


How does the JVM contribute to Java's 'write once, run anywhere' (WORA) capability?
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
introduction
Java's "Written at once, run around" (WORA) feature is a key reason why many programmers choose it. This article aims to explore in-depth how Java Virtual Machine (JVM) supports this feature. By reading this article, you will learn about the internal mechanisms of the JVM and how it ensures consistency and compatibility of Java code across different platforms.
Basic concepts of JVM
JVM is the running environment of Java programs that convert Java bytecode into machine code that can be executed on specific hardware and operating systems. The Java program is first compiled into a platform-independent bytecode file (.class file), which is then interpreted and executed by the JVM. JVM blocks differences in the underlying operating system, allowing Java programs to run on any system with JVM installed.
How to implement WORA by JVM
JVM implements WORA through the following key mechanisms:
Bytecode and interpretation execution
Java source code is compiled into bytecode, rather than directly compiled into machine code specific to a certain operating system. The JVM interprets these bytecodes as machine code on the target machine at runtime. This means that as long as the target machine has the appropriate JVM, the Java program can run.
// Example: Java source code to bytecode conversion public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
This simple HelloWorld program is compiled into bytecode and then executed by the JVM on any supported platform.
Platform-independent API
The Java standard library provides a set of platform-independent APIs that abstract the concrete implementation of the underlying operating system. For example, file I/O operations perform consistently on Windows and Linux because the JVM handles the underlying differences.
// Example: File I/O API using Java import java.io.File; import java.io.FileWriter; import java.io.IOException; <p>public class FileExample { public static void main(String[] args) { try { File file = new File("example.txt"); FileWriter writer = new FileWriter(file); writer.write("Hello, File!"); writer.close(); } catch (IOException e) { e.printStackTrace(); } } }</p>
This code will work the same way on any Java-enabled operating system.
Dynamic links and class loading
The JVM uses dynamic linking and class loading mechanisms, which means that classes can be loaded and linked at runtime. This not only improves program flexibility, but also ensures consistent class loading behavior on different platforms.
// Example: Dynamic class loading public class DynamicLoading { public static void main(String[] args) { try { Class> clazz = Class.forName("com.example.MyClass"); Object instance = clazz.getDeclaredConstructor().newInstance(); System.out.println(instance); } catch (Exception e) { e.printStackTrace(); } } }
This example shows how classes can be loaded and instantiated dynamically at runtime, which works on different platforms.
The advantages and disadvantages of JVM and the pitfalls
advantage
- Cross-platformity : JVM enables Java programs to run on any Java-enabled operating system.
- Security : JVM provides a sandbox environment to protect the system from malicious code attacks.
- Memory management : JVM automatically handles memory allocation and garbage collection, simplifying the development process.
Disadvantages and challenges
- Performance overhead : The JVM may introduce performance overhead because of the need to interpret execution. While modern JVMs alleviate this problem through JIT compilers, it still exists in some cases.
- Startup time : The JVM starts up time, especially in complex applications.
- Memory consumption : The JVM itself requires a certain amount of memory resources, which may become a problem in resource-constrained environments.
Tap points and suggestions
- Version compatibility : There may be API differences between different versions of JVMs, ensuring that your code is tested on the target platform.
- Local code call : When using JNI (Java Native Interface), you need to pay attention to compatibility issues on different operating systems.
- Garbage collection : Understand the JVM's garbage collection mechanism to avoid performance problems caused by memory leaks or frequent GC.
Performance optimization and best practices
In practical applications, optimizing the performance of the JVM is key. Here are some suggestions:
- Using JIT compiler : Modern JVM compiles hotspot code into machine code through JIT compiler, significantly improving performance.
- Adjust JVM parameters : Adjust JVM parameters according to the specific needs of the application, such as heap size, GC policy, etc.
- Code optimization : Write efficient Java code to reduce unnecessary object creation and method calls.
// Example: Adjust JVM parameters java -Xmx1024m -Xms512m -XX: UseG1GC MyApplication
This command adjusts the maximum heap size, minimum heap size, and GC policy, and is optimized for specific applications.
Conclusion
JVM is the core of Java WORA features. Through mechanisms such as bytecode interpretation, platform-independent APIs and dynamic class loading, JVM ensures cross-platform consistency of Java programs. Understanding how JVM works and optimization strategies can help you better utilize the advantages of Java and write efficient and portable code. In actual development, paying attention to version compatibility, JNI calls and garbage collection issues can avoid common pitfalls and improve application performance and stability.
The above is the detailed content of How does the JVM contribute to Java's 'write once, run anywhere' (WORA) capability?. For more information, please follow other related articles on the PHP Chinese website!
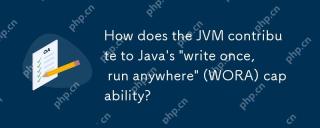
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
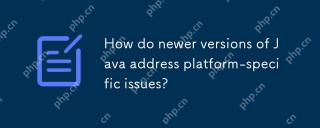
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
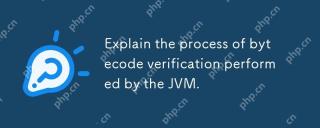
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.
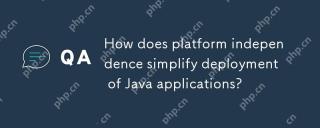
Java'splatformindependenceallowsapplicationstorunonanyoperatingsystemwithaJVM.1)Singlecodebase:writeandcompileonceforallplatforms.2)Easyupdates:updatebytecodeforsimultaneousdeployment.3)Testingefficiency:testononeplatformforuniversalbehavior.4)Scalab
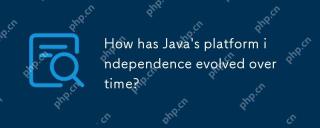
Java's platform independence is continuously enhanced through technologies such as JVM, JIT compilation, standardization, generics, lambda expressions and ProjectPanama. Since the 1990s, Java has evolved from basic JVM to high-performance modern JVM, ensuring consistency and efficiency of code across different platforms.
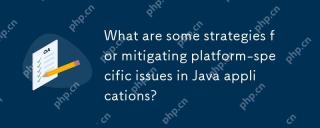
How does Java alleviate platform-specific problems? Java implements platform-independent through JVM and standard libraries. 1) Use bytecode and JVM to abstract the operating system differences; 2) The standard library provides cross-platform APIs, such as Paths class processing file paths, and Charset class processing character encoding; 3) Use configuration files and multi-platform testing in actual projects for optimization and debugging.
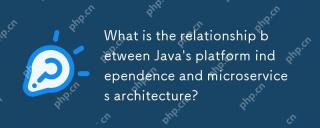
Java'splatformindependenceenhancesmicroservicesarchitecturebyofferingdeploymentflexibility,consistency,scalability,andportability.1)DeploymentflexibilityallowsmicroservicestorunonanyplatformwithaJVM.2)Consistencyacrossservicessimplifiesdevelopmentand
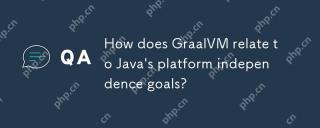
GraalVM enhances Java's platform independence in three ways: 1. Cross-language interoperability, allowing Java to seamlessly interoperate with other languages; 2. Independent runtime environment, compile Java programs into local executable files through GraalVMNativeImage; 3. Performance optimization, Graal compiler generates efficient machine code to improve the performance and consistency of Java programs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
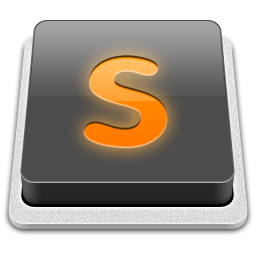
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor
