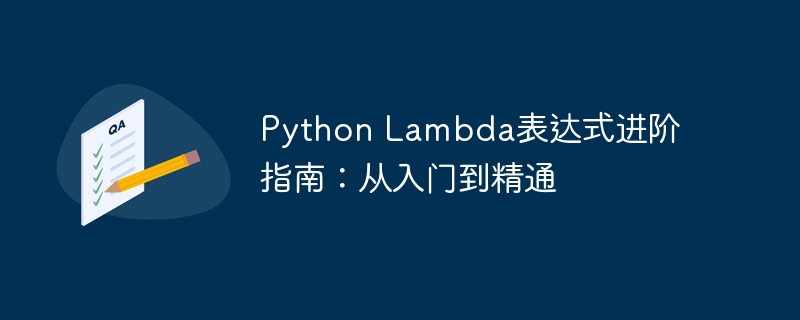
- Introduction and basic syntax of Lambda expression
Lambda expression consists of a function parameter list, a colon and a function body. The function parameter list is the same as that of an ordinary function, and the function body is an expression rather than a set of statements.
# 示例:返回一个函数,该函数接收两个数字并返回它们的和
sum = lambda x, y: x + y
- Application scenarios of Lambda expressions
Lambda expressions are great for use as callback functions, filter functions, and mapping functions.
- Callback function: A callback function refers to a function called in another function. Lambda expressions make it easy to create callback functions without declaring their names.
- Filter function: The filter function is used to filter out elements that meet certain conditions from a sequence. Lambda expressions make it easy to create filter functions to simplify your code.
- Mapping function: The mapping function is used to apply the same operation to each element in the sequence. Lambda expressions make it easy to create mapping functions to simplify your code.
- Advanced techniques for Lambda expressions
- Create closures using Lambda expressions: A closure is a function that can access variables outside its defining scope. Lambda expressions make it easy to create closures to simplify your code.
- Use Lambda expressions to implement higher-order functions: A higher-order function refers to a function that can receive a function as a parameter and return a function. Lambda expressions make it easy to implement higher-order functions to simplify your code.
- Use Lambda expressions to implement anonymous function classes: Anonymous function classes refer to classes without names. Lambda expressions make it easy to implement anonymous function classes to simplify your code.
- Notes on Lambda expressions
- The function body of a Lambda expression can only contain one expression and cannot contain statements. If you need to execute multiple statements, you need to define a normal function using the
def
keyword.
- Lambda expression parameters must be explicitly typed. This means you must specify the parameter type before the parameter type.
- Lambda expressions cannot contain
return
statements. The return value of the function body is the value of the function body expression.
- Summarize
Lambda expressions are a concise and powerful syntax construct in python that make it easy to create anonymous functions without declaring their names. Lambda expressions are great for use as callback functions, filter functions, and map functions, and can also be used to create closures, higher-order functions, and anonymous function classes. Mastering the concepts, syntax, application scenarios and some advanced techniques of Lambda expressions can help you write more concise, efficient and readable Python code.
The above is the detailed content of Advanced Guide to Python Lambda Expressions: From Beginner to Mastery. For more information, please follow other related articles on the PHP Chinese website!