A Practical Guide to Laravel Form Classes: Solutions to Common Problems
Practical Guide to Laravel Form Classes: Solutions to Common Problems
In web development, forms are one of the indispensable elements, and Laravel, as a popular PHP framework provides powerful form processing functions. However, some problems are often encountered during the development process, such as form validation, data storage, etc. This article will provide solutions to these common problems and attach specific code examples.
- Form validation
In Laravel, form validation is a very important part, which can effectively prevent users from entering data that does not meet the requirements. Through Laravel's validator class, developers can easily implement form validation. The following is a simple example that demonstrates how to use Laravel's validator class to validate user-entered data:
public function store(Request $request) { $validatedData = $request->validate([ 'name' => 'required|string|max:255', 'email' => 'required|email|max:255', 'password' => 'required|min:6', ]); // 数据存储逻辑 }
In the above code, we define the field validation rules through the validate
method, When the user submits the form, Laravel will automatically perform data validation and return an error message if the validation fails.
- Form data storage
After the form verification is passed, we usually need to store the data submitted by the user into the database. Laravel provides the Eloquent model to conveniently operate the database. The following is a sample code:
use AppModelsUser; public function store(Request $request) { $user = new User(); $user->name = $request->input('name'); $user->email = $request->input('email'); $user->password = bcrypt($request->input('password')); $user->save(); }
In the above code, we first instantiate a User model, then assign the data entered by the user to the attributes of the model, and finally Call the save
method to store the data in the database.
- Form redirection
Page redirection usually occurs after the form is submitted. You can use the redirect
method provided by Laravel to achieve page redirection. The following is a simple example:
public function store(Request $request) { // 数据处理逻辑 return redirect()->route('success')->with('message', '表单提交成功!'); }
In the above code, we redirect the user to a route named success
through the redirect
method, and pass a success information.
Summary
Through the above practical guide, we have learned about common problem solutions when processing forms in Laravel, and given specific code examples. By rationally using Laravel's form classes, we can develop web applications more efficiently and improve user experience. I hope the above content can be helpful to the problems developers encounter in actual projects.
The above is the detailed content of A Practical Guide to Laravel Form Classes: Solutions to Common Problems. For more information, please follow other related articles on the PHP Chinese website!

Laravel stands out by simplifying the web development process and delivering powerful features. Its advantages include: 1) concise syntax and powerful ORM system, 2) efficient routing and authentication system, 3) rich third-party library support, allowing developers to focus on writing elegant code and improve development efficiency.

Laravelispredominantlyabackendframework,designedforserver-sidelogic,databasemanagement,andAPIdevelopment,thoughitalsosupportsfrontenddevelopmentwithBladetemplates.

Laravel and Python have their own advantages and disadvantages in terms of performance and scalability. Laravel improves performance through asynchronous processing and queueing systems, but due to PHP limitations, there may be bottlenecks when high concurrency is present; Python performs well with the asynchronous framework and a powerful library ecosystem, but is affected by GIL in a multi-threaded environment.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.

Laravel can be used for front-end development. 1) Use the Blade template engine to generate HTML. 2) Integrate Vite to manage front-end resources. 3) Build SPA, PWA or static website. 4) Combine routing, middleware and EloquentORM to create a complete web application.

PHP and Laravel can be used to build efficient server-side applications. 1.PHP is an open source scripting language suitable for web development. 2.Laravel provides routing, controller, EloquentORM, Blade template engine and other functions to simplify development. 3. Improve application performance and security through caching, code optimization and security measures. 4. Test and deployment strategies to ensure stable operation of applications.

Laravel and Python have their own advantages and disadvantages in terms of learning curve and ease of use. Laravel is suitable for rapid development of web applications. The learning curve is relatively flat, but it takes time to master advanced functions. Python's grammar is concise and the learning curve is flat, but dynamic type systems need to be cautious.

Laravel's advantages in back-end development include: 1) elegant syntax and EloquentORM simplify the development process; 2) rich ecosystem and active community support; 3) improved development efficiency and code quality. Laravel's design allows developers to develop more efficiently and improve code quality through its powerful features and tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
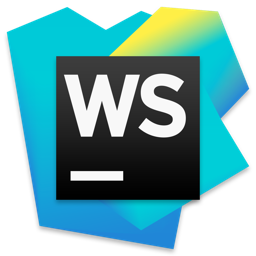
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
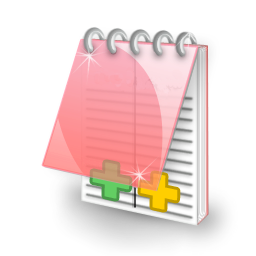
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software