Function pointers and callback functions are both tools for implementing the callback mechanism. Function pointers are created at compile time and cannot be modified and need to be called explicitly; callback functions are created at runtime and can be dynamically bound to different functions and automatically called by the callback function. Therefore, function pointers are suitable for static callbacks, while callback functions are suitable for dynamic callbacks.
Comparison of C function pointers and callback functions
Function pointers and callback functions are both powerful tools used to implement the callback mechanism in C.
Function pointer
- is a pointer variable pointing to a function.
- Created at compile time and cannot be changed at runtime.
- Requires explicit call.
Callback function
- is a function that accepts a function pointer as a parameter.
- Created at runtime and can be dynamically bound to different functions.
- Automatically called by the callback function.
Practical Case
Consider an application that needs to perform different tasks at different times. We can achieve this functionality using the following code:
#include <iostream> // 定义一个打印消息的函数 void print_message(const char* message) { std::cout << message << std::endl; } // 定义一个接受函数指针参数的回调函数 void execute_callback(void (*callback)(const char*)) { callback("Hello world!"); } int main() { // 使用函数指针调用回调函数 execute_callback(print_message); // 动态创建并调用回调函数 auto lambda = [](const char* message) { std::cout << "[Lambda] " << message << std::endl; }; execute_callback(lambda); return 0; }
In this example, print_message
is a function pointer for static callbacks. A lambda expression lambda
is a dynamic callback that is created at runtime and bound to execute_callback
.
Main difference
Features | Function pointer | Callback function |
---|---|---|
Creation timing | Compile time | Run time |
Unmodifiable | Can be modified | |
Explicit | Automatic | |
static | dynamic |
The above is the detailed content of Comparison of C++ function pointers and callback functions. For more information, please follow other related articles on the PHP Chinese website!
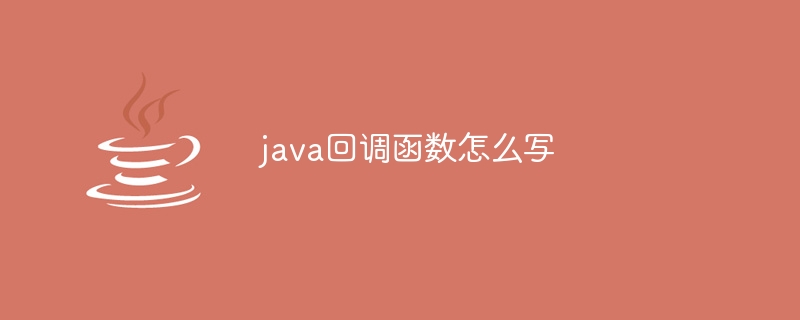
java回调函数的写法有:1、接口回调,定义一个接口,其中包含一个回调方法,在需要触发回调的地方,使用该接口作为参数,并在合适的时机调用回调方法;2、匿名内部类回调,可以使用匿名内部类来实现回调函数,避免创建额外的实现类;3、Lambda表达式回调,在Java 8及以上版本中,可以使用Lambda表达式来简化回调函数的写法等。
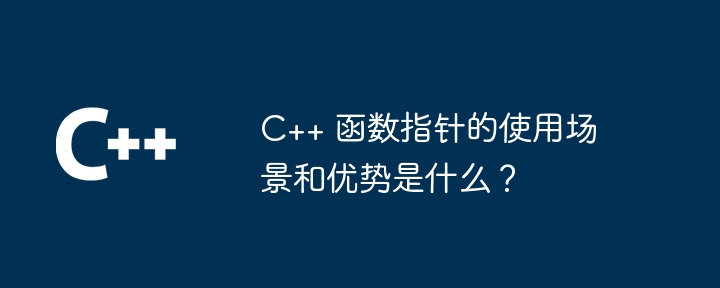
函数指针允许存储对函数的引用,提供额外的灵活性。使用场景包括事件处理、算法排序、数据转换和动态多态。优势包括灵活性、解耦、代码重用和性能优化。实际应用包括事件处理、算法排序和数据转换。凭借函数指针,C++程序员可以创建灵活且动态的代码。
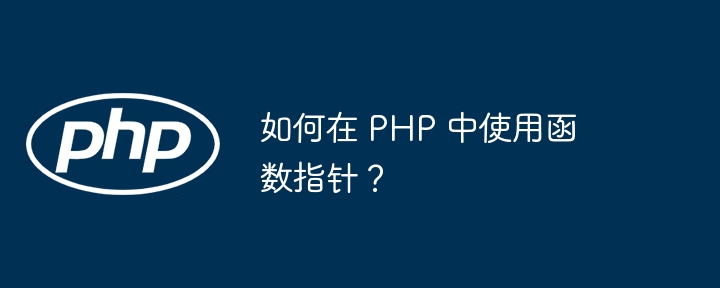
在PHP中,函数指针是称为回调函数的变量,指向函数地址。它允许动态处理函数:语法:$functionPointer='function_name'实战案例:对数组执行操作:usort($numbers,'sortAscending')作为函数参数:array_map(function($string){...},$strings)注意:函数指针指向函数名称,必须与指定的类型匹配,并确保指向的函数始终存在。
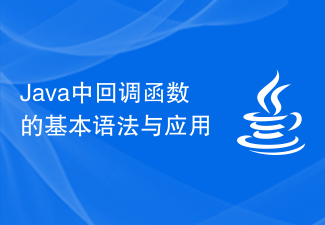
Java回调函数的基本写法和使用方法引言:在Java编程中,回调函数是一种常见的编程模式,通过回调函数,可以将某个方法作为参数传递给另一个方法,从而实现方法的间接调用。回调函数的使用,在事件驱动、异步编程和接口实现等场景中非常常见。本文将介绍Java回调函数的基本写法和使用方法,并提供具体的代码示例。一、回调函数的定义回调函数是一种特殊的函数,它可以作为参数
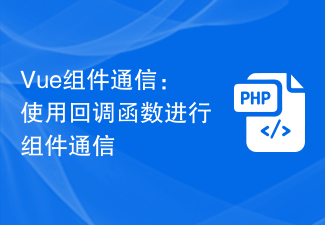
Vue组件通信:使用回调函数进行组件通信在Vue应用程序中,有时候我们需要让不同的组件之间进行通信,以便它们可以共享信息和相互协作。Vue提供了多种方式来实现组件之间的通信,其中一种常用的方式是使用回调函数。回调函数是一种将一个函数作为参数传递给另一个函数并在特定事件发生时被调用的机制。在Vue中,我们可以利用回调函数来实现组件之间的通信,使得一个组件可以在
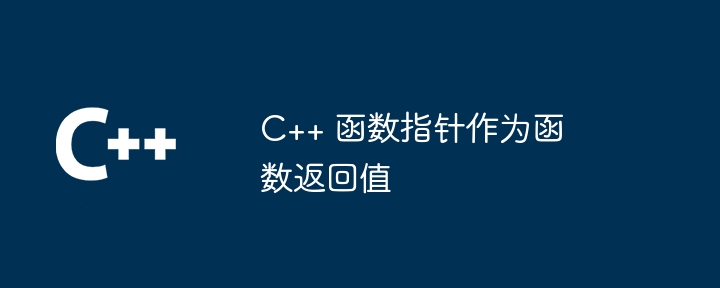
函数指针可以作为函数返回值,允许我们在运行时确定要调用的函数。语法为:returntype(*function_name)(param1,param2,...)。优点包括动态绑定和回调机制,使我们可以根据需要调整函数调用。
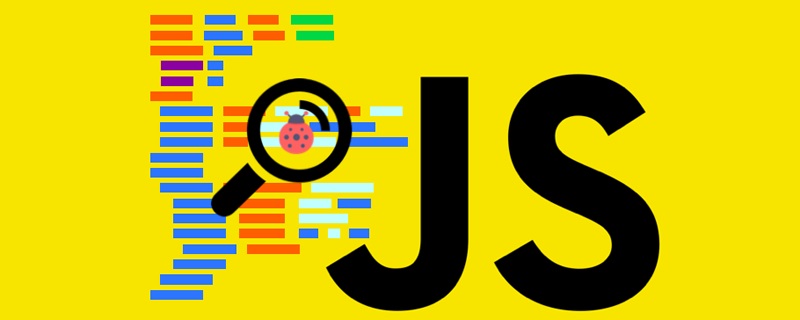
回调函数是每个前端程序员都应该知道的概念之一。回调可用于数组、计时器函数、promise、事件处理中。本文将会解释回调函数的概念,同时帮你区分两种回调:同步和异步。
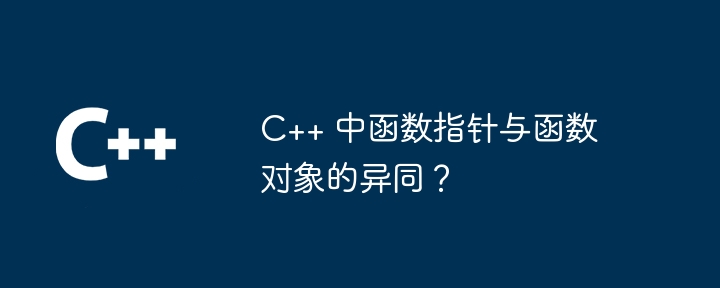
函数指针和函数对象都是处理函数作为数据的机制。函数指针是指向函数的指针,而函数对象是包含重载的operator()的对象。两者都可以捕获变量并创建闭包。不同之处在于,函数指针是原始类型,而函数对象是类;函数指针必须指向有效函数,否则会产生未定义行为,而函数对象可以脱离其创建的函数而存在;函数对象通常比函数指针更易用。实战场景中,可以在排序算法中使用它们指定排序规则。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
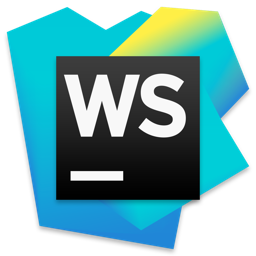
WebStorm Mac version
Useful JavaScript development tools
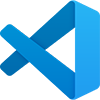
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
