How to handle errors when downloading PDF files in PHP7
How to handle errors when downloading PDF files in PHP7
In website development, it is often necessary to download PDF files. However, sometimes some errors may occur when using PHP7 to download PDF files, such as the downloaded file cannot be opened, the downloaded file is damaged, etc. This article will introduce how to handle errors when downloading PDF files in PHP7, and provide some specific code examples.
1. Confirm the PDF file path
First make sure your PDF file path is correct, make sure the file exists and there is no problem with the path.
$pdfFilePath = 'pdf/test.pdf'; if (file_exists($pdfFilePath)) { // 下载PDF文件的代码 } else { echo "文件不存在或路径错误!"; }
2. Set HTTP header information
Before downloading a PDF file, you need to set the correct HTTP header information to tell the browser that this is a PDF file and needs to be downloaded.
header('Content-Type: application/pdf'); header('Content-Disposition: attachment; filename="test.pdf"');
3. Output the PDF file content
Use the readfile()
function to output the PDF file content.
$pdfFilePath = 'pdf/test.pdf'; if (file_exists($pdfFilePath)) { header('Content-Type: application/pdf'); header('Content-Disposition: attachment; filename="test.pdf"'); readfile($pdfFilePath); } else { echo "文件不存在或路径错误!"; }
4. Dealing with memory overflow problems
Sometimes memory overflow problems occur when downloading large PDF files. You can use the alternative of readfile()
fopen()
and fread()
to avoid this problem.
$pdfFilePath = 'pdf/big_file.pdf'; if (file_exists($pdfFilePath)) { header('Content-Type: application/pdf'); header('Content-Disposition: attachment; filename="big_file.pdf"'); $fp = fopen($pdfFilePath, 'rb'); while (!feof($fp)) { echo fread($fp, 8192); } fclose($fp); } else { echo "文件不存在或路径错误!"; }
5. Dealing with the problem of garbled downloaded file names
Sometimes the downloaded file name will be garbled. You can use the urlencode()
function to encode the file name.
$fileName = '测试文件.pdf'; header('Content-Type: application/pdf'); header('Content-Disposition: attachment; filename="' . urlencode($fileName) . '"');
Conclusion
Through the above methods, you can effectively solve the problem of errors when downloading PDF files in PHP7. In actual projects, choose the appropriate method to download PDF files based on specific circumstances to ensure that users can successfully download and open PDF files.
The above is the detailed content of How to handle errors when downloading PDF files in PHP7. For more information, please follow other related articles on the PHP Chinese website!
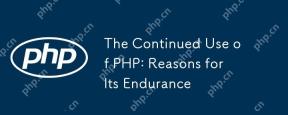
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
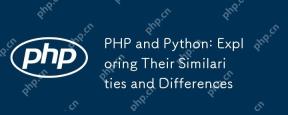
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
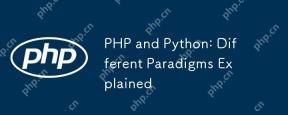
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
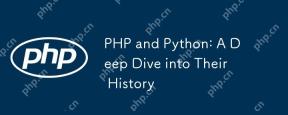
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
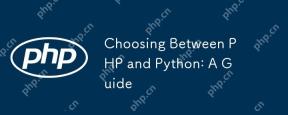
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
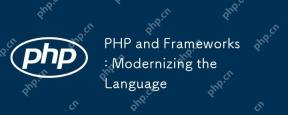
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
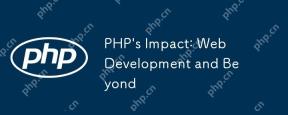
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
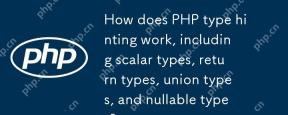
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
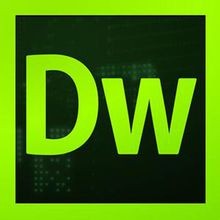
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor