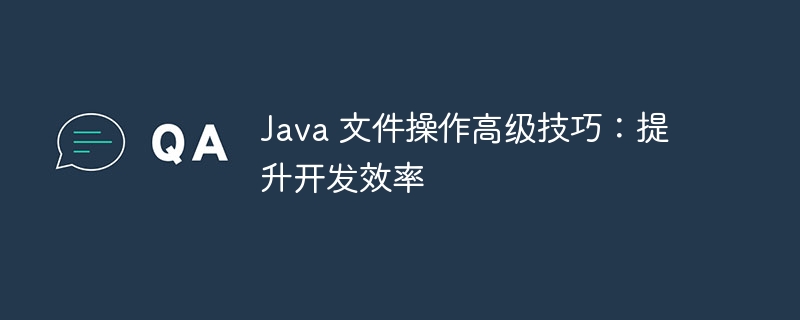
Java file operation is one of the commonly used skills in program development. In actual projects, mastering advanced file operation skills can improve development efficiency. In this article, PHP editor Xinyi introduces you to advanced techniques for Java file operations, including file reading and writing, directory operations, file filtering, etc., to help developers better cope with complex file processing needs and improve code quality and development efficiency.
- Use BufferedReader/BufferedWriter to improve reading and writing efficiency: BufferedReader and BufferedWriter are efficient character streams that can read or write one line of text at a time, which is more efficient than using InputStream or OutputStream directly.
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
BufferedWriter writer = new BufferedWriter(new FileWriter("file.txt"));
- Use Files.readAllBytes/Files.writeAllBytes to read/write files at one time: Files.readAllBytes and Files.writeAllBytes methods can read/write the entire file at one time and are suitable for processing smaller files.
byte[] bytes = Files.readAllBytes(Paths.get("file.txt"));
Files.writeAllBytes(Paths.get("file.txt"), bytes);
2. Efficient file writing
- Use the Files.lines method to write the file line by line: The Files.lines method can write the string list to the file line by line, which is more concise than using BufferedWriter.
List<String> lines = Arrays.asList("line1", "line2");
Files.write(Paths.get("file.txt"), lines);
- Use PrintWriter to write files: PrintWriter is a text output stream that can directly write data to files, eliminating steps such as character encoding conversion.
PrintWriter writer = new PrintWriter("file.txt");
writer.println("line1");
writer.println("line2");
writer.close();
3. Efficient file copy
- Use the Files.copy method to copy files: The Files.copy method can quickly copy one file to another file, supporting source files and target files in different file systems.
Files.copy(Paths.get("file1.txt"), Paths.get("file2.txt"));
- Use the FileChannel.transferTo method to copy files: The FileChannel.transferTo method can efficiently transfer data from one file to another and is suitable for processing large files.
FileChannel inChannel = FileChannel.open(Paths.get("file1.txt"), StandardOpenOption.READ);
FileChannel outChannel = FileChannel.open(Paths.get("file2.txt"), StandardOpenOption.WRITE);
inChannel.transferTo(0, inChannel.size(), outChannel);
4. Efficient file movement
- Use the Files.move method to move files: The Files.move method can move a file to another location, supporting source files and target files in different file systems.
Files.move(Paths.get("file1.txt"), Paths.get("file2.txt"));
- Use the File.renameTo method to move files: The File.renameTo method can rename and move a file to another location. It is more efficient if the source file and target file are in the same directory.
File file1 = new File("file1.txt");
File file2 = new File("file2.txt");
file1.renameTo(file2);
5. Efficient file deletion
- Use the Files.delete method to delete files: The Files.delete method can delete a file and throw an exception if the file does not exist.
Files.delete(Paths.get("file.txt"));
- Use the File.delete method to delete a file: The File.delete method can delete a file and returns false if the file does not exist.
File file = new File("file.txt");
file.delete();
6. File metadata management
- Use the Files.getAttribute method to obtain file attributes: The Files.getAttribute method can obtain file attributes, such as size, creation time, last modification time, etc.
Map<String, Object> attrs = Files.getAttribute(Paths.get("file.txt"), "*");
- Use the Files.setAttribute method to set file attributes: The Files.setAttribute method can set file attributes, such as size, creation time, last modification time, etc.
Files.setAttribute(Paths.get("file.txt"), "creationTime", new PosixFileAttributes.CreationTimeImpl());
7. File lock
- Use the FileChannel.lock method to obtain a fileLock: The FileChannel.lock method can obtain a file lock to prevent other processes from accessing the file.
FileChannel channel = FileChannel.open(Paths.get("file.txt"), StandardOpenOption.WRITE);
FileLock lock = channel.lock();
- Use the FileChannel.release method to release the file lock: The FileChannel.release method can release the file lock and allow other processes to access the file.
lock.release();
Summarize:
This article introduces a variety of advanced Java file operation techniques, including file reading, writing, copying, moving and deleting operations, as well as file metadata management and file locking. Mastering these skills can significantly improve the efficiency of Javadevelopment and lay a solid foundation for writing robust and reliable applications.
>Soft Exam Advanced Exam Preparation Skills/Past Exam Questions/Preparation Essence Materials" target="_blank">Click to download for free>>Soft Exam Advanced Exam Preparation Skills/Past Exam Questions/Exam Preparation Essence Materials
The above is the detailed content of Advanced Java file operation techniques: improve development efficiency. For more information, please follow other related articles on the PHP Chinese website!