


Golang process control: a deeper understanding of the concurrency model
The concurrency model in Go is based on lightweight thread Goroutine, created with the go keyword and scheduled by the runtime. Channels are used for inter-goroutine communication, while WaitGroup and mutex locks are used to coordinate concurrent execution. Practical examples include concurrent web servers, where Goroutines are used to process requests in parallel, thereby increasing performance and throughput.
Golang Process Control: Deep Understanding of the Concurrency Model
Introduction
In Go, understanding the concurrency model is important Writing efficient and robust programs is crucial. Concurrency enables multiple concurrent tasks to be executed simultaneously, improving performance and throughput. This article takes a deep dive into the concurrency model in Go and provides practical examples to help you grasp this powerful concept.
Concurrency Basics
Concurrency in Go is based on Goroutine, which is a lightweight thread. Unlike operating system threads, Goroutines are coroutines that use shared memory and scheduling mechanisms in the Go runtime. This makes Goroutine very lightweight and has very low overhead.
Goroutine and Channel
Create Goroutine through the go
keyword. Unlike threads in other languages, Goroutines in Go are automatically scheduled by the runtime.
go func() { // Goroutine 代码 }
Channels are used to securely communicate between Goroutines. They allow a Goroutine to send values to a channel, and other Goroutines to receive those values from the channel.
ch := make(chan int) go func() { ch <- 42 } x := <-ch // 从通道接收值
WaitGroup and Mutex
Goroutines are easy to create, but coordinating their concurrent execution is crucial. sync.WaitGroup
can be used to wait for a Goroutine group to complete, while sync.Mutex
can be used to protect concurrent access to shared resources.
var wg sync.WaitGroup func main() { for i := 0; i < 10; i++ { wg.Add(1) go func(i int) { // 使用 i 的 Goroutine wg.Done() // 信号 Goroutine 完成 }(i) } wg.Wait() // 等待所有 Goroutine 完成 }
Practical case: Concurrent network server
The following is an example of writing a concurrent network server using Go:
package main import ( "log" "net/http" ) func main() { // 创建一个 HTTP 服务器 http.HandleFunc("/", handler) // 绑定服务器到端口 err := http.ListenAndServe(":8080", nil) if err != nil { log.Fatal(err) } } func handler(w http.ResponseWriter, r *http.Request) { // 这是一个并发 Goroutine go func() { // 执行一些任务 }() // 主 Goroutine 继续处理请求 }
Conclusion
By leveraging Goroutines, channels, sync.WaitGroup
, and sync.Mutex
, you can build robust, high-concurrency Go applications. Understanding the nuances of concurrency models is key to writing efficient, scalable, and responsive code. With exercises and examples, you can master concurrent programming and improve the performance and quality of your Go applications.
The above is the detailed content of Golang process control: a deeper understanding of the concurrency model. For more information, please follow other related articles on the PHP Chinese website!
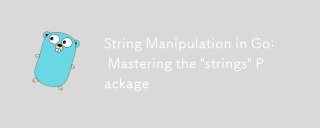
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
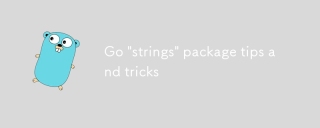
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
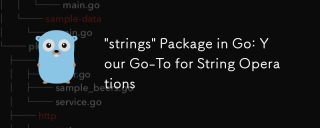
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
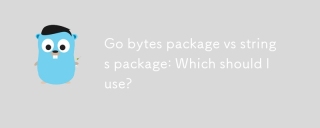
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
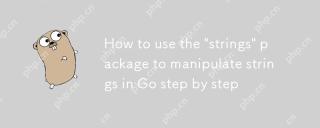
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
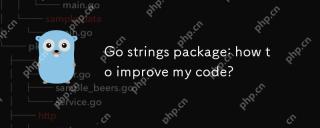
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
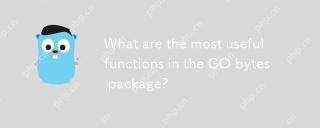
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
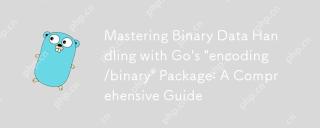
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
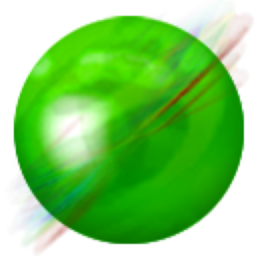
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
