A must-have for veterans: Tips and precautions for * and & in C language
In C language, it represents a pointer, which stores the address of other variables; & represents the address operator, which returns the memory address of the variable. Tips for using pointers include defining pointers, dereferencing pointers, and ensuring that pointers point to valid addresses; tips for using address operators & include obtaining variable addresses, and returning the address of the first element of the array when obtaining the address of an array element. A practical example demonstrating the use of pointer and address operators to reverse a string.
* and & in C language: Tips and precautions
Introduction
Pointer () and address operator (&) are powerful tools in C language that can manipulate memory addresses and data. Understanding its usage is crucial, especially for experienced developers. This article will delve into the techniques and precautions of and &, and provide practical cases to illustrate their usage.
Pointer (*)
- Pointer is a variable that stores the address of other variables.
- Use the * operator to define a pointer:
int *ptr = &var;
(store the address of var in the pointer ptr) - Use the * operator to dereference the pointer:
*ptr
(Access the value stored in ptr)
Note:
- Make sure the pointer points to valid memory address, otherwise a segfault may occur.
- Before using a dereferenced pointer, make sure it is not NULL.
- Prevent wild pointers (pointers that do not point to valid addresses).
Address operator (&)
- The address operator returns the memory address of a variable.
- Use the & operator to get the address of a variable:
int *ptr = &var;
- & operator can be used to initialize a pointer.
Note:
- Only the address of an addressable object (such as a variable) can be obtained.
- When obtaining the address of an array element, the & operator returns the address of the first element of the array, not the address of the actual element.
Practical case
Reversal of string
#include <stdio.h> #include <string.h> void reverse_string(char *str) { int len = strlen(str); int i; for (i = 0; i < len / 2; i++) { char temp = str[i]; str[i] = str[len - i - 1]; str[len - i - 1] = temp; } } int main() { char str[] = "Hello world"; reverse_string(str); printf("%s", str); // 输出:dlrow olleH return 0; }
Conclusion
Mastering the usage of * and & in C language is crucial for advanced programming. By understanding these tips and considerations, developers can effectively manipulate memory addresses and data, improving the efficiency and security of their code.
The above is the detailed content of A must-have for veterans: Tips and precautions for * and & in C language. For more information, please follow other related articles on the PHP Chinese website!
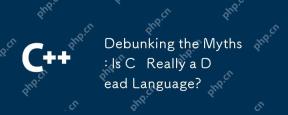
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
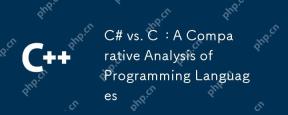
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
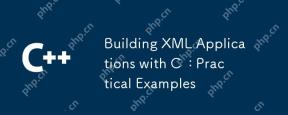
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
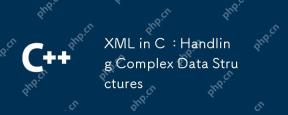
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
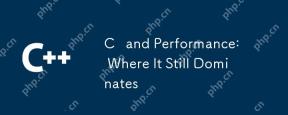
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
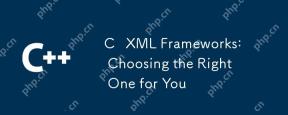
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
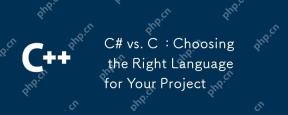
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
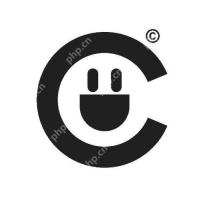
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
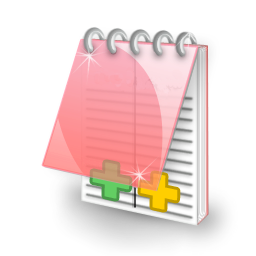
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
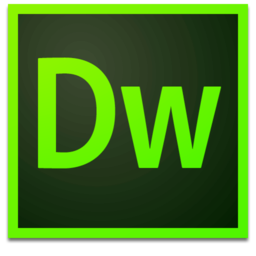
Dreamweaver Mac version
Visual web development tools
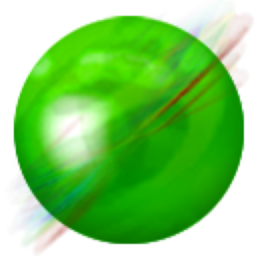
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
