How to set, get and delete cookies in javascript_javascript skills
The example in this article describes the method of setting, obtaining and deleting cookies in javascript. Share it with everyone for your reference. The specific implementation method is as follows:
/* *设置Cookie * * name:cookie所对应的键 * value:cookie所对应的值 * expires:cookie所对应的有效时间 * path:指定可访问cookie的路径 * domain:指定可访问cookie的主机名 * secure:安全性 */ function setCookie (name,value,expires,path,domain,secure) { //cookie键值对 var str = name + "=" + escape(value); //设置cookie的有效期,以小时为单位 if(expires > 0){ var date = new Date(); var ms = expires * 3600 * 1000; date.setTime(date.getTime() + ms); str += "; expires=" + date.toGMTString(); } if(path){ str += "; path=" + path; } if(domain){ str += "; domain=" + domain; } if(secure){ str += "; secure"; } document.cookie = str; } /* *获得Cookie * *cookie_name:cookie的键 */ function getCookie(cookie_name) { var value = null; var allcookies = document.cookie; var cookie_pos = allcookies.indexOf(cookie_name); // 如果找到了索引,就代表cookie存在, // 反之,就说明不存在。 if (cookie_pos != -1) { // 把cookie_pos放在值的开始,只要给值加1即可。 cookie_pos += cookie_name.length + 1; var cookie_end = allcookies.indexOf(";", cookie_pos); if (cookie_end == -1) { cookie_end = allcookies.length; } value = unescape(allcookies.substring(cookie_pos, cookie_end)); } return value; } /* *删除Cookie * *cookie_name:cookie的键 */ function delCookie(cookie_name) { var exp = new Date(); exp.setTime(exp.getTime() - 1); var value = getCookie(cookie_name); if(value){ document.cookie= cookie_name + "=" + value + ";expires=" + exp.toGMTString(); } }
I hope this article will be helpful to everyone’s JavaScript programming design.
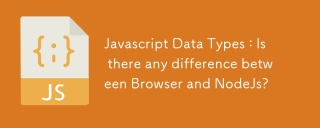
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
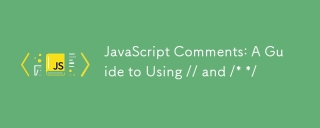
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
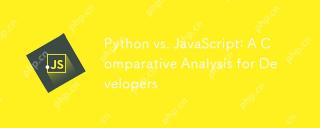
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
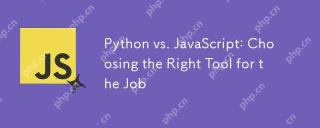
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
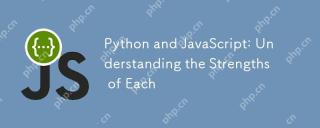
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
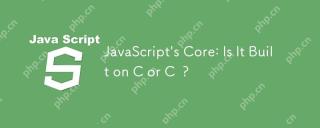
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
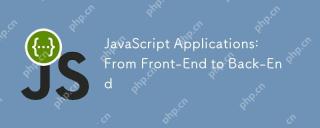
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
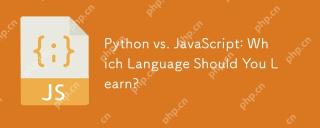
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
