What are the best practices for exception handling in Java?
Java exception handling best practices include using specific exception types to handle errors accurately. Catch only the exceptions you need to avoid cluttering your code. Provide meaningful error messages that are easy to understand. Use finally blocks appropriately to ensure resources are released.
Java Exception Handling Best Practices
Exception handling is an essential part of Java programming, which allows applications to Handle errors gracefully and continue execution when errors occur. When handling exceptions, there are several best practices to follow to write robust and maintainable code:
Use specific exception types
Use as specific as possible Exception type. This will allow you to handle errors more precisely and avoid unnecessary branches in your code. For example, use FileNotFoundException
instead of IOException
.
Catch only the exceptions you need
Catch only the exceptions you need to handle. Catching irrelevant exceptions can make your code difficult to maintain. You can use multiple catch blocks to catch different exception types.
Provide meaningful error messages
Exception messages should provide developers and end users with clear information about the error. Avoid using generic messages such as "An error occurred."
Use the finally block appropriately
#finally
The finally block is always executed after the exception handling block, regardless of whether an exception is thrown. This is useful for freeing resources (such as closing a file processing stream).
Practical Case
The following code demonstrates best practices for proper exception handling:
import java.io.File; import java.io.FileNotFoundException; import java.io.IOException; public class ExceptionHandling { public static void main(String[] args) { try { File file = new File("nonexistent.txt"); if (!file.exists()) { throw new FileNotFoundException("File not found."); } // ... 其他代码 ... } catch (FileNotFoundException e) { System.out.println("Error: " + e.getMessage()); } catch (IOException e) { System.out.println("Unexpected IO error: " + e.getMessage()); } finally { // 释放资源 } } }
In the above example:
- We use a specific exception type
FileNotFoundException
to handle situations where the file does not exist. - We only catch
FileNotFoundException
when we need to handle it. - We provide meaningful information in the exception message.
- We release the resource in the
finally
block.
The above is the detailed content of What are the best practices for exception handling in Java?. For more information, please follow other related articles on the PHP Chinese website!
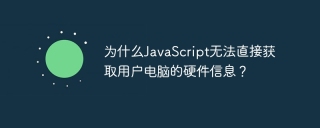
Discussion on the reasons why JavaScript cannot obtain user computer hardware information In daily programming, many developers will be curious about why JavaScript cannot be directly obtained...
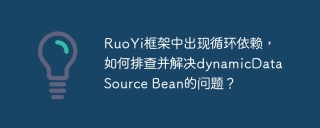
RuoYi framework circular dependency problem troubleshooting and solving the problem of circular dependency when using RuoYi framework for development, we often encounter circular dependency problems, which often leads to the program...

About SpringCloudAlibaba microservices modular development using SpringCloud...
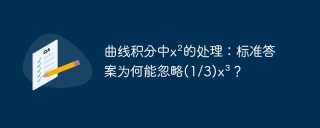
Questions about a curve integral This article will answer a curve integral question. The questioner had a question about the standard answer to a sample question...
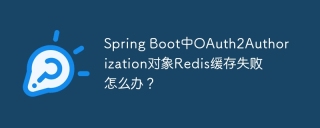
In SpringBoot, use Redis to cache OAuth2Authorization object. In SpringBoot application, use SpringSecurityOAuth2AuthorizationServer...
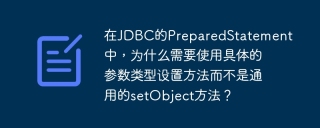
JDBC...
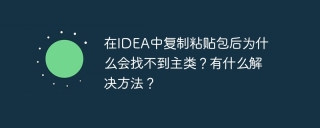
Why can't the main class be found after copying and pasting the package in IDEA? Using IntelliJIDEA...
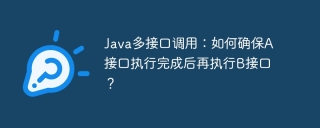
State synchronization between Java multi-interface calls: How to ensure that interface A is called after it is executed? In Java development, you often encounter multiple calls...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
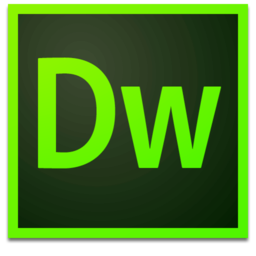
Dreamweaver Mac version
Visual web development tools
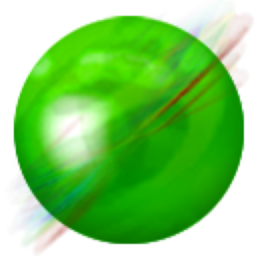
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
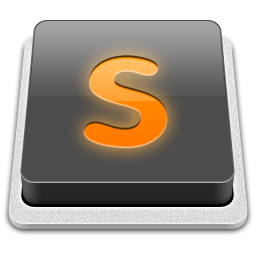
SublimeText3 Mac version
God-level code editing software (SublimeText3)