


An in-depth analysis of Java multithreading: exploring different implementation methods
In-depth analysis of Java multithreading: exploring different implementation methods, requiring specific code examples
Abstract:
As a widely used programming language, Java provides Rich multi-threading support. This article will delve into the implementation of Java multi-threading, including inheriting the Thread class, implementing the Runnable interface, and using the thread pool. Through specific code examples, readers will be able to better understand and apply these methods.
- Introduction
Multi-threaded programming is an important technology that can make full use of multi-core processors and improve program performance. In Java, multi-threading can be implemented by inheriting the Thread class, implementing the Runnable interface, and using a thread pool. Different implementations are suitable for different scenarios, and they will be introduced and compared one by one next. - Inherit the Thread class
Inheriting the Thread class is a simple way to implement multi-threading. Define the execution logic of the thread by creating a subclass of the Thread class and overriding the run() method in the subclass. The following is a sample code that uses the inherited Thread class to implement multi-threading:
public class MyThread extends Thread { @Override public void run() { for (int i = 0; i < 10; i++) { System.out.println("Thread 1: " + i); } } } public class Main { public static void main(String[] args) { MyThread thread1 = new MyThread(); thread1.start(); for (int i = 0; i < 10; i++) { System.out.println("Main thread: " + i); } } }
- Implementing the Runnable interface
The way of inheriting the Thread class has certain limitations, because Java is single inheritance . In order to overcome this limitation, we can implement the Runnable interface and override the run() method in the implementation class to define the execution logic of the thread. The following is a sample code that uses the Runnable interface to implement multi-threading:
public class MyRunnable implements Runnable { @Override public void run() { for (int i = 0; i < 10; i++) { System.out.println("Thread 2: " + i); } } } public class Main { public static void main(String[] args) { MyRunnable myRunnable = new MyRunnable(); Thread thread2 = new Thread(myRunnable); thread2.start(); for (int i = 0; i < 10; i++) { System.out.println("Main thread: " + i); } } }
- Using a thread pool
Using a thread pool can better manage and reuse threads and avoid frequent creation and destroy the thread. Java provides the ExecutorService interface and its implementation class ThreadPoolExecutor to support the use of thread pools. The following is a sample code that uses a thread pool to implement multi-threading:
public class MyTask implements Runnable { private int taskId; public MyTask(int taskId) { this.taskId = taskId; } @Override public void run() { System.out.println("Task " + taskId + " is running."); } } public class Main { public static void main(String[] args) { ExecutorService executorService = Executors.newFixedThreadPool(5); for (int i = 0; i < 10; i++) { MyTask task = new MyTask(i); executorService.execute(task); } executorService.shutdown(); } }
- Summary
By inheriting the Thread class, implementing the Runnable interface and using the thread pool, we can effectively implement Java multi-threading thread. In actual development, we need to choose an appropriate implementation method according to specific needs. Inheriting the Thread class is suitable for simple thread tasks, implementing the Runnable interface is suitable for scenarios that require multiple inheritance, and using the thread pool can better manage threads. Through the introduction and sample code of this article, readers should have a deeper understanding and mastery of Java multithreading.
Reference:
- Oracle. (n.d.). The Java™ Tutorials - Lesson: Concurrency. Oracle. Retrieved from https://docs.oracle.com/ javase/tutorial/essential/concurrency/
The above is the detailed content of An in-depth analysis of Java multithreading: exploring different implementation methods. For more information, please follow other related articles on the PHP Chinese website!
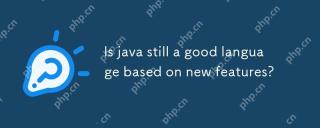
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
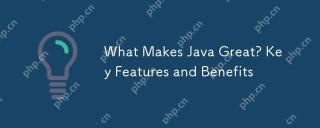
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
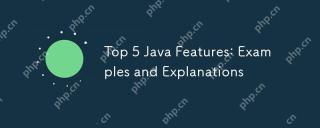
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.
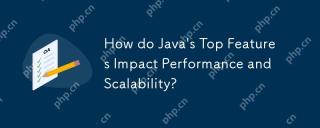
Java'stopfeaturessignificantlyenhanceitsperformanceandscalability.1)Object-orientedprincipleslikepolymorphismenableflexibleandscalablecode.2)Garbagecollectionautomatesmemorymanagementbutcancauselatencyissues.3)TheJITcompilerboostsexecutionspeedafteri
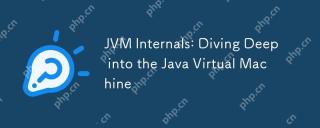
The core components of the JVM include ClassLoader, RuntimeDataArea and ExecutionEngine. 1) ClassLoader is responsible for loading, linking and initializing classes and interfaces. 2) RuntimeDataArea contains MethodArea, Heap, Stack, PCRegister and NativeMethodStacks. 3) ExecutionEngine is composed of Interpreter, JITCompiler and GarbageCollector, responsible for the execution and optimization of bytecode.
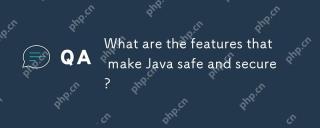
Java'ssafetyandsecurityarebolsteredby:1)strongtyping,whichpreventstype-relatederrors;2)automaticmemorymanagementviagarbagecollection,reducingmemory-relatedvulnerabilities;3)sandboxing,isolatingcodefromthesystem;and4)robustexceptionhandling,ensuringgr
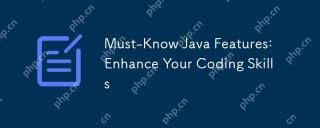
Javaoffersseveralkeyfeaturesthatenhancecodingskills:1)Object-orientedprogrammingallowsmodelingreal-worldentities,exemplifiedbypolymorphism.2)Exceptionhandlingprovidesrobusterrormanagement.3)Lambdaexpressionssimplifyoperations,improvingcodereadability
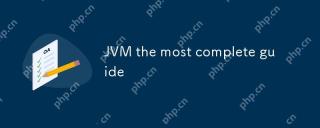
TheJVMisacrucialcomponentthatrunsJavacodebytranslatingitintomachine-specificinstructions,impactingperformance,security,andportability.1)TheClassLoaderloads,links,andinitializesclasses.2)TheExecutionEngineexecutesbytecodeintomachineinstructions.3)Memo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
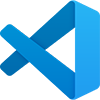
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
