In-depth understanding of the error handling mechanism in Golang
Golang is an efficient and highly concurrency programming language, and its error handling mechanism is very important when writing programs. In Golang, errors are treated as normal return values rather than handled through exceptions like in other languages. This article will delve into the error handling mechanism in Golang and provide specific code examples to illustrate.
Error type
In Golang, errors are represented through the built-in error
interface, which is defined as follows:
type error interface { Error() string }
Any implementation error
Objects of the interface can be treated as an error. Normally, when an error is encountered during function execution, an object that implements the error
interface will be returned.
Error handling example
The following is a sample program that shows how to handle errors in Golang:
package main import ( "errors" "fmt" ) func divide(a, b float64) (float64, error) { if b == 0 { return 0, errors.New("division by zero") } return a / b, nil } func main() { result, err := divide(10, 0) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Result:", result) } }
In the above example, divide
Function is used to calculate the division of two floating point numbers and returns an error object if the divisor is 0. In the main
function, call the divide
function and check the returned error. If there is an error, print the error message, otherwise print the calculation result.
Best practices for error handling
In actual programming, in order to improve the readability and maintainability of the code, the following best practices can be used to handle errors:
- Return an error object instead of
nil
: When a function may error, it should return an error object instead of returningnil
. This provides a clearer representation of program status. - Error passing instead of handling: When calling a function, errors should be passed to the caller for handling rather than handled inside the function. This makes error propagation clearer.
- Use package-level
Errors
variables: For some common errors, you can define them as package-level variables and share them in multiple places.
Chained calls for error handling
In Golang, you can handle multiple errors by chaining functions, such as using errors.Wrap
anderrors.Wrapf
Function:
package main import ( "errors" "fmt" "github.com/pkg/errors" ) func main() { err := errors.New("error occurred") wrappedErr := errors.Wrap(err, "additional information") fmt.Println(wrappedErr) formattedErr := errors.Wrapf(wrappedErr, "more details: %v", 42) fmt.Println(formattedErr) }
In the above code example, use errors.New
to create an error object, and then pass errors.Wrap
and errors.Wrapf
The function wraps the error and adds additional information and formatting information.
Summary
Through the above examples and explanations, we have a deep understanding of the error handling mechanism in Golang. By returning error
interface objects, error transmission and chained function calls, errors that may occur in the program can be better handled and the stability and reliability of the program can be improved. Writing good error handling code will help improve the readability and maintainability of the program, and promote the development and optimization of Golang programming.
The above is the detailed content of In-depth exploration of the error handling mechanism in Golang. For more information, please follow other related articles on the PHP Chinese website!
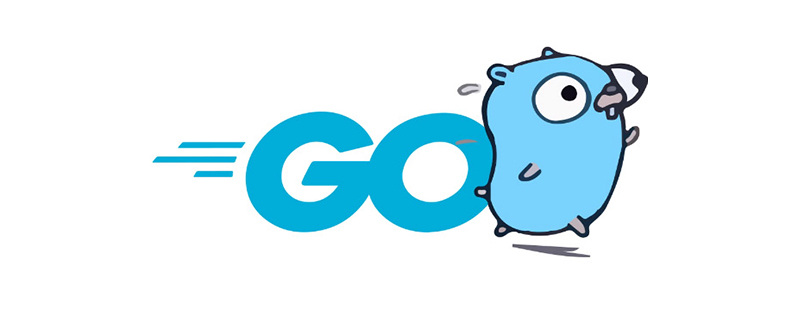
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
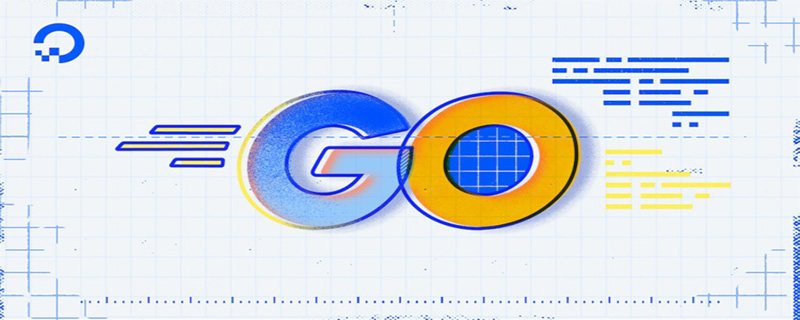
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
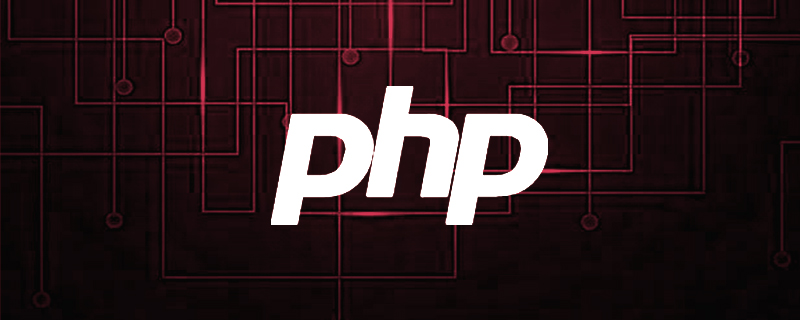
发现 Go 不仅允许我们创建更大的应用程序,并且能够将性能提高多达 40 倍。 有了它,我们能够扩展使用 PHP 编写的现有产品,并通过结合两种语言的优势来改进它们。
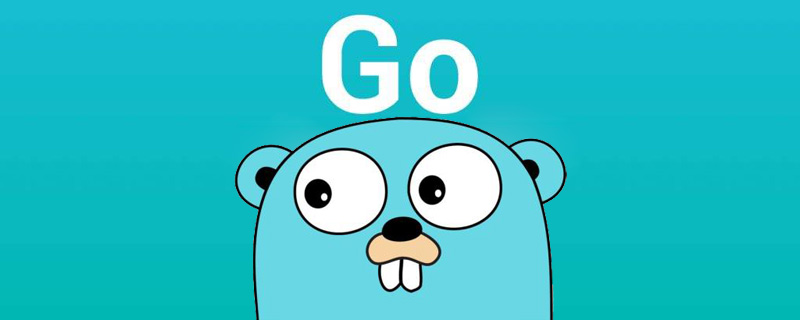
golang是一种静态强类型、编译型、并发型,并具有垃圾回收功能的编程语言;它可以在不损失应用程序性能的情况下极大的降低代码的复杂性,还可以发挥多核处理器同步多工的优点,并可解决面向对象程序设计的麻烦,并帮助程序设计师处理琐碎但重要的内存管理问题。
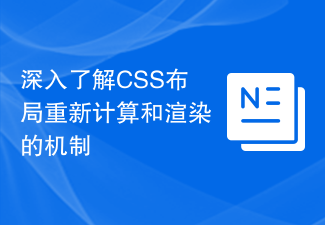
CSS回流(reflow)和重绘(repaint)是网页性能优化中非常重要的概念。在开发网页时,了解这两个概念的工作原理,可以帮助我们提高网页的响应速度和用户体验。本文将深入探讨CSS回流和重绘的机制,并提供具体的代码示例。一、CSS回流(reflow)是什么?当DOM结构中的元素发生可视性、尺寸或位置改变时,浏览器需要重新计算并应用CSS样式,然后重新布局
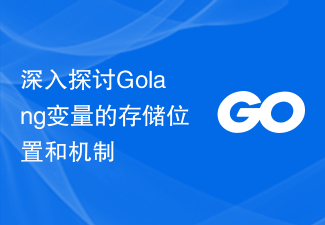
标题:深入探讨Golang变量的存储位置和机制随着Go语言(Golang)在云计算、大数据和人工智能领域的应用逐渐增多,深入了解Golang变量的存储位置和机制变得尤为重要。在本文中,我们将详细探讨Golang中变量的内存分配、存储位置以及相关的机制。通过具体代码示例,帮助读者更好地理解Golang变量在内存中是如何存储和管理的。1.Golang变量的内存


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
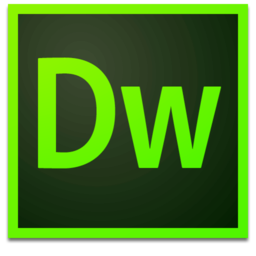
Dreamweaver Mac version
Visual web development tools
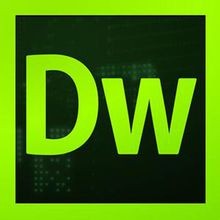
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
