"Revealing the Mysteries of Java RESTful API Creation: A Step-by-Step Guide" carefully created by php editor Banana is designed to help developers deeply understand the creation process of Java RESTful API. This guide will introduce relevant concepts, technologies, and best practices step by step, allowing readers to easily master the key points of creating RESTful APIs and improve development efficiency and technical level. Whether you are a newbie or an experienced developer, this guide will reveal the secrets of Java RESTful API and help you navigate the development process with ease.
Set up development environment
- Install the Java Development Tools package (jdk) and Maven Build Tools.
- Create a Maven project and add spring Boot and JAX-RS dependencies.
Writing Resources
Resources are data models in RESTful APIs. They represent data entities with which clients interact. For example, you can create a Customer
resource to represent customer information.
@Entity @Table(name = "customers") public class Customer { @Id @GeneratedValue private Long id; private String name; private String email; // ...其他属性 }
Create API endpoint
An API endpoint is the URL path that clients use to communicate with your API. You can use JAX-RS annotations (such as @GET
, @POST
) to define endpoints and specify the HTTP methods they handle.
@RestController @RequestMapping("/api/customers") public class CustomerController { @Autowired private CustomerService customerService; @GetMapping public List<Customer> getAllCustomers() { return customerService.findAll(); } @PostMapping public Customer createCustomer(@RequestBody Customer customer) { return customerService.save(customer); } // ...其他端点 }
Handling HTTP methods
RESTful API supports GET, POST, PUT, DELETE and other HTTP methods. You can use JAX-RS annotations to specify which methods are supported by each endpoint.
@GET public List<Customer> getAllCustomers() { // 获取所有客户 } @POST public Customer createCustomer(@RequestBody Customer customer) { // 创建一个新客户 } @PUT public Customer updateCustomer(@RequestBody Customer customer) { // 更新一个现有客户 } @DELETE public void deleteCustomer(@PathVariable Long id) { // 删除一个客户 }
Error handling
Error handling is crucial for any API. You can use Spring Boot's @Except<strong class="keylink">io</strong>nHandler
annotation to handle specific exceptions and return an appropriate error response.
@RestControllerAdvice public class GlobalExceptionHandler { @ExceptionHandler(NotFoundException.class) public ResponseEntity<String> handleNotFoundException(NotFoundException ex) { return new ResponseEntity<>(ex.getMessage(), httpstatus.NOT_FOUND); } // ...其他异常处理程序 }
Test your API
Using Postman or similar toolsTestingIt is very important to use Postman or similar tools. You should test all endpoints to ensure they work properly and return expected responses.
Deployment API
You can deploy your API using Spring Boot's built-in server . You can also use a third-party deployment platform such as Heroku or AWS.
in conclusion
Creating a Java RESTful API is a relatively simple process. By following the steps in this guide, you can quickly build a powerful and scalable API. With continuous practice and improvement, you can become a proficient RESTful API developer.
The above is the detailed content of Uncovering the Secrets of Java RESTful API Creation: A Step-by-Step Guide. For more information, please follow other related articles on the PHP Chinese website!
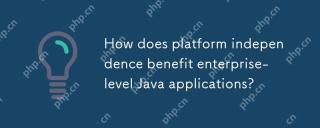
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
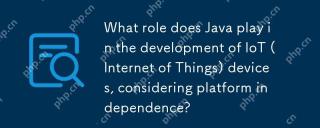
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
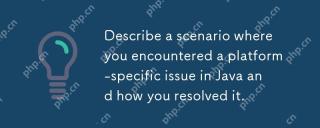
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
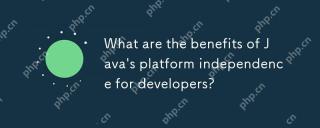
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
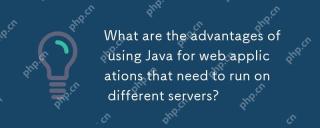
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
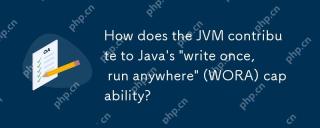
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
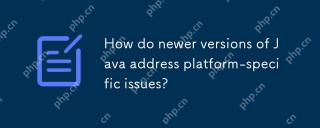
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
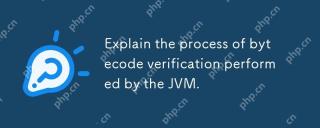
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
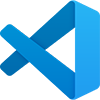
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
