


Learning Golang interface: implementation principles and design patterns
Learning Golang interface: implementation principles and design patterns
In the process of learning the Golang programming language, interface is a very important concept. Interfaces play a very critical role in Golang. They play an important role in achieving polymorphism, decoupling and composition. This article will introduce the implementation principles of Golang interfaces and some common design patterns, and will also give specific code examples to help readers better understand and apply interfaces.
1. Implementation Principle of Golang Interface
In Golang, an interface is an abstract type that defines a set of methods. The implementation principle of interfaces is mainly based on two basic concepts: interface type and interface value.
- Interface type: An interface type is defined by a method set. An interface type can contain zero or more methods. The interface type is defined as follows:
type InterfaceName interface { Method1() returnType1 Method2() returnType2 // 其他方法 }
In the interface type, only the signature of the method needs to be declared without a specific implementation.
- Interface value: The interface value consists of instances of the interface type and the specific implementation type. A type instance that implements all methods in an interface can be assigned to interface values. Interface values can be used to store instances of any type that implement the interface. An example is as follows:
type InterfaceName interface { Method1() returnType1 Method2() returnType2 } type StructName struct{} func (s StructName) Method1() returnType1 { // 方法1的具体实现 } func (s StructName) Method2() returnType2 { // 方法2的具体实现 } var i InterfaceName i = StructName{}
In the above example, the type of variable i
is InterfaceName
, and its value is StructName{}
Instance.
2. Common design patterns
Interfaces are often used to implement design patterns in Golang. The following introduces several common design patterns and their combination with interfaces.
- Strategy pattern: Strategy pattern encapsulates a set of algorithms and makes them interchangeable. The strategy pattern can be implemented through the interface. The example is as follows:
type Strategy interface { DoSomething() } type StrategyA struct{} func (s StrategyA) DoSomething() { // 策略A的具体实现 } type StrategyB struct{} func (s StrategyB) DoSomething() { // 策略B的具体实现 }
- Observer pattern: The observer pattern defines a one-to-many dependency relationship. When an object When the state of an object changes, all objects that depend on it are notified. The observer pattern can be implemented through the interface. The example is as follows:
type Observer interface { Update() } type Subject struct { observers []Observer } func (s Subject) Notify() { for _, observer := range s.observers { observer.Update() } }
3. Specific code example
The following is a simple example to show the specific application of the interface:
// 定义接口 type Shape interface { Area() float64 } // 实现结构体 type Rectangle struct { Width float64 Height float64 } func (r Rectangle) Area() float64 { return r.Width * r.Height } type Circle struct { Radius float64 } func (c Circle) Area() float64 { return 3.14 * c.Radius * c.Radius } func main() { // 创建一个矩形实例 rectangle := Rectangle{Width: 5, Height: 3} // 创建一个圆形实例 circle := Circle{Radius: 2} // 调用接口方法计算面积 shapes := []Shape{rectangle, circle} for _, shape := range shapes { fmt.Println("Area:", shape.Area()) } }
In this example, we define a Shape
interface, containing an Area
method. Then the Rectangle
and Circle
structures were implemented respectively, and the Area
method was implemented. Finally, through the interface Shape
, the areas of different shapes can be calculated.
Through the above examples, readers can better understand the implementation principles of Golang interfaces and the application of design patterns. At the same time, they can also try to write more complex interfaces and implementations themselves to improve their understanding and application capabilities of interface concepts.
The above is the detailed content of Learning Golang interface: implementation principles and design patterns. For more information, please follow other related articles on the PHP Chinese website!
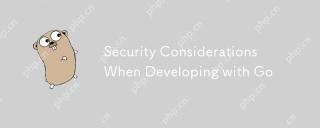
Gooffersrobustfeaturesforsecurecoding,butdevelopersmustimplementsecuritybestpracticeseffectively.1)UseGo'scryptopackageforsecuredatahandling.2)Manageconcurrencywithsynchronizationprimitivestopreventraceconditions.3)SanitizeexternalinputstoavoidSQLinj
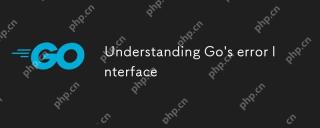
Go's error interface is defined as typeerrorinterface{Error()string}, allowing any type that implements the Error() method to be considered an error. The steps for use are as follows: 1. Basically check and log errors, such as iferr!=nil{log.Printf("Anerroroccurred:%v",err)return}. 2. Create a custom error type to provide more information, such as typeMyErrorstruct{MsgstringDetailstring}. 3. Use error wrappers (since Go1.13) to add context without losing the original error message,
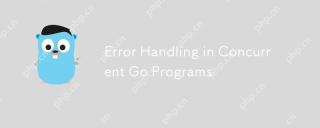
ToeffectivelyhandleerrorsinconcurrentGoprograms,usechannelstocommunicateerrors,implementerrorwatchers,considertimeouts,usebufferedchannels,andprovideclearerrormessages.1)Usechannelstopasserrorsfromgoroutinestothemainfunction.2)Implementanerrorwatcher
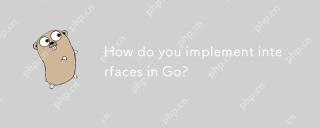
In Go language, the implementation of the interface is performed implicitly. 1) Implicit implementation: As long as the type contains all methods defined by the interface, the interface will be automatically satisfied. 2) Empty interface: All types of interface{} types are implemented, and moderate use can avoid type safety problems. 3) Interface isolation: Design a small but focused interface to improve the maintainability and reusability of the code. 4) Test: The interface helps to unit test by mocking dependencies. 5) Error handling: The error can be handled uniformly through the interface.
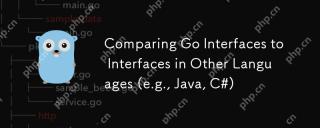
Go'sinterfacesareimplicitlyimplemented,unlikeJavaandC#whichrequireexplicitimplementation.1)InGo,anytypewiththerequiredmethodsautomaticallyimplementsaninterface,promotingsimplicityandflexibility.2)JavaandC#demandexplicitinterfacedeclarations,offeringc
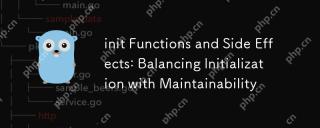
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
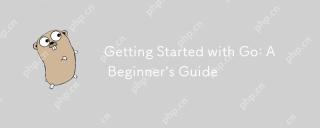
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
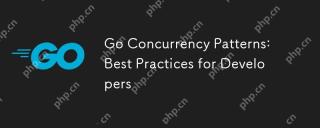
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
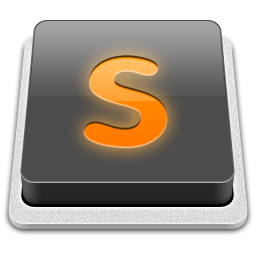
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
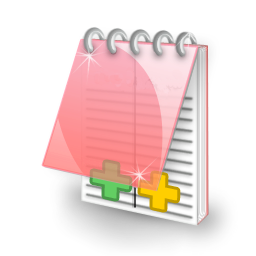
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
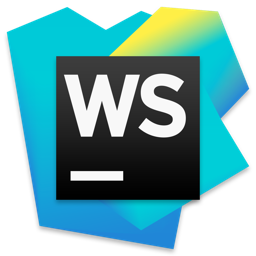
WebStorm Mac version
Useful JavaScript development tools
