PHP closes MySQL connection
In PHP development, closing the MySQL connection is an important operation, which can effectively release resources and improve system performance. By closing the connection, you can avoid occupying the database connection for a long time, resulting in waste of resources and excessive system load. When writing a PHP program, be sure to close the connection in time when it is no longer needed to connect to the database to optimize system operating efficiency. In this article, we will introduce how to close MySQL connections in PHP to help developers better manage database connection resources.
Close MySQL Connection: Best Practices
Introduction
Closing mysql connections in php is critical to freeing resources, preventing memory leaks, and ensuring application stability. This article explores best practices for closing Mysql connections, including various methods using MySQLi and PDO extensions.
Close the connection using MySQLi
MySQLi is an improved extension in PHP for handling MySQL database. The method to close the MySQLi connection is as follows:
-
mysqli_close()
Function: Close the connection directly. It releases all resources allocated by the connection handle.
$conn = new mysqli("localhost", "username", "passWord", "database"); mysqli_close($conn);
- Destructor: When the MySQLi object is destroyed, the destructor will automatically release the connection. This approach is more concise, but may not work in all situations, such as when you need to close a connection at a specific point in program execution.
$conn = new mysqli("localhost", "username", "password", "database"); // use connection... // Automatically release the connection unset($conn);
Use PDO to close the connection
PDO (PHP Data Object) is the object-orienteddatabaseabstraction layer in PHP. Here's how to close a PDO connection:
-
PDO::close()
Method: Close the connection directly. It releases all resources allocated by the connection object.
$conn = new PDO("mysql:host=localhost;dbname=database", "username", "password"); $conn->close();
- Destructor: Similar to MySQLi, the destructor automatically releases the connection when the PDO object is destroyed.
$conn = new PDO("mysql:host=localhost;dbname=database", "username", "password"); // use connection... // Automatically release the connection unset($conn);
Best Practices
The following are some best practices for closing MySQL connections:
- Always close the connection: You should always close the connection to release resources after you have finished using it.
- Use a try-catch block: Wrap the connection closing operation in a try-catch block to handle any potential exceptions.
- Avoid closing connections in a loop: Closing connections in a loop can reduce application performance. It's better to close the connection outside the loop.
- Use connection pooling: Connection pooling can help reuse connections, thereby improving performance and reducing overhead.
Exit processing
In some cases, you may need to close the connection when the PHP script exits. You can use one of the following methods:
- Register shutdown function: Register a shutdown function to close the connection.
reGISter_shutdown_function(function () { //Close connection... });
- Use finally block: Use finally block to ensure that the connection is always closed when the script exits.
try { // use connection... } finally { //Close connection... }
in conclusion
Closing MySQL connections is an important part of ensuring that your PHP application runs stably and efficiently. By following the best practices outlined in this article, you can free up resources, prevent memory leaks, and provide the best experience for your users.
The above is the detailed content of PHP closes MySQL connection. For more information, please follow other related articles on the PHP Chinese website!
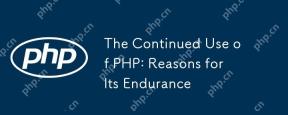
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
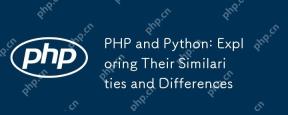
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
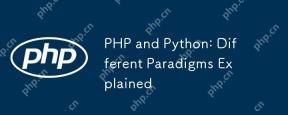
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
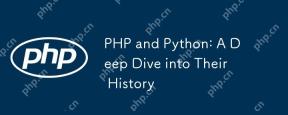
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
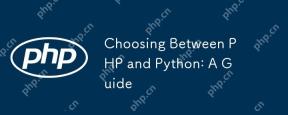
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
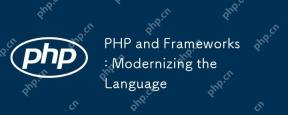
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
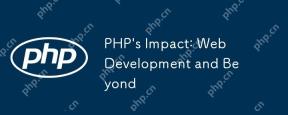
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
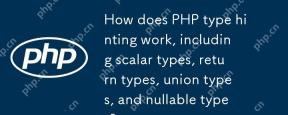
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
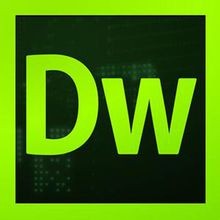
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool