What are the database connection methods in Go language?
As a powerful programming language, Go language has rich database connection methods to facilitate developers to perform database operations in applications. In the Go language, common database connection methods mainly include using native database drivers and using ORM frameworks. These two methods will be introduced in detail below, with specific code examples.
1. Use native database driver
The Go language provides native database connection functions through the database/sql
package in the standard library. Developers can Directly operate the database. The following takes the MySQL database as an example to demonstrate how to use the native database driver to connect to the database:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { // 连接MySQL数据库 db, err := sql.Open("mysql", "username:password@tcp(localhost:3306)/database_name") if err != nil { fmt.Println("数据库连接失败:", err) return } defer db.Close() // 执行SQL查询 rows, err := db.Query("SELECT * FROM users") if err != nil { fmt.Println("查询失败:", err) return } defer rows.Close() // 遍历查询结果 for rows.Next() { var id int var name string err := rows.Scan(&id, &name) if err != nil { fmt.Println("数据解析失败:", err) return } fmt.Printf("ID: %d, Name: %s ", id, name) } }
In the above code, first connect to the MySQL database through the sql.Open
function, and then through db .Query
performs the query operation and parses the query results through the rows.Scan
method.
2. Use ORM framework
In addition to native database drivers, Go language also supports multiple ORM (Object-Relational Mapping) frameworks, such as GORM, XORM, etc. These frameworks can simplify database operations and allow developers to more conveniently perform operations such as additions, deletions, modifications, and searches. The following takes GORM as an example to demonstrate how to use the ORM framework to connect to the database:
First, you need to introduce the GORM library into the Go project:
go get -u gorm.io/gorm go get -u gorm.io/driver/mysql
Then write the following code example:
package main import ( "gorm.io/driver/mysql" "gorm.io/gorm" "fmt" ) type User struct { ID uint Name string } func main() { // 连接MySQL数据库 dsn := "username:password@tcp(localhost:3306)/database_name" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { fmt.Println("数据库连接失败:", err) return } // 自动迁移数据库表结构 db.AutoMigrate(&User{}) // 创建用户 user := User{Name: "Alice"} result := db.Create(&user) if result.Error != nil { fmt.Println("创建用户失败:", result.Error) return } fmt.Println("创建用户成功") }
In the above code, the MySQL database is first connected through the gorm.Open
function, then the database table structure is automatically created through the db.AutoMigrate
method, and finally through the db.Create
Create user data.
Through the above introduction, it can be seen that the Go language has a variety of database connection methods, and developers can choose the appropriate method to perform database operations according to specific needs.
The above is the detailed content of What are the database connection methods in Go language?. For more information, please follow other related articles on the PHP Chinese website!
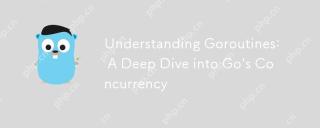
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
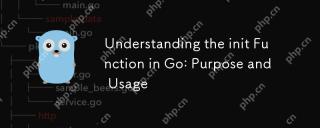
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
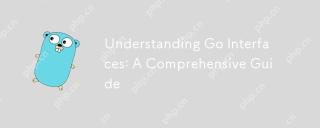
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
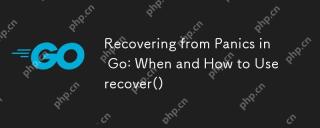
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
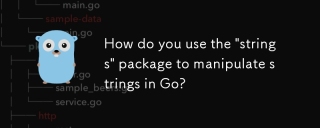
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
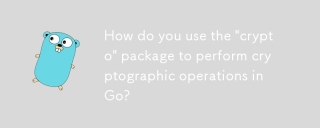
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
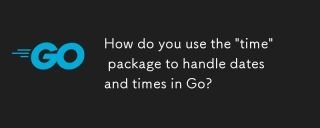
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
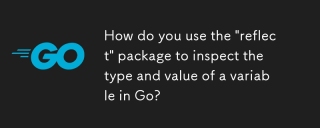
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
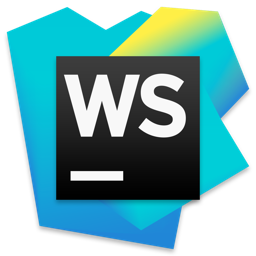
WebStorm Mac version
Useful JavaScript development tools
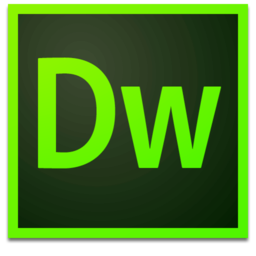
Dreamweaver Mac version
Visual web development tools
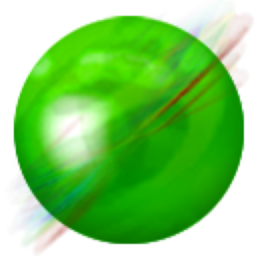
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
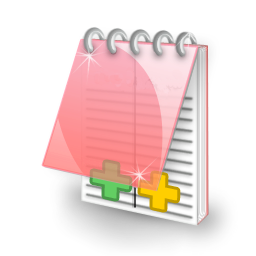
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
