To debug functions using PHP's built-in logging functionality: Use the error_log() function to log messages to a specific destination. Other logging functions are available: trigger_error() throws a custom error, syslog() logs to the system log, and logger() provides advanced control. Creating a custom logging class provides greater flexibility, encapsulating logging operations and setting specific destinations.
How to debug PHP functions through logging
In PHP development, logging errors and debugging information is crucial, especially When working with complex functions or solving problems. This article will introduce how to use PHP's built-in logging function to debug functions, and demonstrate its practicality through practical cases.
Use the error_log() function
The error_log() function is the most basic logging function in PHP. It logs messages to a specific destination, such as the PHP error log or a custom file. The syntax is as follows:
error_log(string $message, int $message_type = 0, string $destination = null, string $extra_headers = null);
Practical example: Logging function errors
// 定义一个可能会抛出错误的函数 function divide($a, $b) { if ($b == 0) { error_log("Error: Divide by zero", 0); return false; } return $a / $b; } // 调用函数并记录任何错误 $result = divide(10, 0); if ($result === false) { echo "Error occurred during division"; }
Running this code will log a message in the PHP error log indicating a divide-by-0 error.
Other logging functions available
In addition to error_log(), there are other PHP functions available for broader logging functions:
- trigger_error(): Throws a custom error, which can be recorded in the log.
- syslog(): Log messages to the system log.
- logger(): Provides more advanced logging control, such as setting level and format.
Using custom logging classes
Creating custom logging classes can further increase the flexibility of logging. This class can encapsulate logging operations and provide application-specific log formats and destinations.
class Logger { private $destination; public function __construct($destination) { $this->destination = $destination; } public function log($message, $level = 'info') { error_log("$level: $message", 0, $this->destination); } } // 实例化 Logger 类并使用它进行日志记录 $logger = new Logger('my_log.txt'); $logger->log('Custom log message');
Debugging PHP functions through logging can greatly simplify the debugging process, improve development efficiency and help solve problems quickly.
The above is the detailed content of How to debug PHP functions through logging?. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
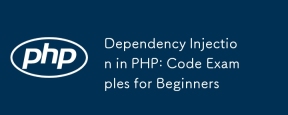
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
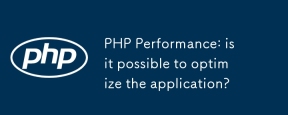
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
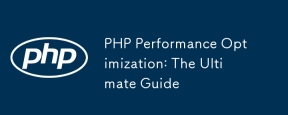
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
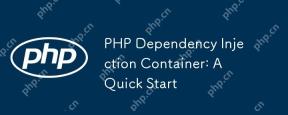
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
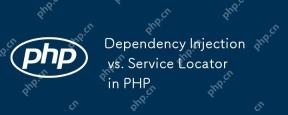
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
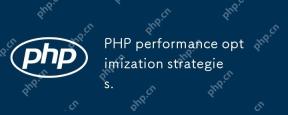
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
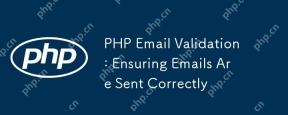
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
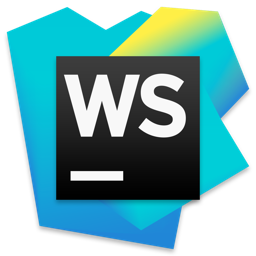
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
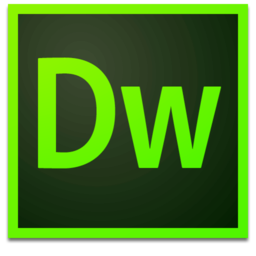
Dreamweaver Mac version
Visual web development tools
