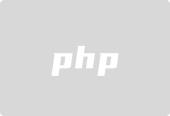
Go language (Golang), as a modern programming language, has been favored by developers since its birth. It has many advantages and applicable scenarios, and has become one of the preferred languages for many large Internet companies. This article will introduce the advantages and applicable scenarios of the Go language, and demonstrate its powerful features through specific code examples.
Advantages of Go language
-
Simple and efficient: Go language is a concise and efficient language with high performance. Its syntax is streamlined, easy to use, and suitable for rapid development and iteration.
-
Concurrency model: The Go language has built-in support for lightweight thread goroutines and channels, making concurrent programming simple and efficient. This concurrency model does not require developers to manually manage threads and avoids common concurrency problems.
-
Built-in Tools: Go language has a rich set of built-in tools, such as its own testing tools, code formatting tools, performance analysis tools, etc., which can improve development efficiency and quality.
-
Cross-platform support: Go language supports multiple operating systems and architectures, and the developed programs can run seamlessly on different platforms.
-
Static typing: Go language is a statically typed language that can detect many errors during compilation, improving the robustness and maintainability of the code.
Applicable scenarios of Go language
-
Web development: The lightweight and efficient features of Go language make it very suitable for building the Web Services and Applications. Its standard library provides rich HTTP functions and can quickly build HTTP services.
-
Cloud native application: Due to the high concurrency performance and built-in concurrency model of the Go language, it is an ideal choice for building cloud native applications. Many cloud native projects such as Kubernetes, Docker, etc. are developed using the Go language.
-
System Programming: Go language is also suitable for system programming fields, such as network programming, operating system development and high-performance computing.
Code Example
The following will show the elegance and efficiency of Go language through a simple example.
package main
import (
"fmt"
)
func main() {
// 创建一个通道
channel := make(chan string)
// 启动一个goroutine发送数据到通道
go func() {
channel <- "Hello, Go!"
}()
// 主goroutine从通道中接收数据并打印
message := <-channel
fmt.Println(message)
}
In this example, we create a channel channel and use goroutine to send and receive data. This concurrency model is simple and efficient, avoiding the complexity and risks of traditional multi-threaded programming.
To sum up, the Go language has the advantages of simplicity, efficiency, concurrency model, and built-in tools, and is suitable for various scenarios such as web development, cloud native applications, and system programming. Through the above code examples, we have also seen the elegant concurrent programming capabilities of Go language. I hope this article can help readers gain a deeper understanding of the charm of the Go language and inspire their enthusiasm for exploring and learning this language.
The above is the detailed content of Advantages and applicable scenarios of Go language. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn