Security considerations and best practices for PHP functions
Security considerations for PHP functions include input validation, escaping output, authorization and authentication, function overrides, and disabling dangerous functions. Best practices include using parameter type checking, safe string functions, input/output filters, the principle of least privilege, and conducting security audits.
Security considerations and best practices for PHP functions
Functions in PHP provide powerful functionality, but if not Consider their safety carefully, they can pose serious risks. This article explores security considerations for PHP functions and provides best practices to help you write safer, more robust code.
Security Considerations
-
Input Validation: Ensure that function inputs are properly validated to prevent malicious input from corrupting the application. Validate input using built-in functions such as
filter_input()
or custom regular expressions. -
Escape output: Be sure to escape potentially dangerous characters before outputting user-supplied input to HTML or other environments. Use built-in functions such as
htmlspecialchars()
to escape output. - Authorization and Authentication: Restrict access to sensitive functions, allowing only authorized users to execute them. Implement appropriate user authorization and authentication mechanisms to ensure that only authorized users have access to protected functions.
- Function Override: Prevent malicious users from executing malicious code by overwriting core PHP functions. Include autoloaders in your code to avoid overriding core functions.
-
Disable dangerous functions: Disable dangerous functions that may pose a security risk. Use the
ini_set()
function or override the configuration inphp.ini
to disable unnecessary functions.
Best Practices
- Use parameter type checking: Declare the types of function parameters and, where possible, Use type hints to force input validation.
-
Use safe string functions: Use functions such as
filter_input()
,preg_replace()
andstr_replace()
etc. Safe string functions to validate and process input. - Implement input/output filters: Create custom filters or use third-party libraries to further validate input and escape output.
- Follow the principle of least privilege: Grant a function only the minimum permissions required to access its execution. Restrict access to sensitive data to mitigate the risk of data breaches.
- Conduct security audits: Conduct regular security audits of the code to identify and fix potential vulnerabilities.
Practical case
Let us consider a function that handles user input:
function processUserInput($input) { return $input; }
In order to improve its security , we can apply the following best practices:
##Input validation: Use regular expressions to verify that the input contains only letters and numbers:
if (!preg_match('/^[a-zA-Z0-9]+$/', $input)) { throw new InvalidArgumentException("Invalid input"); }
Escape output: Escape output before outputting to HTML:
return htmlspecialchars($input);
The above is the detailed content of Security considerations and best practices for PHP functions. For more information, please follow other related articles on the PHP Chinese website!
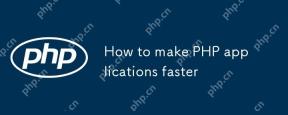
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
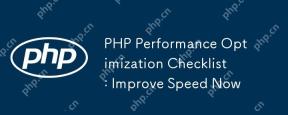
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
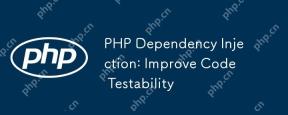
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
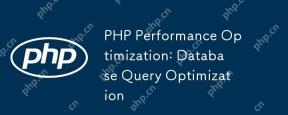
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
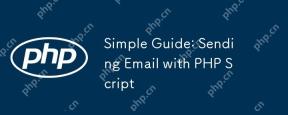
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
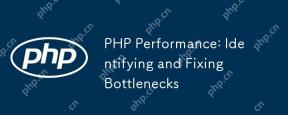
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
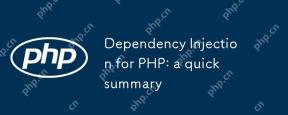
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
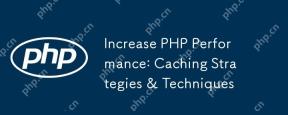
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
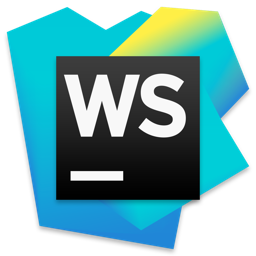
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
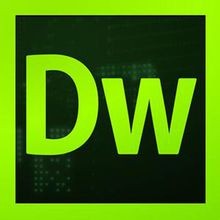
Dreamweaver CS6
Visual web development tools
