Polymorphism concepts and code examples in PHP
In object-oriented programming, polymorphism is an important concept. It allows different objects to respond differently to the same message. In PHP, polymorphism can be achieved through interfaces and inheritance. Next we will analyze the concept of polymorphism in PHP through specific code examples.
First, we create an interface Shape
, which contains a calculateArea
method:
interface Shape { public function calculateArea(); }
Next, we create two classes Circle
and Square
respectively implement the Shape
interface:
class Circle implements Shape { private $radius; public function __construct($radius) { $this->radius = $radius; } public function calculateArea() { return round(pi() * pow($this->radius, 2), 2); } } class Square implements Shape { private $sideLength; public function __construct($sideLength) { $this->sideLength = $sideLength; } public function calculateArea() { return pow($this->sideLength, 2); } }
Next, we create a function getShapeArea
, which accepts implementation Take the object of the Shape
interface as a parameter and call its calculateArea
method to calculate the area:
function getShapeArea(Shape $shape) { return $shape->calculateArea(); }
Now, we can create Circle
and Square
objects and call the getShapeArea
function to calculate their areas:
$circle = new Circle(5); $square = new Square(4); echo "圆的面积:" . getShapeArea($circle) . ";"; // 输出:圆的面积:78.54; echo "正方形的面积:" . getShapeArea($square) . "。"; // 输出:正方形的面积:16。
In the code example above, Circle
and Square# The ## classes respectively implement the
Shape interface and cover the
calculateArea method to calculate the area based on the specific shape. By calling the
getShapeArea function and passing in different objects, we achieve polymorphism based on different object instances.
The above is the detailed content of Analyzing the concept of polymorphism in PHP. For more information, please follow other related articles on the PHP Chinese website!
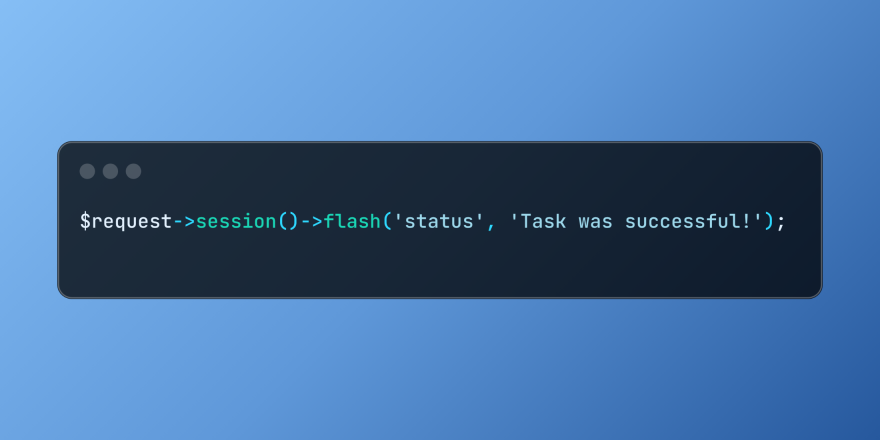
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
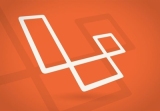
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
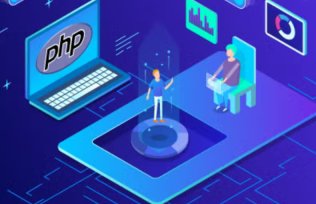
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
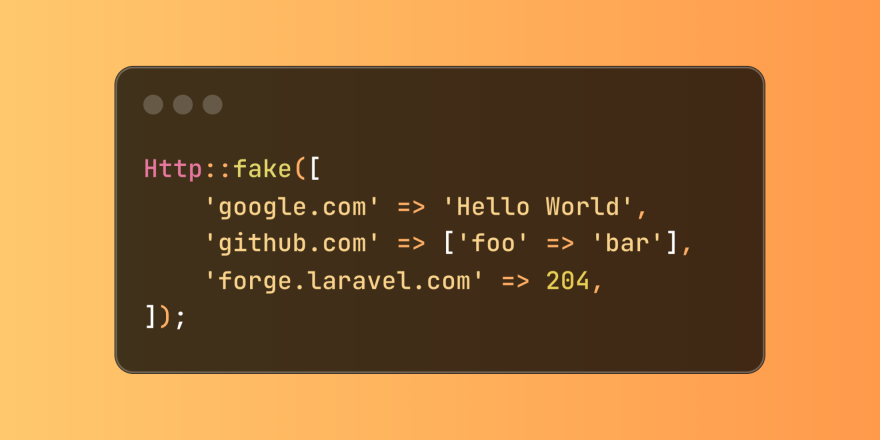
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
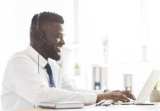
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
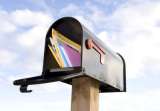
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov
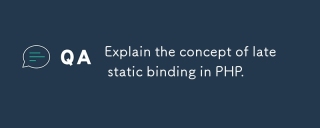
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
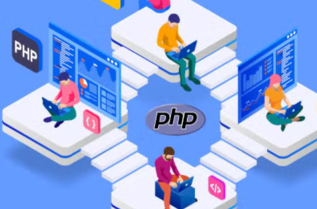
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
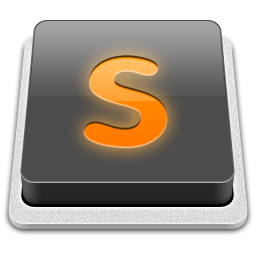
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
