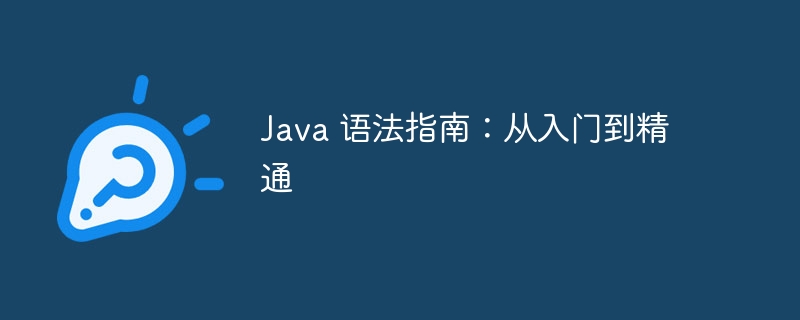
Java is a widely used programming language and is widely used in all walks of life. However, for beginners, the process of learning the Java language may be difficult. PHP editor Xinyi has brought a Java syntax guide, from entry to proficiency, allowing beginners to easily master the core knowledge of the Java language and helping everyone better apply the Java language for development. This guide will provide readers with a comprehensive introduction to the Java language, basic knowledge, common syntax, object-oriented programming, etc., helping everyone quickly become a professional developer of the Java language.
-
Data types: Java provides a rich set of basic data types (such as int, double and boolean) and reference types (such as objects and arrays).
-
Variables: You use variables to store data. They are identified by type and name, for example:
int age = 25;
-
Operators: Java provides various operators for performing arithmetic, comparison and logical operations.
-
Control flow: Use if-else, switch and for loops to control program execution flow.
Objects and classes
-
Class: Objects in Java are instances that encapsulate data. Classes are templates for objects that define their state and behavior.
-
Object: An object is an instance of a class that contains data stored according to the class definition.
-
Inheritance: Subclasses can inherit the properties and methods of the parent class, thereby promoting code reuse.
-
Interface: The interface defines a set of methods that a class must implement to implement the interface.
Arrays and Collections
-
Array: An array is an ordered collection that stores elements of the same type.
-
Collections: Collections are dynamically sized containers used to store objects. Java provides various collection classes such as List, Set, and Map.
Generic
-
Generics: Generics allow you to create classes and methods that operate on different types of objects.
-
Type parameters: Use type parameters in generic declarations to represent data types, for example:
List<String>
.
Exception handling
-
Exception: Exception is an error or abnormal situation that occurs during program execution.
-
try-catch: Use try-catch block to handle exceptions.
-
Throwing exceptions: Use the throw keyword to throw an exception to pass control to the calling method.
Concurrent programming
-
Threads: Threads are independent execution paths that are executed simultaneously in the program.
-
Synchronization: The synchronization mechanism ensures that threads access shared resources in a controlled manner.
-
Concurrent Collections: Concurrent collections are high-performance collections specifically designed for use in multi-threaded environments.
Advanced Features
-
Reflection: ReflectionAllows you to inspect and modify the structure and behavior of a class at runtime.
-
Annotations: Annotations add metadata information to classes, methods and fields.
-
Lambda expressions: Lambda expressions are nameless functions that allow you to express blocks of code succinctly.
Best Practices
- Follow Java coding conventions to improve code readability and maintainability.
- Use clear and meaningful variable names and method names.
- Use comments appropriately to record code.
- Write unit tests to verify the correctness of the code.
- Leverage IDE features such as auto-completion and refactoring to increase productivity.
The above is the detailed content of Java Syntax Guide: From Beginner to Mastery. For more information, please follow other related articles on the PHP Chinese website!