How to encapsulate exceptions in C++ function exception handling?
C Exception encapsulation enhances the readability and maintainability of the code and can separate error information from processing logic. Error information can be encapsulated by defining an exception class that inherits from std::exception. Use throw to throw exceptions and try-catch to catch exceptions. In the actual case, the function that reads the file uses the exception class to encapsulate the error of failure to open the file. When calling this function, the exception can be caught and the error message can be printed.
Exception encapsulation in C function exception handling
In C functions, exception encapsulation can improve the readability and Maintainability. By encapsulating exceptions, you can separate error information from processing logic, creating clearer, easier-to-understand code.
Definition of exception class
First, we need to define an exception class to encapsulate error information. This class should inherit from the standard library exception class std::exception
. For example:
class MyException : public std::exception { public: MyException(const std::string& message) : std::exception(message) {} };
This exception class defines a constructor that accepts a string parameter as the exception message.
Exception throwing in a function
Throwing an exception in a function is simple. You can use the throw
keyword followed by the exception object:
void myFunction() { if (someCondition) { throw MyException("发生了一些错误!"); } }
Exception catching in the function
To catch exceptions, you can use try
and catch
blocks:
int main() { try { myFunction(); } catch (MyException& e) { std::cout << "错误:" << e.what() << std::endl; } }
Practical example
Consider a function that reads a file and counts the total number of lines in the file:
int countLines(const std::string& filepath) { std::ifstream ifs(filepath); if (!ifs.is_open()) { throw MyException("无法打开文件!"); } int count = 0; std::string line; while (std::getline(ifs, line)) { ++count; } return count; }
In this function, we use the MyException
class to encapsulate the error message of file opening failure. When calling this function, we can catch the exception and print the error message:
int main() { try { int lineCount = countLines("inputFile.txt"); std::cout << "文件共 " << lineCount << " 行" << std::endl; } catch (MyException& e) { std::cout << "错误:" << e.what() << std::endl; } }
The above is the detailed content of How to encapsulate exceptions in C++ function exception handling?. For more information, please follow other related articles on the PHP Chinese website!
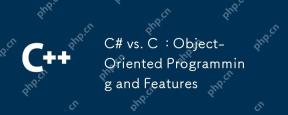
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
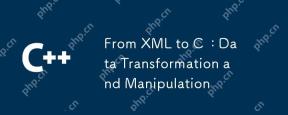
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
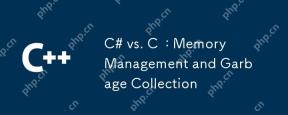
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
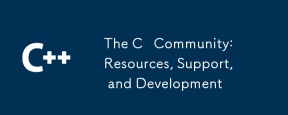
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
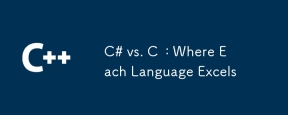
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
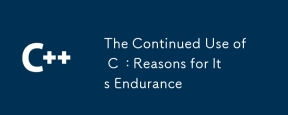
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
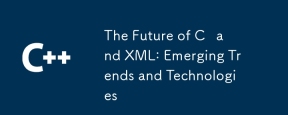
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
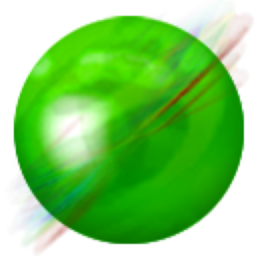
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
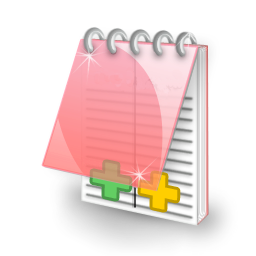
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
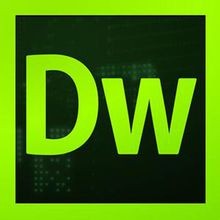
Dreamweaver CS6
Visual web development tools