


Python Blockchain Development Guide: Understand the principles and implementation of blockchain in one article
BlockchainBasic Principle
Blockchain is a distributeddatabase that stores data on multiple nodes rather than on one central server. This makes the blockchain highly secure as attackers cannot steal data by attacking a node.
Data in the blockchain is stored in the form of blocks. Each block contains a hash, the hash of the previous block, a timestamp, and transaction data. A hash is a unique identifier that can be used to verify the integrity of a block.
Blockchain is an ever-growing chain, with each new block added to the end of the chain. This makes the blockchain highly tamper-resistant because once a block is added to the chain, it cannot be modified.
Use python to implement blockchain
Using PythonImplementing blockchain is relatively simple. We can use Python’s built-in module hashlib
to calculate the hash value, the datetime
module to get the timestamp, and the <strong class="keylink">JSON</strong>
module to store transaction data.
The following is a simple Python blockchain implementation:
import hashlib import datetime import json class Block: def __init__(self, index, timestamp, data, previous_hash): self.index = index self.timestamp = timestamp self.data = data self.previous_hash = previous_hash self.hash = self.calculate_hash() def calculate_hash(self): block_string = json.dumps(self.__dict__, sort_keys=True) return hashlib.sha256(block_string.encode()).hexdigest() def __repr__(self): return f"Block {self.index} ({self.hash})" class Blockchain: def __init__(self): self.chain = [] self.create_genesis_block() def create_genesis_block(self): genesis_block = Block(0, datetime.datetime.now(), [], "0") self.chain.append(genesis_block) def add_block(self, data): previous_block = self.chain[-1] new_block = Block(previous_block.index + 1, datetime.datetime.now(), data, previous_block.hash) self.chain.append(new_block) def is_valid(self): for i in range(1, len(self.chain)): current_block = self.chain[i] previous_block = self.chain[i - 1] if current_block.hash != current_block.calculate_hash(): return False if current_block.previous_hash != previous_block.hash: return False return True if __name__ == "__main__": blockchain = Blockchain() blockchain.add_block("Hello, world!") blockchain.add_block("This is a test.") print(blockchain.chain)
This simple blockchain implementation only contains the most basic functions. In practical applications, blockchain also needs to implement more functions, such as smart contracts, consensus mechanisms, etc.
Conclusion
Blockchain technology is developing rapidly and it is expected to have a significant impact on various industries in the next few years. With its powerful programming capabilities and rich library support, Python has become an ideal choice for blockchain development. This article introduces the basic principles of blockchain and demonstrates how to implement blockchain using Python. I hope this article can help you get started with blockchain development.
The above is the detailed content of Python Blockchain Development Guide: Understand the principles and implementation of blockchain in one article. For more information, please follow other related articles on the PHP Chinese website!
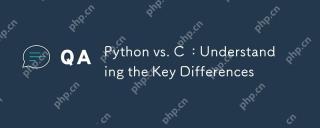
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
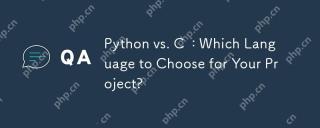
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
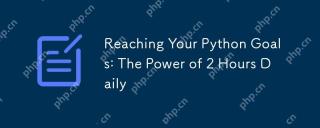
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
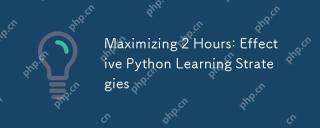
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
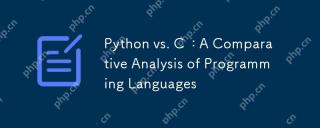
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
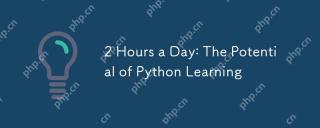
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
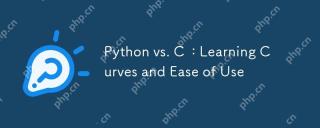
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
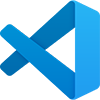
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
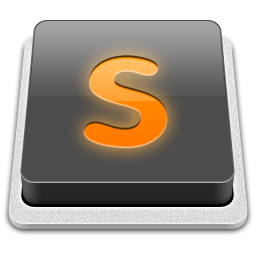
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
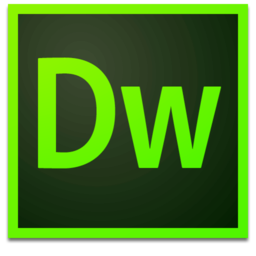
Dreamweaver Mac version
Visual web development tools