


The choice of data structure is crucial to Go function performance. Each structure has advantages and disadvantages: Array: fast indexing, does not support different types of elements. Slice: Dynamic size, supports multiple values of the same type. Linked list: low memory overhead, high insertion/deletion efficiency, low random access efficiency. Stack: Follow the LIFO principle and operate efficiently. Queue: Follows the FIFO principle and is thread-safe. Dictionary (Map): fast search, supports multiple types, has overhead when resizing.
Go function performance optimization data structure selection guide
In Go programming, choosing the appropriate data structure is crucial. It can significantly affect function performance. Each data structure has its advantages and disadvantages, and the specific choice needs to be based on specific scenarios and needs.
Array
Advantages:
- Fast indexing and traversal
- Fixed size, memory Allocation has no overhead
- Supports different element types
Disadvantages:
- Incurs overhead when reallocating the array
Slicing
Advantages:
- The bottom layer is an array, providing similar indexing and traversal performance
- Dynamic resizing without reallocation
- Can encapsulate multiple values of the same type
Disadvantages:
- Does not support different element types
Linked list
Advantages:
- Low memory allocation overhead , suitable for storing large amounts of data
- Can insert and delete elements independently
- High efficiency in sequential traversal
Disadvantages:
- Random access and update efficiency is low
- Cannot be indexed directly
Stack
Advantages:
- Follow the first-in-last-out (LIFO) principle, insertion and removal are efficient
- Useful in application state management and recursive calls
Disadvantages:
- Unable to directly access intermediate elements
- May cause overflow when the stack is full
Queue
Advantages:
- Follow the first-in-first-out (FIFO) principle, insertion and removal are efficient
- Thread-safe
- In Useful in pipeline communication and buffer processing
Disadvantages:
- Unable to directly access intermediate elements
- May cause when the queue is full Blocking
Dictionary (Map)
Advantages:
- Quickly find and retrieve values based on keys
- Supports multiple data types as keys and values
- Automatically resizes when rehashing
Disadvantages:
- The traversal efficiency is lower than that of arrays or slices
- The data types of keys and values are restricted
Practical case:
Suppose we have a function that calculates the average of a set of integers.
// 使用数组 func AvgArray(arr []int) float64 { var sum int for _, v := range arr { sum += v } return float64(sum) / float64(len(arr)) } // 使用切片 func AvgSlice(slice []int) float64 { var sum int for i := 0; i < len(slice); i++ { sum += slice[i] } return float64(sum) / float64(len(slice)) } // 使用链表 type Node struct { Value int Next *Node } func AvgLinkedList(head *Node) float64 { if head == nil { return 0 } var sum int var count int for node := head; node != nil; node = node.Next { sum += node.Value count++ } return float64(sum) / float64(count) }
Through benchmark test comparison, for small data sets, the performance of arrays and slices is similar; for large data sets, the performance of slices and linked lists is better than arrays; for data sets with frequent insertion and deletion operations, linked lists have the best performance . Therefore, it is crucial to choose the right data structure based on your specific needs.
The above is the detailed content of Golang function performance optimization data structure selection guide. For more information, please follow other related articles on the PHP Chinese website!
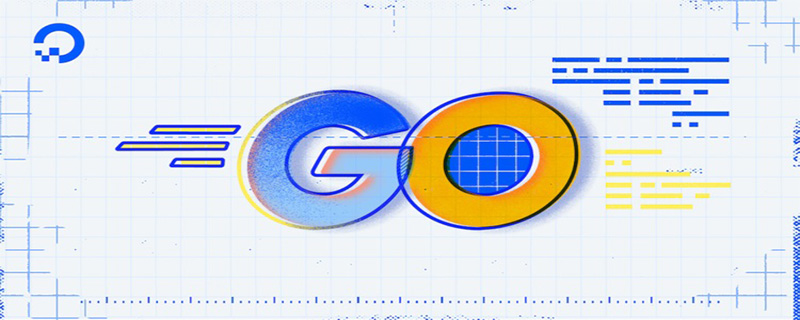
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
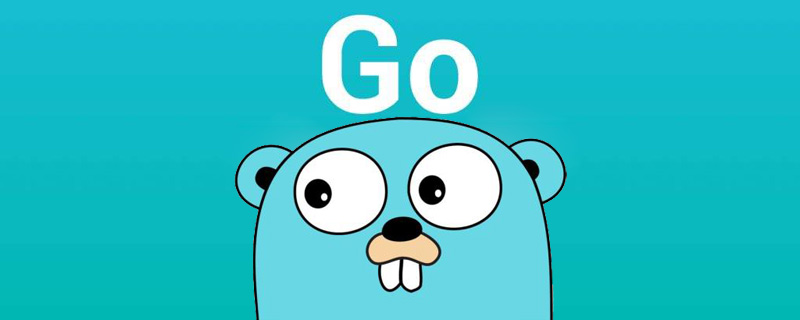
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
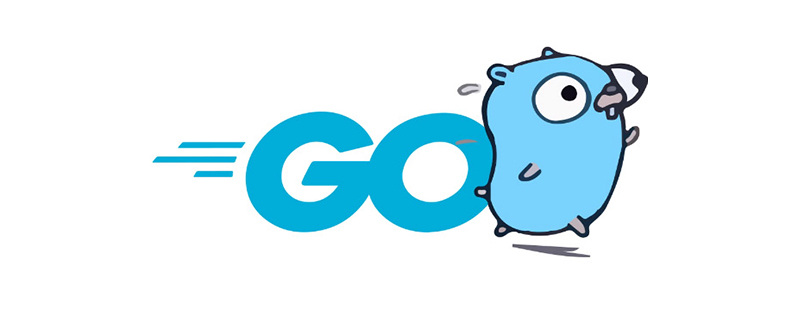
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
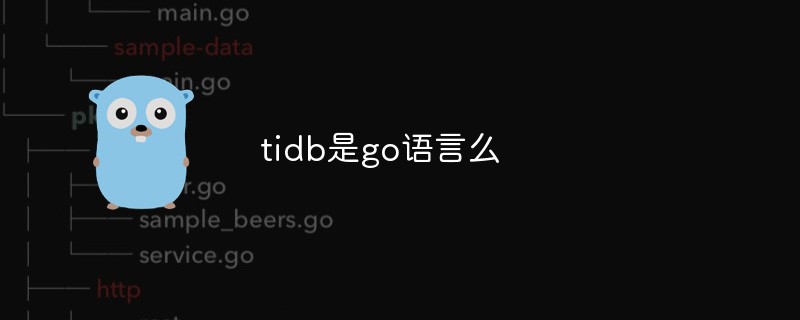
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
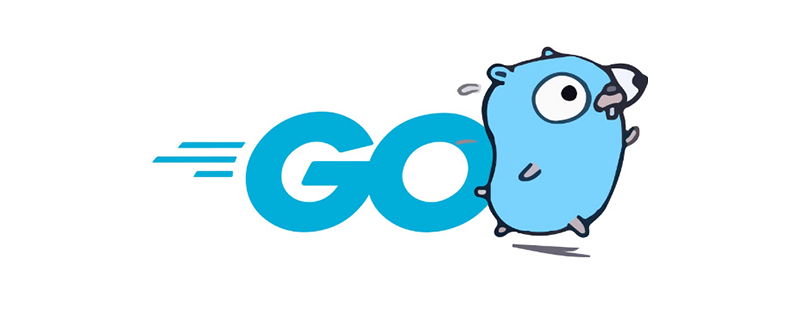
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
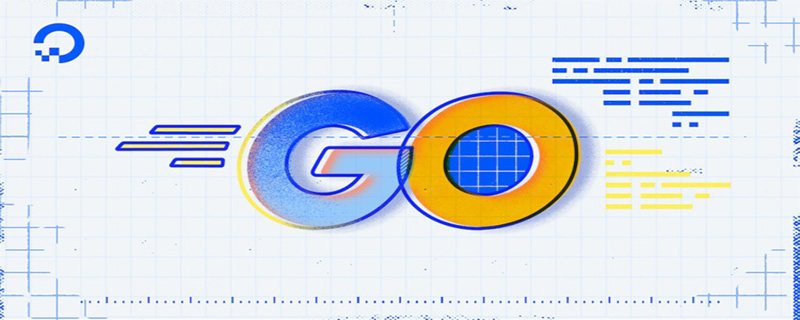
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
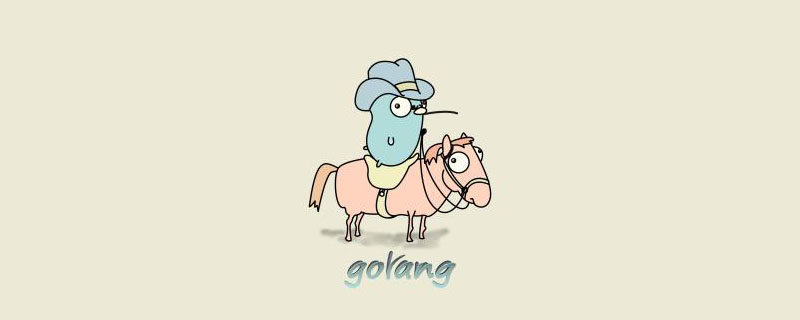
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
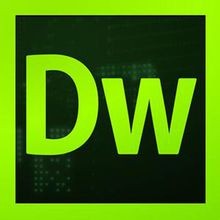
Dreamweaver CS6
Visual web development tools
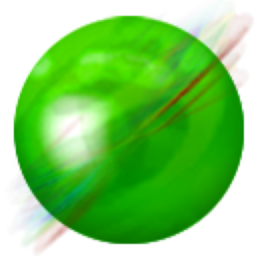
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
