Go's encoding/binary package is a tool for processing binary data. 1) It supports small-endian and large-endian endian endianness and can be used in network protocols and file formats. 2) The encoding and decoding of complex structures can be handled through Read and Write functions. 3) Pay attention to the consistency of byte order and data type when using it, especially when transmitting data between different systems. This package is suitable for efficient processing of binary data, but requires careful management of byte slices and lengths.
Let's dive into the fascinating world of Go's binary encoding and decoding. Ever wondered how data gets transformed into a format that machines can efficiently process? Or how you can ensure your data remains intact when transmitted across networks? Let's explore this together, and by the end of this journey, you'll have a solid grap on using Go's binary package to encode and decode data.
In Go, the encoding/binary
package is your go-to tool for dealing with binary data. Whether you're working on network protocols, file formats, or any other scenario where binary data manipulation is cruel, mastering this package can significantly enhance your programming skills. Let's start with a basic example to see it in action.
package main import ( "encoding/binary" "fmt" "log" ) func main() { var num uint32 = 123456789 var buf [4]byte // Encode the number into a byte slice using little-endian binary.LittleEndian.PutUint32(buf[:], num) fmt.Printf("Encoded: %v\n", buf) // Decode the byte slice back into a number decodedNum := binary.LittleEndian.Uint32(buf[:]) fmt.Printf("Decoded: %d\n", decodedNum) }
This code snippet demonstrates how to encode an integer into a byte slice and then decode it back. But why stop here? Let's delve deeper into the mechanics of binary encoding and explore some advanced use cases.
The encoding/binary
package supports both little-endian and big-endian byte orders. Choosing the right byte order can be critical, especially when working with different systems or protocols. For instance, if you're dealing with a network protocol that specifies big-endian, you'd use binary.BigEndian
. Here's an example showingcasing both:
package main import ( "encoding/binary" "fmt" ) func main() { var num uint32 = 123456789 var buf [4]byte // Little-endian encoding binary.LittleEndian.PutUint32(buf[:], num) fmt.Printf("Little-endian: %v\n", buf) // Big-endian encoding binary.BigEndian.PutUint32(buf[:], num) fmt.Printf("Big-endian: %v\n", buf) }
When working with binary data, it's cruel to understand the implications of byte order. Little-endian is commonly used in x86 architecture, while big-endian is often found in network protocols like IPv4 and IPv6. This choice can affect how you interact with other systems or how you store data.
Now, let's talk about some advanced scenarios. What if you need to encode and decode more complex structures? Go's encoding/binary
package provides functions like Read
and Write
to handle this. Here's an example of encoding and decoding a custom struct:
package main import ( "encoding/binary" "fmt" "log" ) type Person struct { Name string Age uint8 } func main() { person := Person{ Name: "Alice", Age: 30, } // Encode the struct var buf []byte buf = append(buf, byte(len(person.Name))) buf = append(buf, person.Name...) buf = append(buf, person.Age) // Decode the struct var decodedPerson Person nameLength := int(buf[0]) decodedPerson.Name = string(buf[1: 1 nameLength]) decodedPerson.Age = buf[1 nameLength] fmt.Printf("Original: %v\n", person) fmt.Printf("Decoded: %v\n", decodedPerson) }
This example shows how to manually encode and decode a struct. But be aware, this approach requires careful management of byte slices and lengths. A more robust solution might involve using encoding/gob
or encoding/json
for serialization, but they come with their own overhead and are not always suitable for binary data.
Speaking of pitfalls, one common mistake is assuming that the binary representation of data will be the same across different systems. This isn't always true, especially when dealing with floating-point numbers or different integer sizes. Always ensure you're using the correct byte order and data type when encoding and decoding.
Another challenge is dealing with endianness when working with existing binary formats. If you're interfacing with a legacy system or a specific protocol, you'll need to ensure your Go code matches the expected byte order. This can sometimes lead to subtle bugs if not handled correctly.
Performance is another aspect to consider. Binary encoding and decoding are generally fast, but if you're dealing with large amounts of data, you might need to optimize your code. One strategy is to use io.Reader
and io.Writer
interfaces to stream data instead of loading everything into memory at once.
Finally, let's talk about best practices. Always document your binary format clearly, especially if you're defining a custom format. This helps other developers understand how to work with your data. Also, consider using existing formats or protocols when possible, as they often have well-defined specifications and tools for handling them.
In conclusion, Go's encoding/binary
package is a powerful tool for working with binary data. By understanding its capabilities and limitations, you can write efficient and robust code for a wide range of applications. Keep experimenting, and don't be afraid to dive deep into the specifications of your data formats. Happy coding!
The above is the detailed content of Go Binary Encoding/Decoding: A Practical Guide with Examples. For more information, please follow other related articles on the PHP Chinese website!
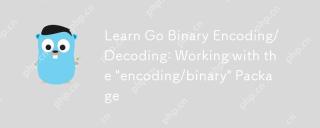
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
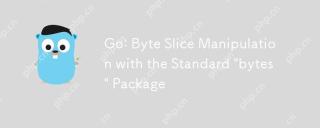
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
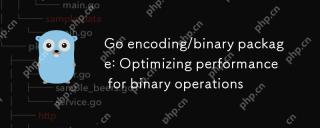
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
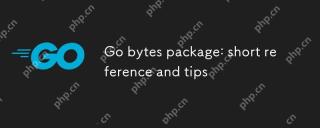
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
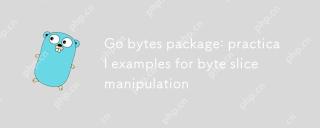
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.
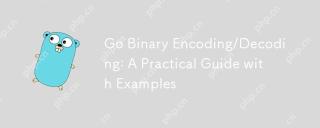
Go's encoding/binary package is a tool for processing binary data. 1) It supports small-endian and large-endian endian byte order and can be used in network protocols and file formats. 2) The encoding and decoding of complex structures can be handled through Read and Write functions. 3) Pay attention to the consistency of byte order and data type when using it, especially when data is transmitted between different systems. This package is suitable for efficient processing of binary data, but requires careful management of byte slices and lengths.
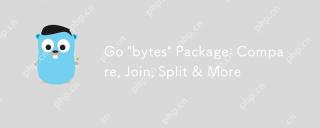
The"bytes"packageinGoisessentialbecauseitoffersefficientoperationsonbyteslices,crucialforbinarydatahandling,textprocessing,andnetworkcommunications.Byteslicesaremutable,allowingforperformance-enhancingin-placemodifications,makingthispackage
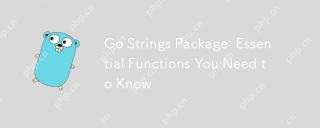
Go'sstringspackageincludesessentialfunctionslikeContains,TrimSpace,Split,andReplaceAll.1)Containsefficientlychecksforsubstrings.2)TrimSpaceremoveswhitespacetoensuredataintegrity.3)SplitparsesstructuredtextlikeCSV.4)ReplaceAlltransformstextaccordingto


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
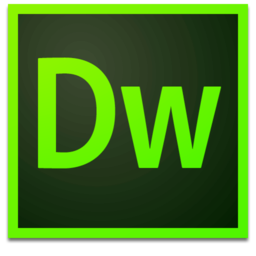
Dreamweaver Mac version
Visual web development tools
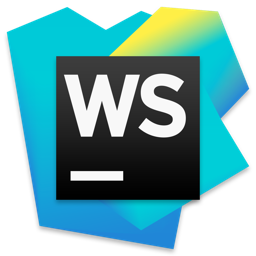
WebStorm Mac version
Useful JavaScript development tools
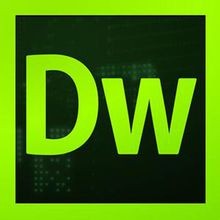
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
