Detailed explanation of PHP error handling
Error report
The general attribution of errors in PHP programs In the following three areas:
1. Grammar errors
Grammar errors are the most common and easy to fix. For example: a semicolon is missing in the code. This type of error will prevent the execution of the script
2. Runtime error
This kind of error will generally not prevent the execution of the PHP script, but will prevent what is currently being done. Output an error, but the php script continues to execute
3. Logic error
This kind of error is the most troublesome. It neither prevents the script from executing nor outputs an error message
[Note] If display_errors in the php.ini configuration file is set from the default on to off, no errors will be displayed
In the PHP script, ini_set( can be called ) function, dynamically set the php.ini configuration file
ini_set("display_errors","On"); //Display all error messages
Error level
Actually, the 13 error types in the table can Divided into 3 categories: attention level, warning level and error level. Generally, during the development process, attention-level errors are ignored
<?php getType($a);//未定义变量,注意级别 echo "1111111111111111<br>"; getType();//未传入参数,警告级别 echo "222222222222222222222<br>"; getType3();//函数名错误,错误级别 echo "333333333333333333333<br>"; ?>
Error handling
1. The first error handling method is to modify Configuration file
The default error level is to prompt all levels of errors: error_reporting = E_ALL
Change error_reporting = E_ALL
to error_reporting = E_ALL & ~E_NOTICE
means not to prompt attention level errors. Then, restart the service to take effect
error_reporting = E_ALL & ~E_NOTICE
Throw any unnoticed errors, the default value error_reporting = E_ERROR | E_PARSE | E_CORE_ERROR
Only consider fatal ones Runtime errors, new parsing errors and core errorserror_reporting = E_ALL & ~(E_USER_ERROR | E_USER_WARNING | E_USER_NOTICE)
Report all errors except user-caused errors
2. The second error handling method is to use the error handling function
In the PHP script, the error reporting level can be dynamically set through the error_reporting() function
<?php error_reporting(E_ALL & ~E_NOTICE); getType($a);//注意级别 echo "1111111111111111<br>"; getType();//警告级别 echo "222222222222222222222<br>"; getType3();//错误级别 echo "333333333333333333333<br>";?>
Customized Error Handling
Customize the way error reports are processed, which can completely bypass the standard PHP error handling function, so that you can print it in a format you define. Error report, or change the location where the error report is printed. You can consider customizing error handling in the following situations: 1. Write down error information and promptly discover some problems in the production environment; 2. Shield errors; 3. Control the output of errors. ; 4. As a debugging tool
Use the set_error_handler() function to set user-defined error handling
<?php //error_reporting(E_ALL & ~E_NOTICE); //在php中注册一个函数来处理错误报告,替代默认的方式 set_error_handler("myerrorfun"); $mess = ""; //自定义错误报告处理函数 function myerrorfun($error_type, $error_message, $error_file, $error_line) { global $mess; $mess.="发生错误级别为{$error_type}类型, 错误消息<b>{$error_message}</b>, 在文件<font >{$error_file}</font>中, 第{$error_line}行。<br>"; } getType($a); echo "1111111111111111<br>"; getType(); echo "222222222222222222222<br>"; echo "--------------------------------------------<br>"; echo $mess; ?>
Error log
Generally, programs will save error logs to record error information when the program is running. And the error log has its default storage location. We can modify the location of error information and error logs
In the PHP.ini configuration file, there are the following items that can be set for the error log
error_reporting = E_ALL
//Every error will be sent to PHP display_errors=Off
//Do not display error reportlog_errors=On //Determine the location of the log statement record
log_errors_max_log=1024
//The maximum length of each log itemerror_log=G:/myerror.log
//Specify the file where errors are written
In the php file, we can Use the function error_log() to customize error messages
<?phperror_log("登录失败了!");?>
Exception handling
Exception (Exception) handling is used to change the normal behavior of the script when a specified error occurs. Process is a new important feature in PHP5. Exception handling is an scalable and easy-to-maintain error handling mechanism, and provides a new object-oriented error handling method
try{
使用try去包含可能会发生异常的代码
一旦出现异常try进行捕获异常,交给catch处理。
抛出异常语句:throw 异常对象。
}catch(异常对象参数){
在这里做异常处理。
}[catch(。,,){
.. .. ..
}]
<?php try { $error = 'Always throw this error'; throw new Exception($error); //创建一个异常对象,通过throw语句抛出 echo 'Never executed'; //从这里开始,try代码块内的代码将不会再被执行 } catch (Exception $e) { echo ‘Caught exception: ’.$e->getMessage()." "; //输出捕获的异常消息 } echo 'Hello World'; //程序没有崩溃继续向下执行?>
自定义异常
用户可以用自定义的异常处理类来扩展PHP内置的异常处理类。以下的代码说明了在内置的异常处理类中,哪些属性和方法在子类中是可访问和可继承的
<?phpclass Exception{ protected $message = 'Unknown exception'; // 异常信息 private $string; // __toString cache protected $code = 0; // 用户自定义异常代码 protected $file; // 发生异常的文件名 protected $line; // 发生异常的代码行号 private $trace; // backtrace private $previous; // previous exception if nested exception public function __construct($message = null, $code = 0, Exception $previous = null); final private function __clone(); // Inhibits cloning of exceptions. final public function getMessage(); // 返回异常信息 final public function getCode(); // 返回异常代码 final public function getFile(); // 返回发生异常的文件名 final public function getLine(); // 返回发生异常的代码行号 final public function getTrace(); // backtrace() 数组 final public function getPrevious(); // 之前的 exception final public function getTraceAsString(); // 已格成化成字符串的 getTrace() 信息 // Overrideable public function __toString(); // 可输出的字符串}?>
[注意]如果使用自定义的类来扩展内置异常处理类,并且要重新定义构造函数的话,建议同时调用parent::__construct()来检查所有的变量是否已被赋值。当对象要输出字符串的时候,可以重载__toString() 并自定义输出的样式
<?php /* 自定义的一个异常处理类,但必须是扩展内异常处理类的子类 */ class MyException extends Exception{ //重定义构造器使第一个参数 message 变为必须被指定的属性 public function __construct($message, $code=0){ //可以在这里定义一些自己的代码 //建议同时调用 parent::construct()来检查所有的变量是否已被赋值 parent::__construct($message, $code); } public function __toString() { //重写父类方法,自定义字符串输出的样式 return __CLASS__.":[".$this->code."]:".$this->message."<br>"; } public function customFunction() { //为这个异常自定义一个处理方法 echo "按自定义的方法处理出现的这个类型的异常<br>"; } }?>
<?php try { //使用自定义的异常类捕获一个异常,并处理异常 $error = '允许抛出这个错误'; throw new MyException($error); //创建一个自定义的异常类对象,通过throw语句抛出 echo 'Never executed'; //从这里开始,try代码块内的代码将不会再被执行 } catch (MyException $e) { //捕获自定义的异常对象 echo '捕获异常: '.$e; //输出捕获的异常消息 $e->customFunction(); //通过自定义的异常对象中的方法处理异常 } echo '你好呀'; //程序没有崩溃继续向下执行?>
相关参考:php教程
The above is the detailed content of Detailed explanation of error handling in PHP. For more information, please follow other related articles on the PHP Chinese website!
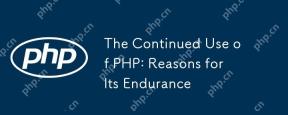
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
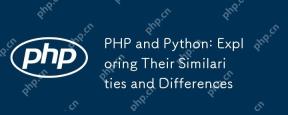
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
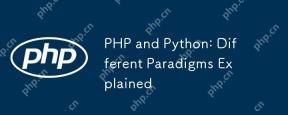
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
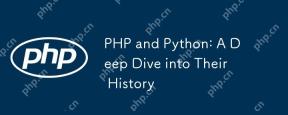
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
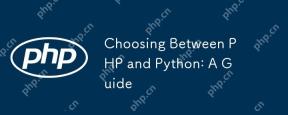
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
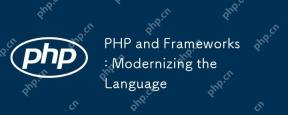
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
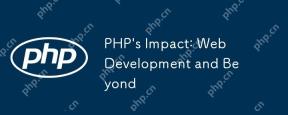
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
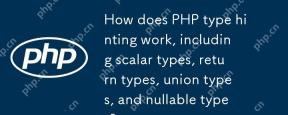
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
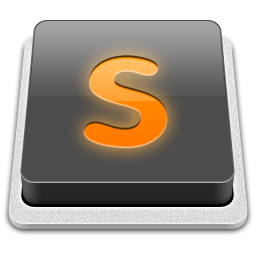
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor