


GoLang has excellent concurrency, high performance and ease of use, and is suitable for the following scenarios: Concurrency: suitable for processing a large number of parallel tasks, such as web servers, microservices and distributed systems. High performance: The compilation mode generates efficient machine code, and the garbage collection mechanism reduces the memory management burden, making it suitable for applications with high performance requirements. Ease of use: The syntax is clear, the standard library is powerful and easy to use, and the built-in development tools support unit testing, code formatting and document generation.
GoLang Usability Evaluation: Application Scenarios from a Technical Perspective
Introduction
GoLang, also known as Go, is a popular open source programming language praised for its concurrency, high performance, and ease of use. This article will explore the usability of GoLang in various application scenarios and provide an in-depth explanation from a technical perspective.
Concurrency
GoLang is known for its excellent concurrency, implemented through goroutines (lightweight threads). This makes GoLang ideal for handling massively parallel tasks, such as web servers, microservices, and distributed systems.
package main import ( "fmt" "time" ) func main() { go func() { for i := 0; i < 10; i++ { fmt.Println("This is a goroutine") } }() for i := 0; i < 10; i++ { fmt.Println("This is the main thread") } }
High performance
GoLang adopts compiled mode to generate efficient machine code. In addition, its memory management uses a garbage collection mechanism, eliminating the burden of memory management on the programmer. These features make GoLang ideal for performance-critical applications such as high-traffic websites, machine learning algorithms, and financial modeling.
package main import ( "fmt" "math/big" ) func main() { num1 := big.NewInt(1000000) num2 := big.NewInt(1000000) sum := num1.Mul(num1, num2) fmt.Println(sum) }
Ease of use
GoLang philosophy emphasizes simplicity and readability. The language syntax is clear and the standard library is powerful and easy to use. In addition, GoLang has built-in support for development tools such as unit testing, code formatting, and documentation generation.
package main import ( "fmt" "testing" ) func add(a, b int) int { return a + b } func TestAdd(t *testing.T) { tests := []struct { a, b int want int }{ {1, 2, 3}, {5, 10, 15}, } for _, test := range tests { got := add(test.a, test.b) if got != test.want { t.Errorf("add(%d, %d) = %d, want %d", test.a, test.b, got, test.want) } } }
Practical case
- Kubernetes: Kubernetes is a popular container orchestration system written in GoLang, taking advantage of its concurrency and high-performance features to efficiently manage and schedule containers.
- Docker: Docker is a container management platform, also written in GoLang, that leverages its lightweight threads to handle containerized applications.
- Netflix: Netflix uses GoLang to build its media streaming service, which needs to handle a large number of concurrent requests and high throughput.
Conclusion
GoLang is a language well suited for a variety of application scenarios, including applications with high requirements for concurrency, high performance, and ease of use. . Its powerful features and wide range of practical use cases make it a popular choice in modern software development.
The above is the detailed content of Golang usability evaluation: interpreting its application scope from a technical perspective. For more information, please follow other related articles on the PHP Chinese website!
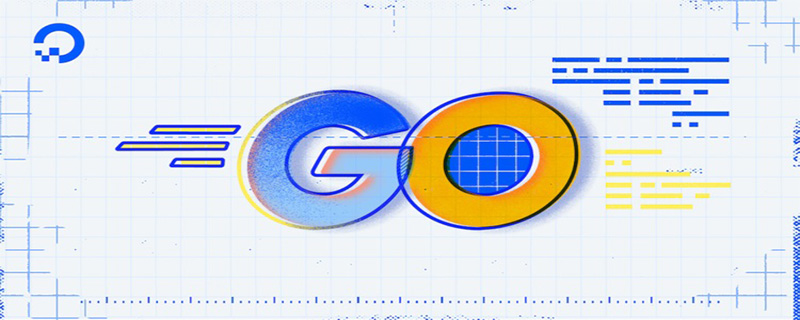
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
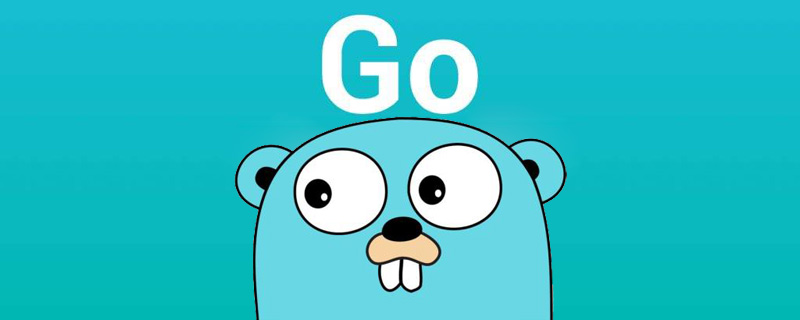
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
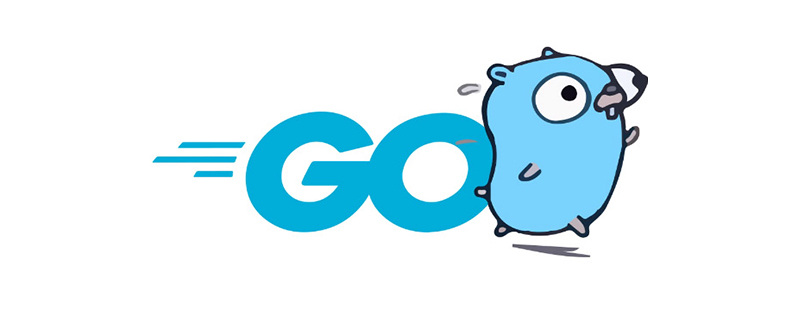
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
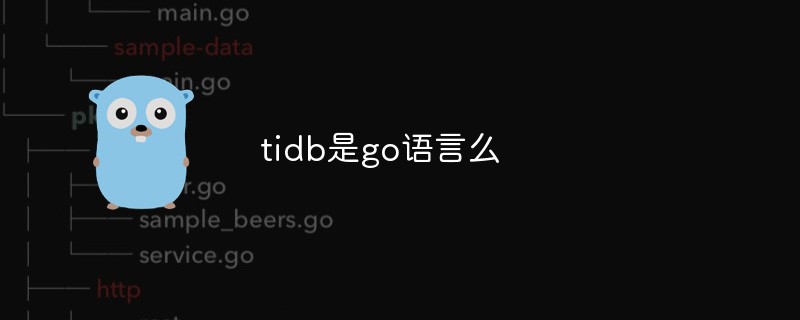
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
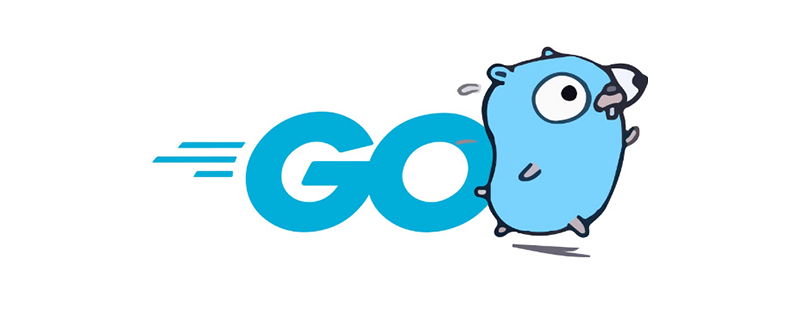
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
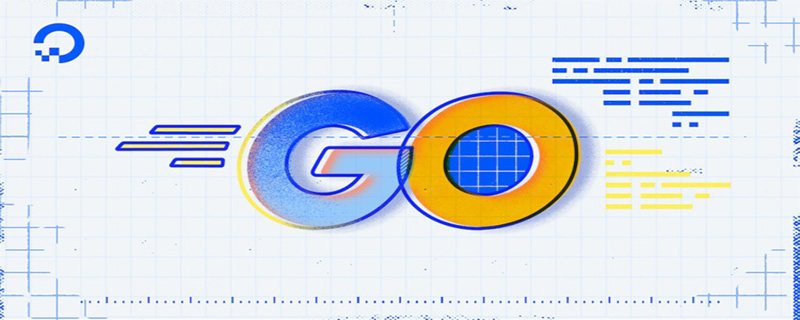
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
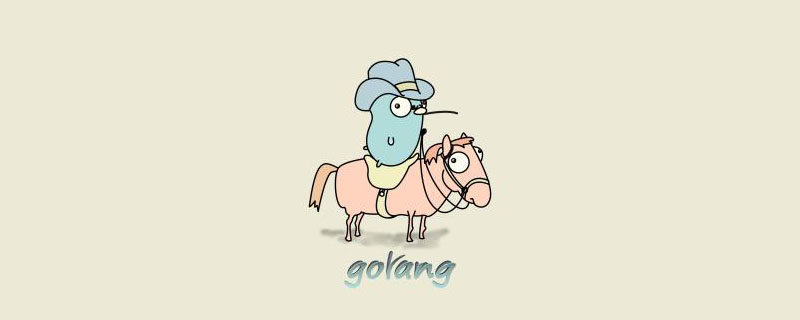
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
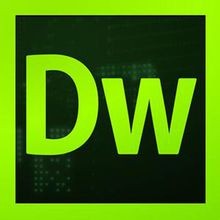
Dreamweaver CS6
Visual web development tools
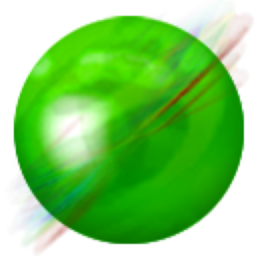
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
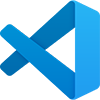
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
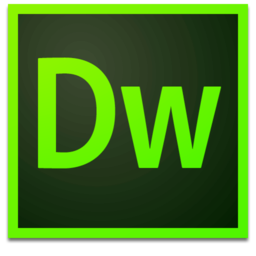
Dreamweaver Mac version
Visual web development tools
