return 1; } return n * factorial(n - 1); } // 优化函数 inline int factorial(int n) { if (n == 0) { return 1; } return n * factorial(n - 1); }
When compiling and running this code, after optimization The version (with inline functions) shows significant performance improvements, especially when large factorials need to be calculated.
The above is the detailed content of What are the specific ways to improve function performance in C++?. For more information, please follow other related articles on the PHP Chinese website!
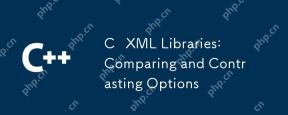
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
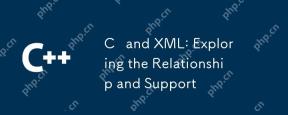
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
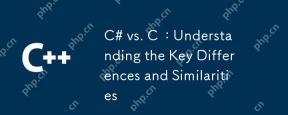
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
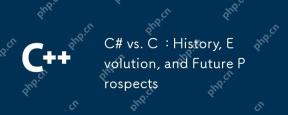
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
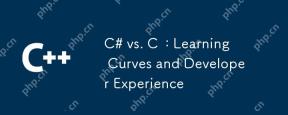
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
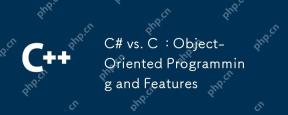
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
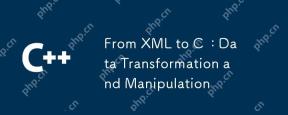
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
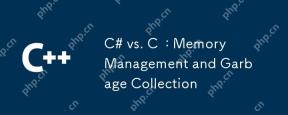
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
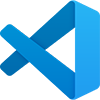
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor