How to use Golang to create an efficient game development framework
As a popular and efficient programming language in the industry, Golang is also widely used in the field of game development. This article will introduce how to use Golang to create an efficient game development framework and provide specific code examples. We will use a simple 2D game as an example to explain.
Part One: Game Engine Construction
First, we need to build a simple game engine, including game loop, graphics rendering and other functions. The following is a simple game engine framework:
package engine import ( "github.com/veandco/go-sdl2/sdl" ) type Engine struct { window *sdl.Window renderer *sdl.Renderer } func NewEngine() *Engine { err := sdl.Init(sdl.INIT_EVERYTHING) if err != nil { panic(err) } window, err := sdl.CreateWindow("Game", sdl.WINDOWPOS_UNDEFINED, sdl.WINDOWPOS_UNDEFINED, 800, 600, sdl.WINDOW_SHOWN) if err != nil { panic(err) } renderer, err := sdl.CreateRenderer(window, -1, sdl.RENDERER_ACCELERATED) if err != nil { panic(err) } return &Engine{ window: window, renderer: renderer, } } func (e *Engine) Run() { defer sdl.Quit() defer e.window.Destroy() defer e.renderer.Destroy() for { e.renderer.SetDrawColor(255, 255, 255, 255) e.renderer.Clear() // 渲染游戏画面 e.renderer.Present() } }
In this code, we create an Engine structure, which contains the window and renderer. In the Run()
method, we continuously render the game screen in a loop.
Part 2: Game Objects and Collision Detection
In the game, there are many game objects, such as characters, enemies, etc. We can define a basic GameObject structure to represent these objects and implement simple collision detection logic.
package engine type GameObject struct { x, y, width, height float32 } func (o *GameObject) CollidesWith(other *GameObject) bool { return o.x < other.x+other.width && o.x+o.width > other.x && o.y < other.y+other.height && o.y+o.height > other.y }
In this code, we define a GameObject structure that contains position and size information. And implemented the CollidesWith()
method to detect whether two game objects collide.
Part 3: Event Handling and Input
In games, user input is crucial. We can implement user interaction by listening to keyboard events.
package engine func (e *Engine) HandleEvents() { for { for event := sdl.PollEvent(); event != nil; event = sdl.PollEvent() { switch event.(type) { case *sdl.QuitEvent: return } } } }
In this code, we implement a simple event processing function HandleEvents()
, which closes the game window by monitoring whether there is an exit event.
Part 4: Game Main Loop
Finally, we integrate the above content into a simple game.
package main import "github.com/your-github-name/your-game/engine" func main() { engine := engine.NewEngine() go engine.Run() engine.HandleEvents() }
Through the above steps, we successfully built a simple game development framework and implemented basic functions such as game running, object collision detection, and event processing. Of course, the specific game logic and content still need to be expanded and improved according to actual needs. I hope this article can help readers who are interested in Golang game development. I wish you all a happy game development!
The above is the detailed content of How to use Golang to create an efficient game development framework. For more information, please follow other related articles on the PHP Chinese website!
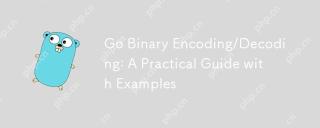
Go's encoding/binary package is a tool for processing binary data. 1) It supports small-endian and large-endian endian byte order and can be used in network protocols and file formats. 2) The encoding and decoding of complex structures can be handled through Read and Write functions. 3) Pay attention to the consistency of byte order and data type when using it, especially when data is transmitted between different systems. This package is suitable for efficient processing of binary data, but requires careful management of byte slices and lengths.
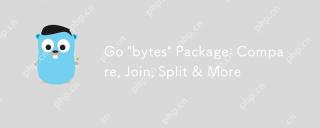
The"bytes"packageinGoisessentialbecauseitoffersefficientoperationsonbyteslices,crucialforbinarydatahandling,textprocessing,andnetworkcommunications.Byteslicesaremutable,allowingforperformance-enhancingin-placemodifications,makingthispackage
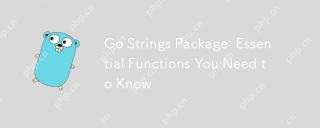
Go'sstringspackageincludesessentialfunctionslikeContains,TrimSpace,Split,andReplaceAll.1)Containsefficientlychecksforsubstrings.2)TrimSpaceremoveswhitespacetoensuredataintegrity.3)SplitparsesstructuredtextlikeCSV.4)ReplaceAlltransformstextaccordingto
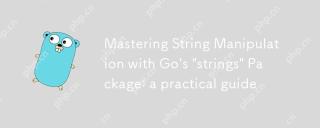
ThestringspackageinGoiscrucialforefficientstringmanipulationduetoitsoptimizedfunctionsandUnicodesupport.1)ItsimplifiesoperationswithfunctionslikeContains,Join,Split,andReplaceAll.2)IthandlesUTF-8encoding,ensuringcorrectmanipulationofUnicodecharacters
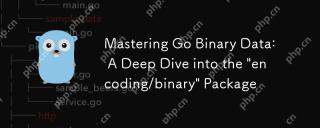
The"encoding/binary"packageinGoiscrucialforefficientbinarydatamanipulation,offeringperformancebenefitsinnetworkprogramming,fileI/O,andsystemoperations.Itsupportsendiannessflexibility,handlesvariousdatatypes,andisessentialforcustomprotocolsa
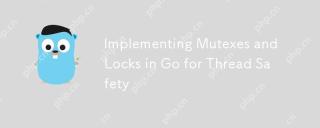
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
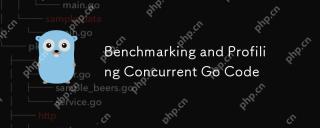
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
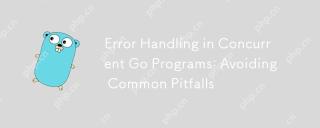
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
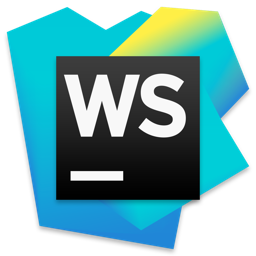
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
