


In PHP development, we often encounter situations where we need to use the POST method to pass parameters and jump to the page. This is particularly common in scenarios such as form submission and user login. This article will introduce how to use the POST method to pass parameters in PHP, and demonstrate how to implement page jumps through code examples.
First of all, we need to understand the difference between the POST method and the GET method when passing parameters. The GET method passes parameters to the server through the URL, and the parameters will be displayed in the URL address, while the POST method hides the parameters in the message body of the HTTP request and passes them to the server, which is safer. Therefore, for some operations involving sensitive user information, we usually choose to use the POST method.
Next, we use a simple example to demonstrate how to use the POST method to pass parameters and jump to the page. Suppose we have a login page where the user needs to enter a username and password. We pass these two parameters to the server through the POST method to verify the login information, and jump to different pages based on the verification results.
First, create an HTML form for users to enter their username and password, and submit the form to a PHP processing page.
<!DOCTYPE html> <html> <head> <title>Login</title> </head> <body> <h2 id="Login-Form">Login Form</h2> <form action="login.php" method="post"> <label for="username">Username:</label> <input type="text" id="username" name="username"><br><br> <label for="password">Password:</label> <input type="password" id="password" name="password"><br><br> <input type="submit" value="Login"> </form> </body> </html>
In the above form, we use the POST method to submit the username and password to the PHP processing page named login.php
. Next, we will write the login.php
file to verify the login information and jump to different pages based on the verification results.
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST['username']; $password = $_POST['password']; // 这里可以根据实际需求进行登录验证 // 假设用户名为"admin",密码为"123456"才能登录成功 if ($username === 'admin' && $password === '123456') { header('Location: welcome.php'); exit; } else { header('Location: login_failed.php'); exit; } } ?>
In login.php
, we first obtain the username and password entered by the user through $_POST
, and then perform simple login verification. If the username and password are correct, use the header
function to jump to welcome.php
, otherwise jump to login_failed.php
.
Finally, we create welcome.php
and login_failed.php
pages to display login success and failure information. The content can be designed according to actual needs.
Through the above examples, we learned how to use the POST method in PHP to pass parameters and jump to the page. This is often encountered in actual development. I hope the examples in this article can help everyone understand and apply the method of passing parameters through the POST method.
(Note: The above example is a simplified example, security, exception handling and other issues should be considered in actual development.)
The above is the detailed content of PHP Tutorial: Learn how to use the POST method to pass parameters and jump to the page. For more information, please follow other related articles on the PHP Chinese website!
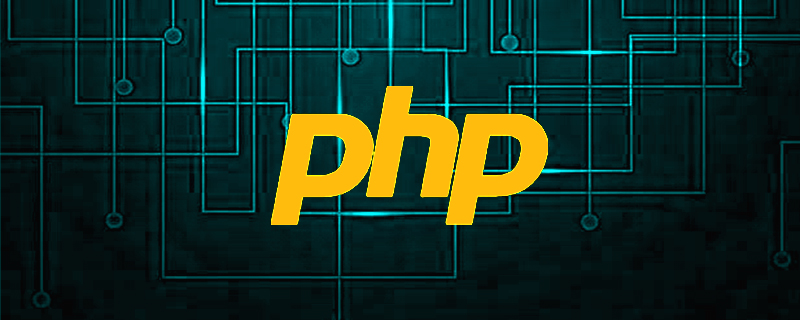
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
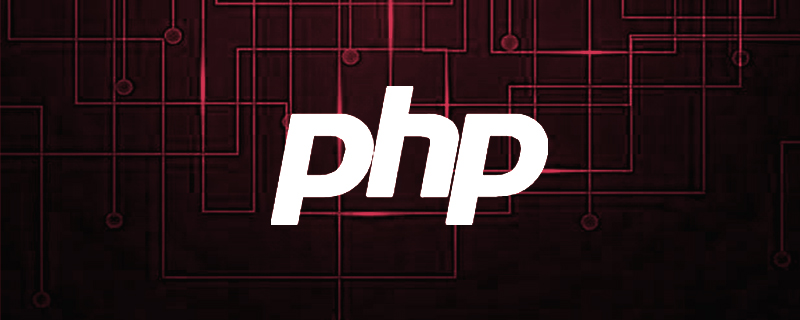
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
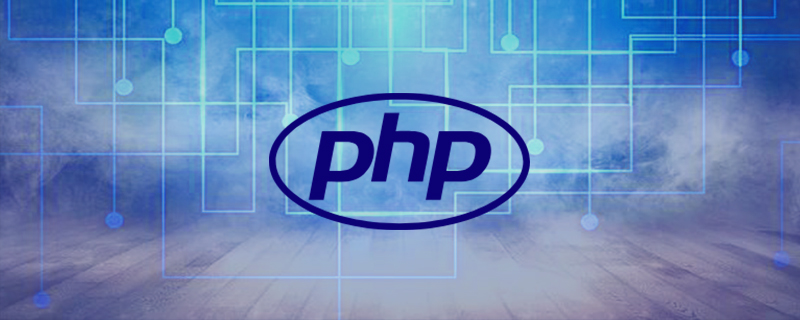
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
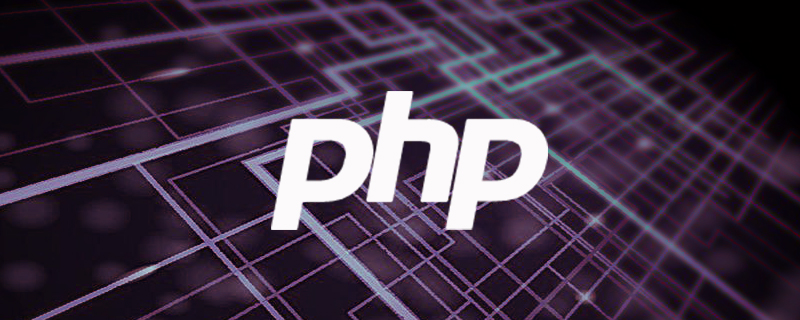
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
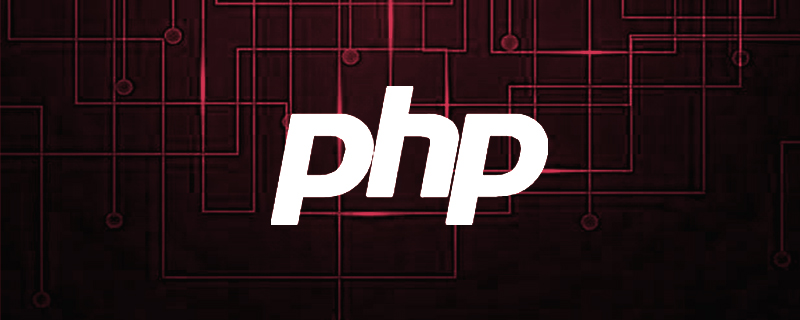
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
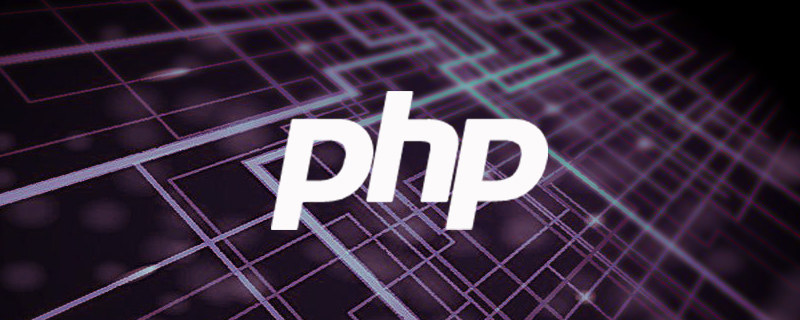
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
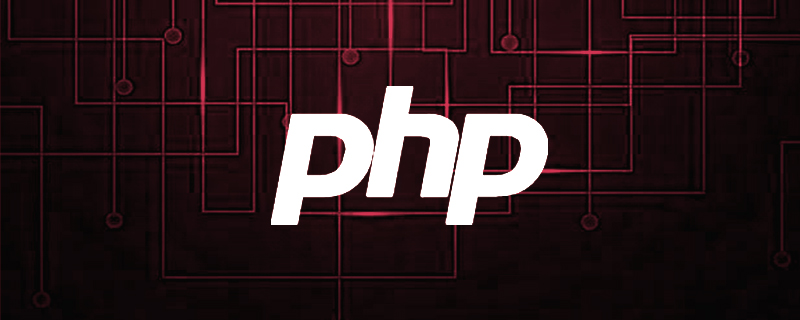
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
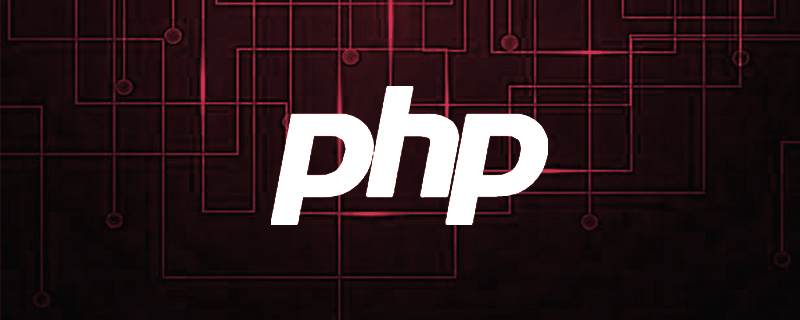
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
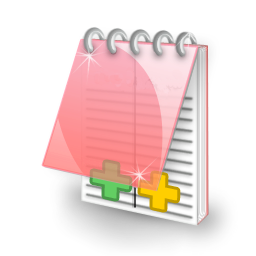
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
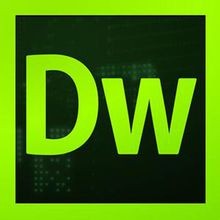
Dreamweaver CS6
Visual web development tools
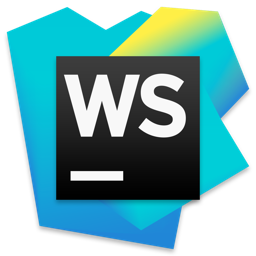
WebStorm Mac version
Useful JavaScript development tools
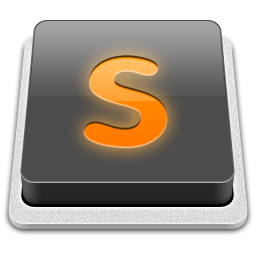
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
