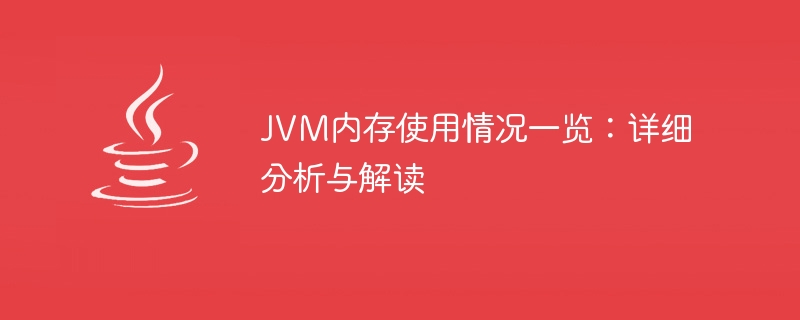
JVM memory usage overview: detailed analysis and interpretation
Abstract: JVM memory is an important part of Java application runtime, correctly analyze and interpret JVM memory Usage is critical to optimizing application performance. This article will delve into all aspects of JVM memory, including memory models, memory partitions, heap memory, stack memory, method areas, garbage collection, etc., and explain the usage of JVM memory through specific code examples.
- JVM memory model
The JVM memory model consists of three parts: heap, stack and method area. The heap is the main memory area managed by the Java virtual machine and is used to store object instances and arrays. The stack is used to store method calls, local variables, etc. The method area is used to store class information, constant pool, static variables, etc.
- JVM memory partition
The JVM memory is divided into three main areas: Young area, Old area and Permanent area. The Young area is mainly used to store newly created objects, the Old area is used to store objects with long survival times, and the Permanent area is used to store static variables, constants and other objects that are not easy to recycle.
- Heap memory
Heap memory is the largest memory area in the JVM, used to store created object instances. The heap memory is divided into the new generation and the old generation. The new generation is divided into the Eden area and two Survivor areas. Objects are first created in the Eden area. When the Eden area is full, Minor GC (new generation garbage collection) is triggered and the surviving objects are copied to the Survivor area. When the Survivor area is full, surviving objects will be copied to the old generation, and non-surviving objects will be recycled.
- Stack memory
Stack memory is used to store method calls and local variables. Each thread has its own stack frame, and one stack frame corresponds to one method call. The stack frame contains local variable table, operand stack, dynamic link, return address and additional information, etc. The local variable table is used to store local variables in methods.
- Method area
The method area stores class information, constant pool, static variables, etc. Full GC will be triggered when there is insufficient memory in the method area. After JDK8, the method area was removed and replaced by Metaspace, which uses local memory to store class information.
- Garbage Collection
The JVM uses the garbage collection mechanism to automatically recycle unused memory to prevent memory leaks. There are many garbage collection algorithms, including mark-sweep, copy, mark-compact, etc. Garbage collectors include Serial GC, Parallel GC, CMS GC, G1 GC, etc. Each collector is suitable for different scenarios.
The following is a sample code illustrating JVM memory usage:
public class MemoryUsageExample {
public static void main(String[] args) {
// 声明一个数组,占用一定的内存
int[] array = new int[1000000];
// 打印JVM的总内存和可用内存
System.out.println("Total Memory: " + Runtime.getRuntime().totalMemory());
System.out.println("Free Memory: " + Runtime.getRuntime().freeMemory());
// 强制进行垃圾回收
System.gc();
// 打印JVM的总内存和可用内存
System.out.println("Total Memory: " + Runtime.getRuntime().totalMemory());
System.out.println("Free Memory: " + Runtime.getRuntime().freeMemory());
}
}
In the above code, we create an array containing 1 million integers, which will occupy a certain amount of heap Memory. Then, we print the total memory and available memory of the JVM through the totalMemory()
method and the freeMemory()
method of the Runtime
class respectively. Finally, we force a garbage collection and again print the total and free memory of the JVM. By comparing the results of the two prints, we can observe the impact of garbage collection on memory.
Conclusion: Correctly analyzing and interpreting JVM memory usage is critical to optimizing application performance. By understanding the JVM memory model, memory partitions, heap memory, stack memory, method area, and garbage collection, developers can better tune the performance and memory usage of Java applications.
References:
- "Understanding JVM Architecture", Oracle Docs
- "The Memory Management, Java SE 11 Edition", OpenJDK
(Word count: 800)
The above is the detailed content of JVM memory usage overview: detailed analysis and interpretation. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn