Basic principles of XML parsing
XML (Extensible Markup Language) is a markup language widely used for data exchange and storage. XML parsers are responsible for converting XML documents into Java objects so that we can process and manipulate XML data.
DOM parsing
DOM (Document Object Model) is a parsing method based on a tree structure. It loads the XML document into memory and builds an object tree corresponding to the document structure. This approach provides complete access and manipulation of the XML document, but is more expensive.
Demo code:
import org.w3c.dom.*; public class DOMParser { public static void main(String[] args) { DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); try { DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("example.xml"); // 访问根元素 Element rootElement = document.getDocumentElement(); System.out.println("Root element: " + rootElement.getnodeName()); // 遍历子元素 NodeList childNodes = rootElement.getChildNodes(); for (int i = 0; i < childNodes.getLength(); i++) { Node childNode = childNodes.item(i); if (childNode.getNodeType() == Node.ELEMENT_NODE) { System.out.println("Child element: " + childNode.getNodeName()); } } } catch (Exception e) { e.printStackTrace(); } } }
SAX parsing
SAX (Simple api for XML) is an event-driven parsing method. It parses XML documents line by line in a streaming manner and provides an event interface to handle events during the parsing process. This approach is less expensive but provides limited access and manipulation of the XML document.
Demo code:
import org.xml.sax.Attributes; import org.xml.sax.SAXException; import org.xml.sax.helpers.DefaultHandler; public class SAXParser extends DefaultHandler { @Override public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException { System.out.println("Start element: " + qName); } @Override public void endElement(String uri, String localName, String qName) throws SAXException { System.out.println("End element: " + qName); } @Override public void characters(char[] ch, int start, int length) throws SAXException { String data = new String(ch, start, length); System.out.println("Text content: " + data); } public static void main(String[] args) { try { SAXParserFactory factory = SAXParserFactory.newInstance(); SAXParser parser = factory.newSAXParser(); parser.parse("example.xml", new SAXParser()); } catch (Exception e) { e.printStackTrace(); } } }
Choose the appropriate parsing method
DOM and SAX are both valid XML parsing methods, and choosing the appropriate parsing method depends on your specific needs. If complete access and manipulation of XML documents is required, DOM is the best choice. If fast parsing and low overhead are required, SAX is a better choice.
Performance optimization
XML parsing can become a performance bottleneck for your application. The following are some suggestions for optimizing parsing performance:
- Use caching mechanism to reduce repeated parsing.
- Optimize the XML document structure to improve parsing efficiency.
- Use asynchronous parsing technology such as SAX to avoid blocking.
- Consider using a memory mapped XML parser to improve performance.
Summarize
Mastering XML parsing is critical to efficiently processing and manipulating XML data. DOM and SAX are two popular parsing methods in Java. They have different characteristics and applicable scenarios. By understanding these methods and choosing the appropriate parsing method, you can take full advantage of XML parsing technology and improve the performance and efficiency of your applications.
The above is the detailed content of Demystifying the Art of Java XML Parsing: Mastering DOM and SAX. For more information, please follow other related articles on the PHP Chinese website!
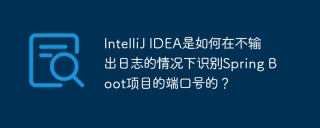
Start Spring using IntelliJIDEAUltimate version...
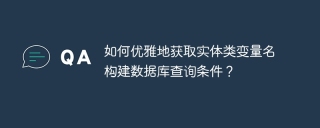
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
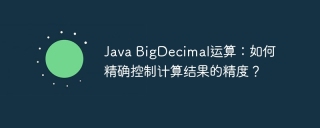
Java...
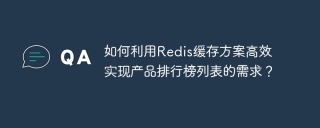
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
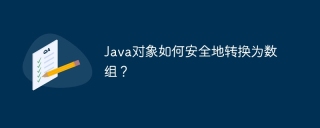
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
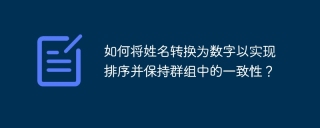
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
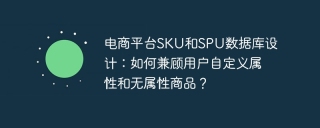
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
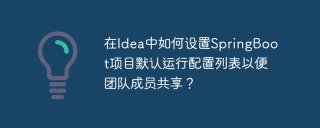
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
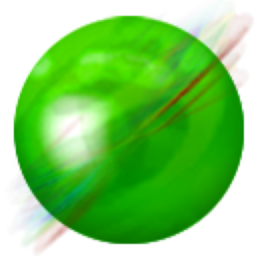
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
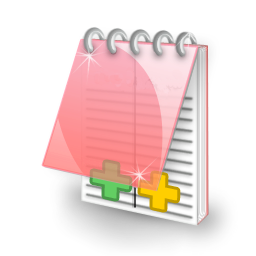
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor