How to use Go language to develop efficient and stable software?
Go language is an open source programming language developed by Google. After years of development, it has become a favorite choice of many software developers. Go language has a rich standard library, efficient concurrency model and fast compilation speed, making it very suitable for developing efficient and stable software. This article will introduce some methods on how to use Go language to develop efficient and stable software, and provide specific code examples.
1. Utilize the concurrency features of Go language
Go language provides a lightweight goroutine concurrency model, which can better utilize the resources of multi-core processors and thereby improve the performance of the software. Through goroutine, a function or method can be executed in a concurrent manner to avoid blocking the main thread and improve the response speed of the program. The following is a simple concurrency example:
package main import ( "fmt" "time" ) func printNumbers() { for i := 0; i < 10; i++ { time.Sleep(1 * time.Second) fmt.Println(i) } } func main() { go printNumbers() time.Sleep(11 * time.Second) }
In the above example, the printNumbers
function will print a number every 1 second, while in the main
function, We use the go
keyword to open a new goroutine to execute the printNumbers
function. In this way, the program will execute the printNumbers
function in the background without blocking the main thread.
2. Use the error handling mechanism of the Go language
The Go language provides a built-in error handling mechanism, through the error
type and panic
, recover
mechanism can effectively manage and handle errors. Good error handling is crucial when writing efficient, stable software. The following is a simple error handling example:
package main import ( "fmt" "errors" ) func divide(x, y int) (int, error) { if y == 0 { return 0, errors.New("division by zero") } return x / y, nil } func main() { result, err := divide(10, 0) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Result:", result) } }
In the above example, the divide
function is used to calculate the division of two numbers and returns an error if the divisor is 0. In the main
function, we obtain the calculation results and errors by calling the divide
function, and handle them accordingly based on the returned errors.
3. Using the testing framework of Go language
Go language provides a rich testing framework, such as testing
package and go test
command, you can Help developers write efficient and stable test cases. Testing is an important means to ensure software quality. By writing comprehensive test cases, potential problems can be discovered and solved early. The following is a simple test example:
package main import "testing" func add(x, y int) int { return x + y } func TestAdd(t *testing.T) { result := add(3, 4) if result != 7 { t.Errorf("Expected 7 but got %d", result) } }
In the above example, we defined a add
function to calculate the sum of two numbers and wrote a test functionTestAdd
, used to test the correctness of the add
function. By running the go test
command, you can execute the test case and output the test results.
Conclusion
Using Go language to develop efficient and stable software requires comprehensive consideration of concurrency models, error handling mechanisms, and testing frameworks. By properly utilizing the features and tools of the Go language, developers can improve the performance, stability, and maintainability of software. We hope that the methods and examples introduced above can help readers use Go language for software development more efficiently.
The above is the detailed content of How to use Go language to develop efficient and stable software?. For more information, please follow other related articles on the PHP Chinese website!
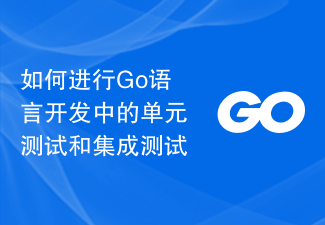
如何进行Go语言开发中的单元测试和集成测试摘要:在软件开发中,单元测试和集成测试是确保代码质量和功能稳定性的重要手段。而在Go语言中,也有一套完善的工具支持,使得单元测试和集成测试变得更加简单和高效。本文将介绍如何进行Go语言开发中的单元测试和集成测试,并通过一些示例代码进行演示。引言Go语言是一种开源的编程语言,因其简洁而强大的特性而受到越来越多开发者的喜
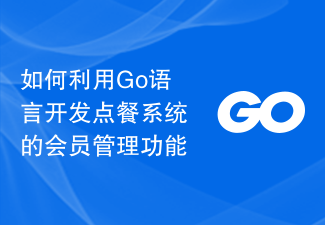
如何利用Go语言开发点餐系统的会员管理功能一、引言随着移动互联网的普及,点餐系统成为了餐饮行业不可或缺的一部分。而会员管理功能作为点餐系统的重要组成部分,对于提升用户体验、增强用户黏性具有重要作用。本文将介绍如何利用Go语言开发点餐系统的会员管理功能,并提供具体的代码示例。二、会员管理功能的需求分析会员注册:用户可以通过手机号、邮箱等方式注册成为会员。会员登
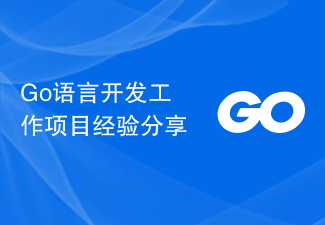
随着互联网的发展,计算机科学领域也迎来了许多全新的编程语言。其中,Go语言以其并发性和简洁的语法,逐渐成为许多开发者的首选。作为一名从事软件开发的工程师,我有幸参与了一个基于Go语言的工作项目,并在这个过程中积累了一些宝贵的经验和教训。首先,选择适合的框架和库是至关重要的。在开始项目之前,我们进行了详细的调研,尝试了不同的框架和库,最终选择了Gin框架作为我
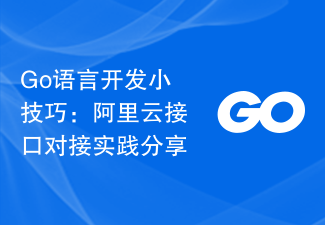
Go语言开发小技巧:阿里云接口对接实践分享前言:现如今,云计算已经成为了企业信息化建设的核心技术之一,而阿里云作为国内知名的云计算服务提供商,拥有丰富的云产品和服务。本文将分享笔者在使用Go语言对接阿里云接口时的一些实践经验,并以代码示例的形式进行阐述。一、引入阿里云GoSDK在使用Go语言对接阿里云接口之前,首先我们需要引入相应的阿里云GoSDK,以便
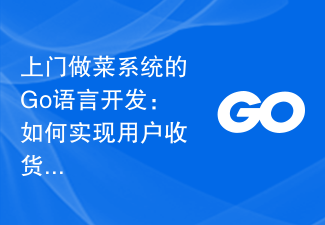
随着人们生活质量的提高,越来越多的家庭开始选择在家享用优质的餐饮服务。而上门做菜系统应运而生,成为了一种便捷、安全、健康的饮食选择方式。在这样的服务下,用户可以在网上下单,由专业厨师上门准备食材、烹饪美食,并送到用户家中享用。Go语言有着高效、稳定、安全等特点,因此配合上门做菜系统进行开发可以得到非常好的效果。本文将介绍如何在上门做菜系统中实现用户收货地址
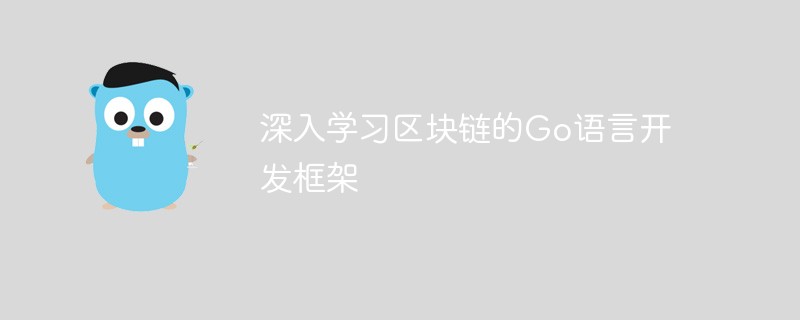
区块链技术的出现,使得数字货币的应用成为可能,也在许多领域得到了广泛应用。随着区块链技术领域的扩大,开发人员对于更好的应用程序编写方式的需求也高涨起来。于是,一个叫做Go语言(简称Golang)的编程语言悄悄兴起,成为了区块链开发人员的最爱。Go语言是谷歌公司开发的一种系统级编程语言,自诞生以来,一直着重强调程序设计的简捷和高效。Go语言的优点包括:静态类型
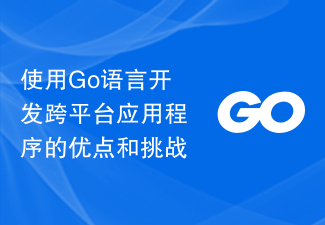
使用Go语言开发跨平台应用程序的优点和挑战随着移动互联网的迅速发展,跨平台应用程序成为了开发者们的必备技能。Go语言作为一门简洁高效、并发性能出色的语言,因其独特的特性而逐渐受到开发者的青睐。本文将探讨使用Go语言开发跨平台应用程序的优点和挑战,并提供相应的代码示例。一、优点1.语言特性齐备:Go语言提供了丰富的标准库,涵盖了各种常用功能,如文件操作、网络通
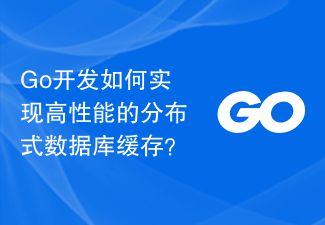
如何在Go语言开发中实现高性能的分布式数据库缓存系统引言:随着互联网的高速发展,数据量和访问量不断增加,对数据库性能的要求也越来越高。分布式数据库缓存系统是一种提高数据库访问性能的解决方案,它将数据库中的数据缓存在内存中,以提供更快速的读写操作。本文将介绍如何利用Go语言开发高性能的分布式数据库缓存系统。选择合适的缓存存储引擎在开发分布式数据库缓存系统时,选


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
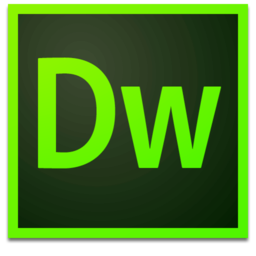
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
