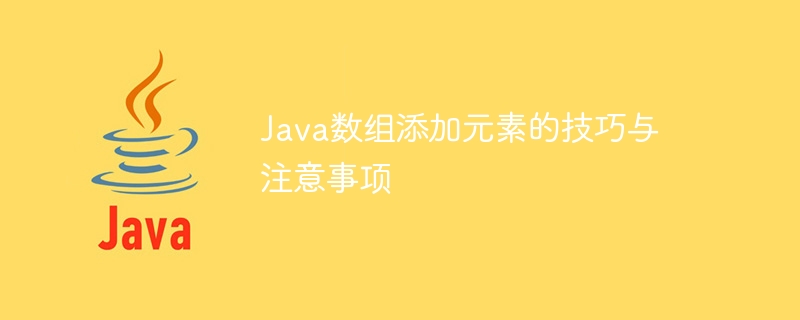
Tips and precautions for adding elements to Java arrays
In Java, arrays are a very common and important data structure. An array provides a container for storing multiple elements of the same type, and the elements in it can be accessed and modified through indexing. Sometimes, we need to add new elements to an existing array. This article will introduce some tips and precautions for adding elements to Java arrays, and illustrate them with specific code examples.
- Using the copyOf method
Java provides the copyOf method of the Arrays class, which can be used to create a new array and copy the elements of the original array to the new array. We can add an element to the end of the new array by setting the size of the new array to the length of the original array plus one. The following is a sample code that uses the copyOf method to add elements:
int[] originalArray = {1, 2, 3, 4, 5};
int[] newArray = Arrays.copyOf(originalArray, originalArray.length + 1);
newArray[newArray.length - 1] = 6;
In the above code, an original array originalArray is first created, and then the Arrays.copyOf method is used to copy it to a new array newArray. , and set the size of the new array to the length of the original array plus one. Finally, place the element 6 you want to add at the end of the new array.
- Using the ArrayList class
Another commonly used way to add elements is to use the ArrayList class. ArrayList is a dynamic array implementation in the Java collection framework that automatically adjusts its size as needed. ArrayList provides the add method to add elements to the end of the array. The following is a sample code that uses the ArrayList class to add elements:
ArrayList<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
list.add(3);
list.add(4);
list.add(5);
In the above code, an ArrayList object list is first created, and the add method is used to add elements to the end of the array.
- Notes
When adding elements using the above method, there are some things to note:
- The size of the array is fixed, once created It can't be changed. Therefore, when adding elements, you need to create a new array or use a dynamic array implementation like ArrayList.
- When using the copyOf method to add elements, you need to pay attention to copying the elements of the original array to the new array, and adding new elements to the new array.
- When using the ArrayList class to add elements, you do not need to specify the size of the array in advance. It will automatically adjust the size of the array according to actual needs.
To sum up, this article introduces some tips and precautions for adding elements to Java arrays, and illustrates them through specific code examples. I hope readers can master these techniques and use them flexibly in actual development.
The above is the detailed content of Tips and precautions for adding elements to Java arrays. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn